用c语言写出实验题目1的代码,输入任何多项式都能保证运行
时间: 2024-10-20 15:15:44 浏览: 32
以下是使用C语言实现的一元稀疏多项式的基本操作的示例代码。该代码实现了多项式的创建、销毁、输出、加法和减法功能。为了简洁起见,这里假设多项式的系数和指数都是整数。
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct Node {
int coef; // 系数
int exp; // 指数
struct Node *next;
} PolyNode;
// 创建一个新节点
PolyNode* createNode(int coef, int exp) {
PolyNode *newNode = (PolyNode*)malloc(sizeof(PolyNode));
newNode->coef = coef;
newNode->exp = exp;
newNode->next = NULL;
return newNode;
}
// 销毁多项式
void destroyPoly(PolyNode *head) {
while (head != NULL) {
PolyNode *temp = head;
head = head->next;
free(temp);
}
}
// 插入节点到多项式中
void insertNode(PolyNode **head, int coef, int exp) {
PolyNode *newNode = createNode(coef, exp);
if (*head == NULL || (*head)->exp < exp) {
newNode->next = *head;
*head = newNode;
} else {
PolyNode *current = *head;
while (current->next != NULL && current->next->exp > exp) {
current = current->next;
}
if (current->next != NULL && current->next->exp == exp) {
current->next->coef += coef;
if (current->next->coef == 0) {
PolyNode *temp = current->next;
current->next = temp->next;
free(temp);
}
} else {
newNode->next = current->next;
current->next = newNode;
}
}
}
// 输出多项式
void printPoly(PolyNode *head) {
if (head == NULL) {
printf("0\n");
return;
}
for (PolyNode *p = head; p != NULL; p = p->next) {
if (p != head) {
if (p->coef > 0) {
printf(" + ");
} else {
printf(" - ");
p->coef = -p->coef;
}
}
if (p->exp == 0) {
printf("%d", p->coef);
} else if (p->exp == 1) {
if (p->coef != 1) {
printf("%dx", p->coef);
} else {
printf("x");
}
} else {
if (p->coef != 1) {
printf("%dx^%d", p->coef, p->exp);
} else {
printf("x^%d", p->exp);
}
}
}
printf("\n");
}
// 多项式相加
PolyNode* addPoly(PolyNode *poly1, PolyNode *poly2) {
PolyNode *result = NULL;
while (poly1 != NULL && poly2 != NULL) {
if (poly1->exp > poly2->exp) {
insertNode(&result, poly1->coef, poly1->exp);
poly1 = poly1->next;
} else if (poly1->exp < poly2->exp) {
insertNode(&result, poly2->coef, poly2->exp);
poly2 = poly2->next;
} else {
int sum = poly1->coef + poly2->coef;
if (sum != 0) {
insertNode(&result, sum, poly1->exp);
}
poly1 = poly1->next;
poly2 = poly2->next;
}
}
while (poly1 != NULL) {
insertNode(&result, poly1->coef, poly1->exp);
poly1 = poly1->next;
}
while (poly2 != NULL) {
insertNode(&result, poly2->coef, poly2->exp);
poly2 = poly2->next;
}
return result;
}
// 多项式相减
PolyNode* subPoly(PolyNode *poly1, PolyNode *poly2) {
PolyNode *negPoly2 = NULL;
for (PolyNode *p = poly2; p != NULL; p = p->next) {
insertNode(&negPoly2, -p->coef, p->exp);
}
return addPoly(poly1, negPoly2);
}
int main() {
PolyNode *poly1 = NULL;
PolyNode *poly2 = NULL;
// 输入多项式1
int n1;
printf("Enter the number of terms in polynomial 1: ");
scanf("%d", &n1);
for (int i = 0; i < n1; i++) {
int coef, exp;
printf("Enter coefficient and exponent: ");
scanf("%d %d", &coef, &exp);
insertNode(&poly1, coef, exp);
}
// 输入多项式2
int n2;
printf("Enter the number of terms in polynomial 2: ");
scanf("%d", &n2);
for (int i = 0; i < n2; i++) {
int coef, exp;
printf("Enter coefficient and exponent: ");
scanf("%d %d", &coef, &exp);
insertNode(&poly2, coef, exp);
}
// 输出多项式
printf("Polynomial 1: ");
printPoly(poly1);
printf("Polynomial 2: ");
printPoly(poly2);
// 多项式相加
PolyNode *sum = addPoly(poly1, poly2);
printf("Sum: ");
printPoly(sum);
// 多项式相减
PolyNode *diff = subPoly(poly1, poly2);
printf("Difference: ");
printPoly(diff);
// 销毁多项式
destroyPoly(poly1);
destroyPoly(poly2);
destroyPoly(sum);
destroyPoly(diff);
return 0;
}
```
### 说明
1. **创建节点**:`createNode` 函数用于创建新的多项式节点。
2. **插入节点**:`insertNode` 函数将节点按指数降序插入多项式链表中,并处理相同指数的合并。
3. **销毁多项式**:`destroyPoly` 函数释放多项式链表占用的内存。
4. **输出多项式**:`printPoly` 函数以标准形式输出多项式。
5. **多项式相加**:`addPoly` 函数实现两个多项式的相加。
6. **多项式相减**:`subPoly` 函数通过先取第二个多项式的负值再相加来实现相减。
### 使用方法
1. 运行程序后,首先输入第一个多项式的项数。
2. 输入每个项的系数和指数。
3. 同样步骤输入第二个多项式的项数和每个项的系数和指数。
4. 程序会输出两个多项式、它们的和与差。
希望这段代码能满足您的需求!如果有任何问题或需要进一步的帮助,请随时告诉我。
阅读全文
相关推荐
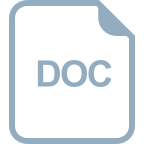
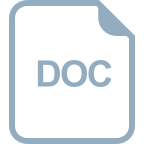
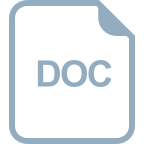

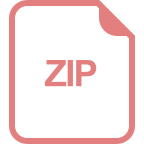
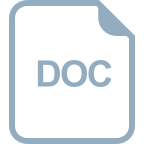
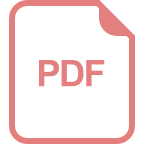
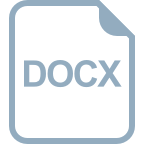
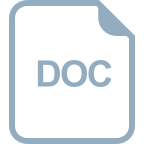
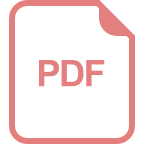
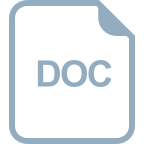
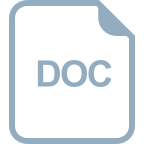
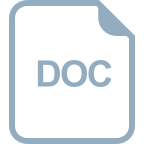

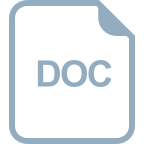
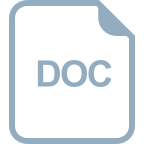
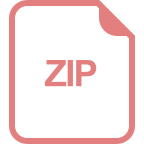
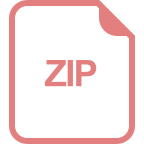
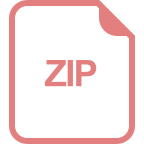