char *b=NULL;SetUpTCPtoSendInformation("get",b);b可以取到"set"的值么
时间: 2023-12-28 21:03:49 浏览: 77
不,使用 `char *b = NULL;` 声明的字符指针 `b` 是一个空指针,无法直接传递给 `SetUpTCPtoSendInformation` 函数。此外,`SetUpTCPtoSendInformation` 函数中的参数 `char *command` 是一个指向字符数组的指针,而不是一个指向指针的指针。因此,无法直接将空指针 `b` 传递给 `SetUpTCPtoSendInformation` 函数来获取 "set" 的值。
如果你想使用 `b` 来获取 "set" 的值,你需要对函数进行修改。以下是一个示例修改的函数:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int SetUpTCPtoSendInformation(char option[], char **command) {
static int isFirstCall = 1;
pthread_mutex_lock(&mutex); // 加锁
if (isFirstCall && strstr(option, "set")) {
*command = malloc(strlen(TCPCommand) + 1);
if (*command == NULL) {
printf("Failed to set and obtain TCP command variable memory allocation\n");
goto fail;
}
strcpy(*command, TCPCommand);
printf("set: %s\n", *command);
isFirstCall = 0;
goto succeed;
} else if (!isFirstCall && strstr(option, "get") && TCPCommand != NULL && strlen(TCPCommand)) {
memset(*command, '\0', strlen(*command));
*command = realloc(*command, strlen(TCPCommand) + 1);
if (*command == NULL) {
printf("Failed to reallocate memory for command\n");
goto fail;
}
strcpy(*command, TCPCommand);
printf("get: %s\n", *command);
isFirstCall = 1;
goto succeed;
}
memset(*command, 0, sizeof(*command));
strcpy(*command, "");
fail:
pthread_mutex_unlock(&mutex); // 解锁
return 0;
succeed:
pthread_mutex_unlock(&mutex); // 解锁
return 1;
}
```
在这个修改后的函数中,我们将 `command` 参数改为了 `char **command`,这样我们可以通过间接引用来修改指针指向的值。当 `option` 是 "set" 时,我们使用 `*command` 来分配内存并复制 `TCPCommand` 的值。当 `option` 是 "get" 时,我们使用 `*command` 来重新分配内存并复制 `TCPCommand` 的值。
调用修改后的函数的示例代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
int SetUpTCPtoSendInformation(char option[], char **command);
int main() {
char *b = NULL;
if (SetUpTCPtoSendInformation("get", &b)) {
printf("Value of b: %s\n", b);
free(b);
}
return 0;
}
```
在这个示例程序中,我们首先声明了字符指针 `b` 并将其初始化为 `NULL`。然后,我们调用 `SetUpTCPtoSendInformation` 函数,并将 `&b` 传递给函数来获取 "set" 的值。如果调用成功,我们将打印 `b` 的值并释放分配的内存。
请注意,在使用指针进行内存分配和操作时,务必小心并进行适当的错误处理。以上代码仅供参考,实际情况可能需要根据具体需求进行适当修改。
阅读全文
相关推荐
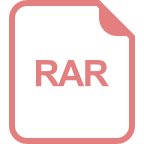
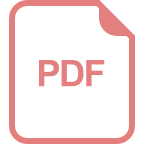
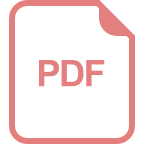











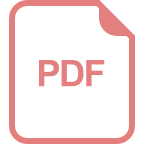
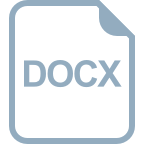
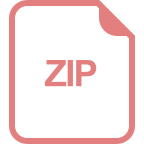