easyx 滑动拼图游戏
时间: 2023-12-14 13:03:48 浏览: 31
以下是使用easyx制作滑动拼图游戏的示例代码:
```python
from easygraphics import *
import random
# 定义常量
WIDTH = 400
HEIGHT = 400
SIZE = 100
ROWS = 4
COLS = 4
BLANK_ROW = 3
BLANK_COL = 3
FONT_SIZE = 30
FONT_COLOR = "white"
FONT_FAMILY = "Arial"
IMAGE_PATH = "puzzle.jpg"
# 加载图片
img = Image.open(IMAGE_PATH)
img = img.resize((WIDTH, HEIGHT))
img.save("puzzle.jpg")
# 初始化窗口
init_graph(WIDTH, HEIGHT, "Sliding Puzzle")
# 定义方块类
class Block:
def __init__(self, row, col, img):
self.row = row
self.col = col
self.img = img
self.x = col * SIZE
self.y = row * SIZE
self.selected = False
def draw(self):
if not self.selected:
set_fill_color("white")
draw_rectangle(self.x, self.y, self.x + SIZE, self.y + SIZE)
set_color("black")
draw_rectangle(self.x, self.y, self.x + SIZE, self.y + SIZE)
draw_image(self.x, self.y, self.img.crop((self.col * SIZE, self.row * SIZE, (self.col + 1) * SIZE, (self.row + 1) * SIZE)))
else:
set_fill_color("red")
draw_rectangle(self.x, self.y, self.x + SIZE, self.y + SIZE)
set_color("black")
draw_rectangle(self.x, self.y, self.x + SIZE, self.y + SIZE)
draw_image(self.x, self.y, self.img.crop((self.col * SIZE, self.row * SIZE, (self.col + 1) * SIZE, (self.row + 1) * SIZE)))
def is_clicked(self, x, y):
if x >= self.x and x <= self.x + SIZE and y >= self.y and y <= self.y + SIZE:
return True
else:
return False
def move(self, row, col):
self.row = row
self.col = col
self.x = col * SIZE
self.y = row * SIZE
# 初始化方块列表
blocks = []
for row in range(ROWS):
for col in range(COLS):
if row == BLANK_ROW and col == BLANK_COL:
blocks.append(None)
else:
block_img = img.crop((col * SIZE, row * SIZE, (col + 1) * SIZE, (row + 1) * SIZE))
blocks.append(Block(row, col, block_img))
# 随机打乱方块
for i in range(100):
blank_index = BLANK_ROW * COLS + BLANK_COL
move_index = random.choice([blank_index - 1, blank_index + 1, blank_index - COLS, blank_index + COLS])
if move_index >= 0 and move_index < ROWS * COLS:
blocks[move_index], blocks[blank_index] = blocks[blank_index], blocks[move_index]
blocks[move_index].move(move_index // COLS, move_index % COLS)
blocks[blank_index] = None
BLANK_ROW = blank_index // COLS
BLANK_COL = blank_index % COLS
# 游戏循环
while is_run():
# 绘制方块
for block in blocks:
if block is not None:
block.draw()
# 绘制文字
set_font_size(FONT_SIZE)
set_font_color(FONT_COLOR)
set_font_family(FONT_FAMILY)
draw_text(WIDTH // 2, HEIGHT - FONT_SIZE, "Sliding Puzzle", Align.CENTER)
# 处理鼠标事件
if has_mouse_msg():
msg = get_mouse_msg()
if msg.type == MouseMessage.DOWN:
for block in blocks:
if block is not None and block.is_clicked(msg.x, msg.y):
if block.row == BLANK_ROW and block.col == BLANK_COL - 1:
block.move(BLANK_ROW, BLANK_COL)
blocks[BLANK_ROW * COLS + BLANK_COL] = block
blocks[BLANK_ROW * COLS + BLANK_COL - 1] = None
BLANK_COL -= 1
elif block.row == BLANK_ROW and block.col == BLANK_COL + 1:
block.move(BLANK_ROW, BLANK_COL)
blocks[BLANK_ROW * COLS + BLANK_COL] = block
blocks[BLANK_ROW * COLS + BLANK_COL + 1] = None
BLANK_COL += 1
elif block.row == BLANK_ROW - 1 and block.col == BLANK_COL:
block.move(BLANK_ROW, BLANK_COL)
blocks[BLANK_ROW * COLS + BLANK_COL] = block
blocks[(BLANK_ROW - 1) * COLS + BLANK_COL] = None
BLANK_ROW -= 1
elif block.row == BLANK_ROW + 1 and block.col == BLANK_COL:
block.move(BLANK_ROW, BLANK_COL)
blocks[BLANK_ROW * COLS + BLANK_COL] = block
blocks[(BLANK_ROW + 1) * COLS + BLANK_COL] = None
BLANK_ROW += 1
break
delay_fps(60)
# 关闭窗口
close_graph()
```
运行上述代码后,即可得到一个滑动拼图游戏窗口。玩家可以通过鼠标点击方块来移动它们,直到拼图完成。
相关推荐
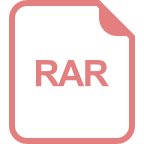














