帮我用C++语言实现一个的图书管理系统,设计基类和派生类,并运用多态性和虚函数的知识。在控制台进行操作,需要有详细的代码注释,系统要有这些功能: 1)建立书籍类、图书类型类、图书类 2)书籍、图书类型、图书信息的初始化 3)书籍、图书类型、图书信息的添加、修改、删除、查找 4)图书信息的输出 5)对图书信息按编号、书名排序 6)将图书信息保存为文件 7)读入保存的图书信息
时间: 2023-11-24 22:07:03 浏览: 106
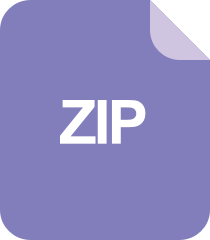
C++实验 多态性与虚函数

好的,我可以帮你解答这个问题。下面是一个简单的示例代码,我们可以根据这个代码进行修改和扩展,实现一个基本的图书管理系统。
首先,我们需要设计基类和派生类。基类是Book类,派生类有Fiction类和NonFiction类。Book类包含图书编号、书名、作者、价格等基本信息,Fiction类和NonFiction类分别表示小说类和非小说类的图书,它们有自己的特定属性。
```cpp
#include <iostream>
#include <string>
#include <vector>
#include <algorithm>
#include <fstream>
using namespace std;
class Book {
protected:
string book_id; // 图书编号
string book_name; // 书名
string author; // 作者
double price; // 价格
public:
Book() {}
Book(string id, string name, string auth, double p) :
book_id(id), book_name(name), author(auth), price(p) {}
virtual ~Book() {}
virtual void display() = 0; // 纯虚函数,输出图书信息
virtual string get_type() = 0; // 纯虚函数,获取图书类型
string get_id() { return book_id; } // 获取图书编号
string get_name() { return book_name; } // 获取书名
};
class Fiction : public Book {
private:
string genre; // 小说类别
public:
Fiction() {}
Fiction(string id, string name, string auth, double p, string gen) :
Book(id, name, auth, p), genre(gen) {}
virtual ~Fiction() {}
virtual void display(); // 虚函数,输出小说信息
virtual string get_type() { return "小说"; } // 实现基类中的纯虚函数
};
class NonFiction : public Book {
private:
string subject; // 非小说类别
public:
NonFiction() {}
NonFiction(string id, string name, string auth, double p, string sub) :
Book(id, name, auth, p), subject(sub) {}
virtual ~NonFiction() {}
virtual void display(); // 虚函数,输出非小说信息
virtual string get_type() { return "非小说"; } // 实现基类中的纯虚函数
};
```
接下来,我们需要实现图书管理系统的各个功能。首先是初始化图书信息,这里我们使用向量来存储所有图书。
```cpp
// 初始化图书信息
vector<Book*> books;
void init_books() {
books.push_back(new Fiction("001", "三体", "刘慈欣", 45.0, "科幻"));
books.push_back(new Fiction("002", "流浪地球", "刘慈欣", 40.0, "科幻"));
books.push_back(new NonFiction("003", "计算机网络", "谢希仁", 65.0, "计算机科学"));
books.push_back(new NonFiction("004", "操作系统", "汤小丹", 55.0, "计算机科学"));
books.push_back(new Fiction("005", "活着", "余华", 28.0, "文学"));
}
```
添加图书信息需要根据图书类型来创建不同的派生类对象,并将对象指针添加到向量中。
```cpp
// 添加图书信息
void add_book() {
string id, name, author, type;
double price;
cout << "请输入图书编号:";
cin >> id;
cout << "请输入书名:";
cin >> name;
cout << "请输入作者:";
cin >> author;
cout << "请输入价格:";
cin >> price;
cout << "请输入图书类型(小说/非小说):";
cin >> type;
if (type == "小说") {
string genre;
cout << "请输入小说类别:";
cin >> genre;
books.push_back(new Fiction(id, name, author, price, genre));
cout << "添加成功!" << endl;
}
else if (type == "非小说") {
string subject;
cout << "请输入非小说类别:";
cin >> subject;
books.push_back(new NonFiction(id, name, author, price, subject));
cout << "添加成功!" << endl;
}
else {
cout << "输入错误!" << endl;
}
}
```
修改图书信息需要根据图书编号查找到对应的图书,并进行修改。
```cpp
// 修改图书信息
void modify_book() {
string id;
cout << "请输入要修改的图书编号:";
cin >> id;
bool found = false;
for (auto it = books.begin(); it != books.end(); it++) {
if ((*it)->get_id() == id) {
string name, author;
double price;
cout << "请输入新的书名:";
cin >> name;
cout << "请输入新的作者:";
cin >> author;
cout << "请输入新的价格:";
cin >> price;
(*it)->Book::book_name = name;
(*it)->Book::author = author;
(*it)->Book::price = price;
found = true;
cout << "修改成功!" << endl;
break;
}
}
if (!found) {
cout << "未找到该图书!" << endl;
}
}
```
删除图书信息根据图书编号进行查找,并将图书对象从向量中删除。
```cpp
// 删除图书信息
void delete_book() {
string id;
cout << "请输入要删除的图书编号:";
cin >> id;
bool found = false;
for (auto it = books.begin(); it != books.end(); it++) {
if ((*it)->get_id() == id) {
books.erase(it);
found = true;
cout << "删除成功!" << endl;
break;
}
}
if (!found) {
cout << "未找到该图书!" << endl;
}
}
```
查找图书信息可以根据图书编号或书名进行查找。
```cpp
// 按图书编号查找图书信息
void find_by_id() {
string id;
cout << "请输入要查找的图书编号:";
cin >> id;
bool found = false;
for (auto it = books.begin(); it != books.end(); it++) {
if ((*it)->get_id() == id) {
(*it)->display();
found = true;
break;
}
}
if (!found) {
cout << "未找到该图书!" << endl;
}
}
// 按书名查找图书信息
void find_by_name() {
string name;
cout << "请输入要查找的书名:";
cin >> name;
bool found = false;
for (auto it = books.begin(); it != books.end(); it++) {
if ((*it)->get_name() == name) {
(*it)->display();
found = true;
}
}
if (!found) {
cout << "未找到该图书!" << endl;
}
}
```
输出所有图书信息可以遍历向量,并调用每个图书对象的display()函数进行输出。
```cpp
// 输出所有图书信息
void display_all_books() {
cout << "图书编号\t书名\t作者\t价格\t类型\t类别" << endl;
for (auto it = books.begin(); it != books.end(); it++) {
(*it)->display();
}
}
```
按编号或书名排序可以使用STL中的sort()函数进行排序。
```cpp
// 按编号排序
bool sort_by_id(Book* b1, Book* b2) {
return b1->get_id() < b2->get_id();
}
// 按书名排序
bool sort_by_name(Book* b1, Book* b2) {
return b1->get_name() < b2->get_name();
}
// 对图书信息按编号排序
void sort_by_id() {
sort(books.begin(), books.end(), sort_by_id);
cout << "按编号排序完成!" << endl;
}
// 对图书信息按书名排序
void sort_by_name() {
sort(books.begin(), books.end(), sort_by_name);
cout << "按书名排序完成!" << endl;
}
```
将图书信息保存为文件需要使用文件流,将每本图书的信息写入文件中。
```cpp
// 将图书信息保存为文件
void save_books_to_file() {
string filename;
cout << "请输入文件名:";
cin >> filename;
ofstream fout(filename);
if (fout.is_open()) {
for (auto it = books.begin(); it != books.end(); it++) {
fout << (*it)->get_id() << '\t'
<< (*it)->get_name() << '\t'
<< (*it)->Book::author << '\t'
<< (*it)->Book::price << '\t'
<< (*it)->get_type() << '\t';
if ((*it)->get_type() == "小说") {
Fiction* fic = dynamic_cast<Fiction*>(*it);
fout << fic->display() << endl;
}
else if ((*it)->get_type() == "非小说") {
NonFiction* non = dynamic_cast<NonFiction*>(*it);
fout << non->display() << endl;
}
}
fout.close();
cout << "保存成功!" << endl;
}
else {
cout << "文件打开失败!" << endl;
}
}
```
读入保存的图书信息需要使用文件流,逐行读取文件内容,并根据每行的内容创建相应的图书对象。
```cpp
// 读入保存的图书信息
void read_books_from_file() {
string filename;
cout << "请输入文件名:";
cin >> filename;
ifstream fin(filename);
if (fin.is_open()) {
books.clear();
string line;
while (getline(fin, line)) {
string id, name, author, type, genre, subject;
double price;
stringstream ss(line);
ss >> id >> name >> author >> price >> type;
if (type == "小说") {
ss >> genre;
books.push_back(new Fiction(id, name, author, price, genre));
}
else if (type == "非小说") {
ss >> subject;
books.push_back(new NonFiction(id, name, author, price, subject));
}
}
fin.close();
cout << "读取成功!" << endl;
}
else {
cout << "文件打开失败!" << endl;
}
}
```
最后,我们需要在main函数中调用各个功能函数,实现图书管理系统的完整功能。
```cpp
int main() {
init_books(); // 初始化图书信息
while (true) {
cout << endl;
cout << "-----------------------" << endl;
cout << "1. 添加图书信息" << endl;
cout << "2. 修改图书信息" << endl;
cout << "3. 删除图书信息" << endl;
cout << "4. 按编号查找图书信息" << endl;
cout << "5. 按书名查找图书信息" << endl;
cout << "6. 输出所有图书信息" << endl;
cout << "7. 按编号排序" << endl;
cout << "8. 按书名排序" << endl;
cout << "9. 将图书信息保存为文件" << endl;
cout << "10. 读入保存的图书信息" << endl;
cout << "0. 退出" << endl;
cout << "-----------------------" << endl;
cout << "请输入数字选择功能:";
int choice;
cin >> choice;
switch (choice) {
case 1:
add_book();
break;
case 2:
modify_book();
break;
case 3:
delete_book();
break;
case 4:
find_by_id();
break;
case 5:
find_by_name();
break;
case 6:
display_all_books();
break;
case 7:
sort_by_id();
break;
case 8:
sort_by_name();
break;
case 9:
save_books_to_file();
break;
case 10:
read_books_from_file();
break;
case 0:
return 0;
default:
cout << "输入错误,请重新输入!" << endl;
break;
}
}
return 0;
}
```
这就是一个基本的图书管理系统的实现。需要注意的是,这只是一个示例代码,实际使用时还需要考虑更多的细节和功能实现。
阅读全文
相关推荐
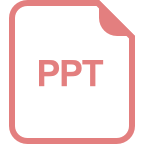
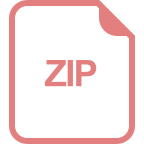














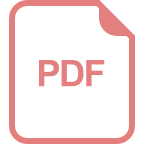
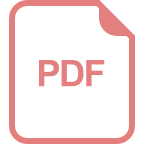