c++判断素数代码 判断最大公因子代码 求私钥代码 加密过程代码 解密过程代码
时间: 2024-09-25 17:14:09 浏览: 51
在C++中,这里分别介绍一些基本的代码片段,但请注意,加密、解密涉及到复杂的数学算法,尤其是涉及RSA(一种非对称加密算法)的私钥生成和数据加密解密。
1. **判断素数** (质数判定)
```cpp
bool isPrime(int num) {
if (num <= 1) return false;
if (num <= 3) return true;
if (num % 2 == 0 || num % 3 == 0) return false;
for (int i = 5; i * i <= num; i += 6) {
if (num % i == 0 || num % (i + 2) == 0) return false;
}
return true;
}
```
2. **求最大公约数** (Euclidean Algorithm)
```cpp
int gcd(int a, int b) {
while (b != 0) {
int temp = a % b;
a = b;
b = temp;
}
return a;
}
```
3. **生成RSA私钥** (简化版,实际操作需要安全库)
```cpp
#include <openssl/pem.h>
#include <openssl/rand.h>
// ...
BIGNUM* generateKeys() {
BIGNUM* p, *q, *n, *d, *e;
// ... 随机生成两个大素数p和q...
RSA* rsa = RSA_new();
RSA_generate_key_ex(rsa, key_size, &p, &q);
d = BN_mod_inverse(NULL, p, q); // 计算私钥d
e = RSA_get_e(rsa); // 公钥e直接获取
// ... 将密钥转换成PEM格式存储...
return d;
}
```
4. **RSA加密过程**
```cpp
string encrypt(const string& plaintext, const BIGNUM* pubKeyE, const BIGNUM* modulusN) {
string ciphertext;
unsigned char buffer[plaintext.size() * 2 + 1];
RSA_public_encrypt(plaintext.length(), reinterpret_cast<const unsigned char*>(plaintext.c_str()), buffer, pubKeyE, RSA_PKCS1_OAEP_PADDING);
ciphertext = string(reinterpret_cast<char*>(buffer), buffer长度);
return ciphertext;
}
```
5. **RSA解密过程**
```cpp
string decrypt(const string& ciphertext, const BIGNUM* privKeyD, const BIGNUM* modulusN) {
string decrypted;
unsigned char buffer[ciphertext.size()];
RSA_private_decrypt(ciphertext.length(), reinterpret_cast<const unsigned char*>(ciphertext.c_str()), buffer, privKeyD, RSA_PKCS1_OAEP_PADDING);
decrypted = string(reinterpret_cast<char*>(buffer));
return decrypted;
}
```
以上代码仅作教学用途,实际生产环境中会使用专业的加密库并采取更为严格的保密措施。
阅读全文
相关推荐
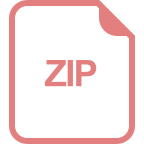
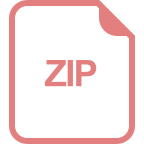
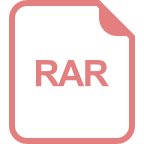
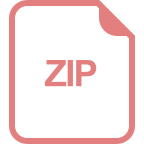
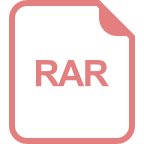
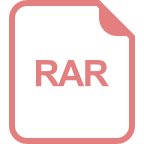
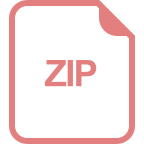
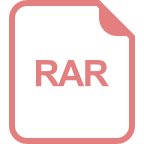
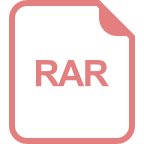
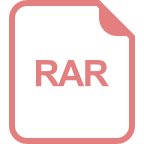
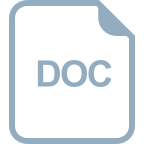
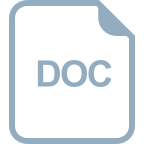
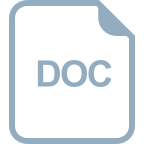





