C语言(编程题)将字符串中的小写字母转换为对应的大写字母,其它字符不变 输入描述: 输入为多行,每行为一个字符串,字符串只由字母和数字组成,长度不超过80。输入以“End of file”结束。
时间: 2024-09-14 16:10:40 浏览: 55
在C语言中,你可以使用标准库函数`toupper()`来实现这个功能。`toupper()`函数接收一个字符作为参数,并返回大写的版本,如果该字符已经是大写,则保持原样。下面是一个简单的示例程序,它会遍历字符串并将所有小写字母转换为大写字母:
```c
#include <stdio.h>
#include <ctype.h> // 包含 toupper 函数
void convert_to_uppercase(char *str) {
int i;
for (i = 0; str[i] != '\0'; ++i) { // 遍历字符串直到遇到'\0'
if (islower(str[i])) { // 检查字符是否为小写
str[i] = toupper(str[i]); // 转换为大写
}
}
}
int main() {
char input[81]; // 存储单个字符串
while (fgets(input, sizeof(input), stdin)) { // 从标准输入读取一行
input[strcspn(input, "\n")] = '\0'; // 移除行尾的换行符
convert_to_uppercase(input); // 调用转换函数
printf("%s\n", input); // 打印结果
}
return 0;
}
相关问题
用c语言5-2 字符串字母大小写转换 题目描述 编写程序,将字符串中小写字母字符转换为大写。 输入格式: 在一行中输入一个字符串(不超过 80 个字符)。 输出格式: 将字符串中小写字母字符转换为大写后输出。 输入样例: strings 输出样例: STRINGS
以下是 C 语言代码实现:
```c
#include <stdio.h>
#include <string.h>
#include <ctype.h>
int main()
{
char s[81];
scanf("%s", s);
for (int i = 0; i < strlen(s); i++) {
if (islower(s[i])) {
s[i] = toupper(s[i]);
}
}
printf("%s\n", s);
return 0;
}
```
这里使用了 C 语言内置的 `islower()` 函数和 `toupper()` 函数。`islower()` 函数用于判断一个字符是否是小写字母,`toupper()` 函数用于将一个小写字母转换为大写字母。
遍历字符串中的每一个字符,如果是小写字母,则使用 `toupper()` 函数将其转换为大写字母。最后输出转换后的字符串即可。
如果输入样例为 strings,则输出为 STRINGS。
c语言,将字符串中的小写字母转换为对应的大写字母 其他字符不变
可以使用C语言中的字符数组和循环结构来实现将字符串中的小写字母转换为对应的大写字母,其他字符不变。可以使用标准库函数toupper()将小写字母转换为大写字母。
下面是一个示例代码:
```c
#include <stdio.h>
#include <ctype.h>
int main() {
char str[100];
int i = 0;
printf("请输入一个字符串:");
scanf("%s", str);
while (str[i]) {
if (islower(str[i])) { // 判断是否为小写字母
str[i] = toupper(str[i]); // 将小写字母转换为大写字母
}
i++;
}
printf("转换后的字符串为:%s\n", str);
return 0;
}
```
运行示例:
```
请输入一个字符串:hello world
转换后的字符串为:HELLO WORLD
```
阅读全文
相关推荐
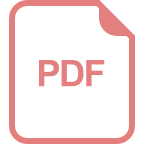
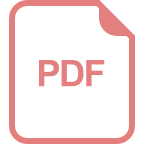



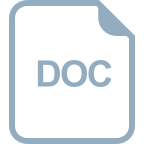









