用c语言程序实现求解表达式的值,例如输入1+2*3-16/2,则输出为-1。(40分)
时间: 2024-10-18 21:10:27 浏览: 28
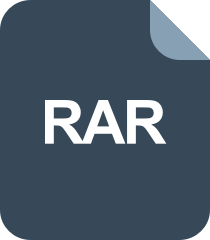
逆波兰表达式的求解:输入逆波兰表达式,输出结果
在C语言中,实现一个简单的表达式求值器通常需要利用递归函数以及栈来存储操作数和运算符。这里是一个基本的示例,用于计算这种类型的算术表达式:
```c
#include <stdio.h>
#include <stdlib.h>
#include <ctype.h>
// 定义数据结构存储操作数和运算符
typedef enum { NUM, OP } token_type;
struct exp {
token_type type;
union {
int number;
char op; // 运算符
};
};
// 函数处理输入流并解析表达式
struct exp* parse(char* input);
// 根据操作符优先级进行计算
int apply_op(int a, int b, char op);
// 计算表达式的值
int calculate(struct exp* exp);
int main() {
char input[50];
printf("请输入一个表达式 (如: 1+2*3-16/2): ");
fgets(input, sizeof(input), stdin);
struct exp* expr = parse(input);
int result = calculate(expr);
printf("结果: %d\n", result);
free(expr);
return 0;
}
// 解析表达式
struct exp* parse(char* input) {
struct exp* head = malloc(sizeof(*head));
head->type = NUM;
head->number = atoi(input); // 如果遇到数字,直接转换成整数
while (*input != '\0') {
if (isdigit(*input)) {
// 遇到数字,继续读取直到非数字字符
int num = 0;
while (isdigit(*(++input))) {
num *= 10;
num += *input - '0';
}
struct exp* new_node = malloc(sizeof(*new_node));
new_node->type = NUM;
new_node->number = num;
head->next = new_node;
head = new_node;
} else {
// 遇到运算符,创建新的节点并插入到适当的位置
struct exp* current = head;
while (current->next && current->next->type == OP &&
((current->next->op == '*' || current->next->op == '/') &&
(op_precedence(current->next->op) >= op_precedence(*input)))) {
current = current->next;
}
if (!current->next) {
// 将运算符添加到最后
current->next = malloc(sizeof(*current->next));
current->next->type = OP;
current->next->op = *input;
} else {
// 否则将当前节点的运算符和新运算符合并
current->next->op = apply_op(current->next->number, *input, current->next->op);
}
input++;
}
}
return head;
}
// 比较运算符的优先级
int op_precedence(char op) {
switch (op) {
case '+':
case '-':
return 1;
case '*':
case '/':
return 2;
default:
return 0;
}
}
// 应用运算符
int apply_op(int a, int b, char op) {
switch (op) {
case '+': return a + b;
case '-': return a - b;
case '*': return a * b;
case '/': return a / b; // 需要考虑除数为零的情况
default: return -1; // 非法运算符
}
}
// 计算整个表达式的值
int calculate(struct exp* exp) {
struct exp* temp = exp;
while (temp->next) {
if (temp->type == OP) {
temp->number = apply_op(temp->number, temp->next->number, temp->next->op);
free(temp->next);
temp = temp->prev;
} else {
temp = temp->next;
}
}
return temp->number;
}
```
阅读全文
相关推荐
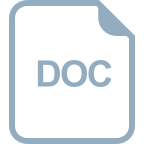
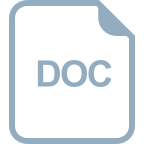















