Android 写一个消息 队列 用于socket 接发指令
时间: 2024-12-02 16:28:17 浏览: 14
在Android中,如果你需要通过Socket与服务器进行双向通信,并且希望管理发送和接收的消息,你可以创建一个基于`BlockingQueue`的消息队列。这里我们可以使用`ArrayBlockingQueue`作为基础,它是一个无界的阻塞队列,非常适合这种场景。下面是一个简单的例子:
```java
import java.io.IOException;
import java.io.OutputStream;
import java.nio.charset.StandardCharsets;
import java.util.concurrent.BlockingQueue;
import java.util.concurrent.LinkedBlockingQueue;
public class SocketMessageQueue {
private final BlockingQueue<String> messageQueue = new LinkedBlockingQueue<>();
private OutputStream outputStream;
private InputStream inputStream;
public SocketMessageQueue(OutputStream outputStream, InputStream inputStream) {
this.outputStream = outputStream;
this.inputStream = inputStream;
}
public synchronized void sendMessage(String message) throws IOException {
messageQueue.add(message);
sendDataToServer();
}
private synchronized void sendDataToServer() throws IOException {
String message = messageQueue.take(); // 取出队首消息
outputStream.write(message.getBytes(StandardCharsets.UTF_8));
outputStream.flush();
}
public synchronized String receiveMessage() throws IOException, InterruptedException {
byte[] bytes = new byte[4096]; // 假设最大接收长度
int readBytes = inputStream.read(bytes);
return new String(bytes, 0, readBytes, StandardCharsets.UTF_8);
}
// 在实际的网络连接上,你需要在适当的地方启动读取输入流的任务
// 同样地,在接收到新的消息后,也需要启动发送数据到服务端的任务
}
```
在这个例子中,`sendMessage`方法将消息添加到队列,然后立即尝试发送出去。`receiveMessage`方法从输入流中读取并返回消息。注意,你需要在后台维护一个循环,不断从队列中取出消息发送,同时监听输入流是否有新消息。
阅读全文
相关推荐
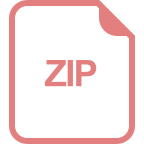
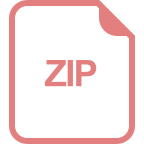
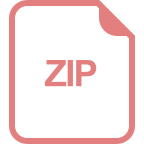















