数制转化:编写程序,将十进制整数N转换为二进制数。要求:使用栈的基本运算(包括InitStack(S),Pop(S),Push(S),IsEmpty(S)
时间: 2024-11-16 11:20:25 浏览: 29
要编写一个C语言程序,使用栈数据结构将十进制整数转换成二进制数,我们可以按照以下步骤进行:
1. 初始化栈 `S` 和创建一个临时数组用于存储中间结果。
2. 定义一个辅助函数来获取当前数字的二进制位。
3. 将十进制数不断除以2并取余,直到商为0。每次得到的余数就是二进制数的一位。
4. 使用栈将这些余数存入栈,因为栈的后进先出特性保证了正确的顺序。
5. 当栈不为空时,取出栈顶元素并打印出来。
下面是对应的C语言代码:
```c
#include <stdio.h>
#include <stdlib.h>
// 声明栈的数据结构
typedef struct Stack {
int top;
int data[100];
} Stack;
// 初始化栈
void InitStack(Stack *s) {
s->top = -1;
}
// 判断栈是否为空
int IsEmpty(Stack *s) {
return s->top == -1;
}
// 入栈操作
void Push(Stack *s, int val) {
if (s->top < sizeof(s->data) - 1) {
s->top++;
s->data[s->top] = val;
} else {
printf("Stack overflow.\n");
}
}
// 出栈操作
int Pop(Stack *s) {
if (IsEmpty(s)) {
printf("Stack is empty.\n");
return -1;
}
int val = s->data[s->top];
s->top--;
return val;
}
// 十进制转二进制
void decimalToBinary(int N, Stack *s) {
while (N > 0) {
Push(s, N % 2);
N /= 2;
}
}
// 打印栈中的二进制数
void printBinary(Stack *s) {
while (!IsEmpty(s)) {
int bit = Pop(s);
printf("%d", bit);
}
printf("\n");
}
int main() {
Stack s;
InitStack(&s);
int N;
printf("Enter a decimal number: ");
scanf("%d", &N);
// 转换并打印二进制
decimalToBinary(N, &s);
printf("Binary representation: ");
printBinary(&s);
return 0;
}
```
在这个程序中,`decimalToBinary` 函数负责将十进制数转换成二进制并入栈,然后`printBinary` 函数从栈中读出并打印这些二进制位。
阅读全文
相关推荐
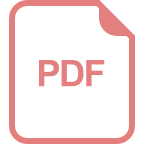
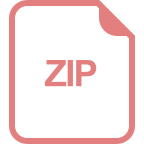
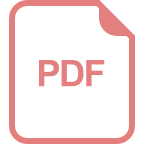
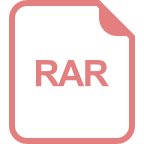
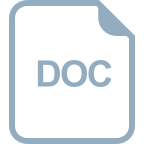
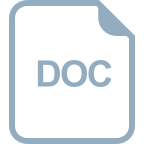
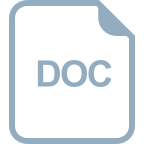
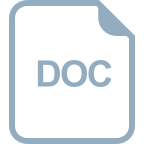
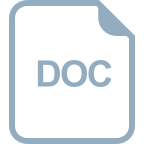
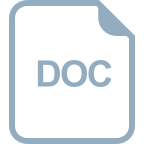
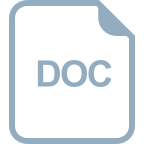
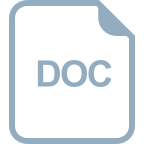
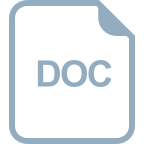
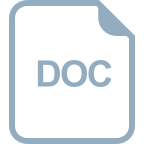
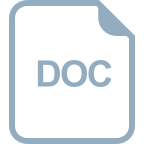
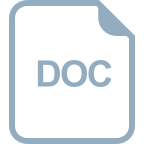
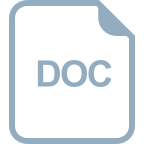
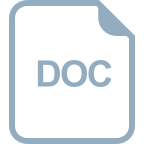
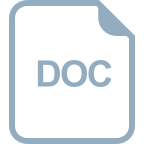