C#实现当ssh远程执行一条命令时,在该进程尚未结束时远程结束掉该进程
时间: 2024-11-09 22:18:41 浏览: 9
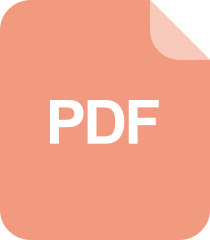
C#实现查杀本地与远程进程的方法
在C#中,要实现SSH远程执行命令并在进程尚未结束时强制终止,你可以使用第三方库如`SSH.NET`来连接SSH服务器,然后利用操作系统提供的API(如Windows的`System.Diagnostics.Process`)来控制远程进程。以下是一个简单的示例:
首先,确保安装了SSH.NET库,你可以通过NuGet包管理器添加:
```sh
Install-Package SSH.NET
```
然后,编写一个方法来执行命令和控制进程:
```csharp
using SSH.NET;
using System.Diagnostics;
public void RemoteExecuteAndKill(string host, string user, string password, string command, int timeoutSeconds)
{
using (var client = new SshClient(host))
{
client.Connect(user, password);
// 执行命令
var startInfo = new ProcessStartInfo("bash", $"-c \"{command}\"");
var process = client.ExecuteCommand(startInfo);
try
{
// 等待进程运行指定时间,如果超时,则强制终止
if (!process.WaitForExit(timeoutSeconds * 1000)) // 考虑将毫秒转换为秒
{
process.Kill(); // 强制停止进程
Console.WriteLine($"[Warning] Command {command} took longer than {timeoutSeconds} seconds and was killed.");
}
else
{
Console.WriteLine($"Command {command} completed successfully.");
}
}
catch (Exception ex)
{
Console.WriteLine($"Error executing command: {ex.Message}");
}
client.Disconnect();
}
}
```
在这个例子中,你需要替换`host`, `user`, 和 `password`为你的SSH服务器信息。注意,这只是一个基础示例,实际使用时需要处理更多的错误场景,并考虑权限问题。
阅读全文
相关推荐
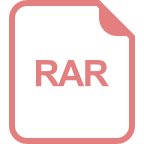
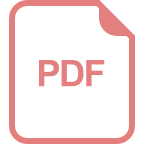




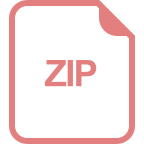
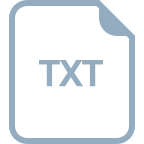
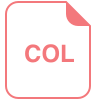


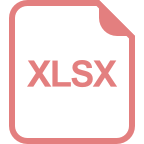
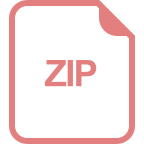
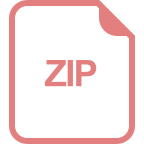
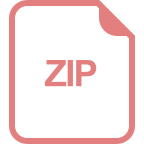
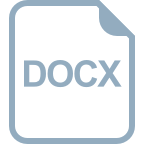
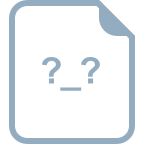