用C语言写一个程序 用孩子兄弟法表示一棵树或者一个森林,编写函数输出其深度 函数接口定义: int Get_Depth_Of_CSTree(CSTree CST); 其中 T是用户传入的参数,表示二叉树根节点的地址。 每行给出不多于N(N<31)个数,对应一棵树。
时间: 2024-10-25 20:11:15 浏览: 18
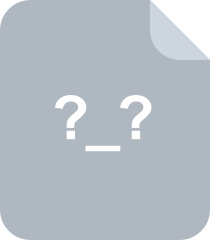
C语言程序设计-编写函数fun将一个数组中的值按逆序存放,并在main()函数中输出;

在C语言中,我们可以使用结构体来表示二叉树,并通过递归的方式来计算树的深度。首先,我们定义一个`CSTree`结构体,表示二叉树的一个节点:
```c
typedef struct {
int data; // 节点数据
CSTree* left; // 左子树指针
CSTree* right; // 右子树指针
} CSTree;
```
然后,编写`Get_Depth_Of_CSTree`函数来获取整棵树的深度:
```c
int Get_Depth_Of_CSTree(CSTree* T) {
if (T == NULL) { // 空树的情况,深度为0
return 0;
} else {
// 如果不是空树,递归地计算左子树和右子树的深度,取较大值加1作为当前节点的深度
int left_depth = Get_Depth_Of_CSTree(T->left);
int right_depth = Get_Depth_Of_CSTree(T->right);
return (left_depth > right_depth ? left_depth : right_depth) + 1;
}
}
```
为了处理森林(由多个树组成),你可以先创建一个数组或链表来存储所有的树根节点,然后遍历这个数组调用上述函数:
```c
void PrintForest(int N, CSTree** forest) {
for (int i = 0; i < N; i++) {
printf("Tree %d depth: %d\n", i+1, Get_Depth_Of_CSTree(forest[i]));
}
}
// 使用示例:
int main() {
CSTree trees[3] = {
{1, &trees[1], &trees[2]}, // 树1有两棵子树
{2, NULL, NULL}, // 子树为空
{3, NULL, NULL} // 子树为空
};
PrintForest(3, trees);
return 0;
}
```
在这个例子中,`PrintForest`函数会打印出每个树的索引及其对应的深度。
阅读全文
相关推荐
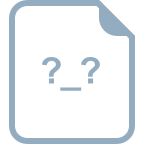
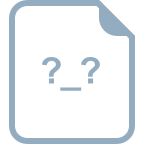




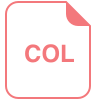
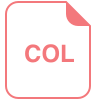
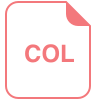
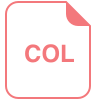
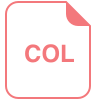






