watch_folder = r'D:/gangko/zip' upload_url = 'http://192.168.1.226:6060/dz' class FileEventHandler(FileSystemEventHandler): def on_created(self, event): if not event.is_directory: file_path = event.src_path with open(file_path, 'rb') as file: files = {'file': file} response = requests.post(upload_url, files=files) print(f"Uploaded {file_path}: {response.status_code}") if name == "main": event_handler = FileEventHandler() observer = Observer() observer.schedule(event_handler, watch_folder, recursive=True) try: observer.start() while True: time.sleep(1) except KeyboardInterrupt: observer.stop() observer.join()这是上传的代码@app.route('/dz', methods=['POST']) def dezip(): now = datetime.now() current_time = now.strftime("%Y-%m-%d-%H-%M-%S") target_folder = (os.path.join(r'D:/backup', current_time)) os.mkdir(target_folder) files = request.files.getlist('file') for file in files: filename = file.filename file_path = os.path.join(target_folder, filename) file.save(file_path) return jsonify({"code": 200, "msg": "success"}) if name == 'main': app.run(host='0.0.0.0', port=6060) 这是接收压缩文件的代码,为什么文件会损坏?
时间: 2024-04-16 16:25:34 浏览: 22
根据你提供的代码,无法确定为什么文件会损坏。这段代码是一个简单的文件上传和保存的过程。
在上传的代码中,接收到的文件被保存在目标文件夹 `target_folder` 中。这段代码没有涉及到对文件进行压缩或解压缩的操作。因此,在这段代码中,文件不应该出现损坏问题。
如果你发现上传后的文件损坏,可能是其他代码或操作导致的问题。以下是一些可能引起文件损坏的原因:
1. 文件在上传过程中被修改或损坏。你可以尝试手动上传文件并检查是否损坏。
2. 文件在保存到 `target_folder` 时发生了错误。你可以检查是否存在目标文件夹 `target_folder`,并确保该文件夹有适当的写入权限。
3. 文件在保存过程中发生了错误,可能是由于存储介质故障或其他硬件问题导致的。你可以尝试将文件保存到其他位置或设备上,以验证是否仍然出现损坏问题。
此外,还应该确保接收文件的服务正常工作,并且能够正确处理接收到的文件。
如果问题仍然存在,我建议你检查其他与文件上传、保存或处理相关的代码或操作。可能有其他部分的代码导致了文件损坏的问题。
希望能帮到你!如果还有其他问题,请随时提问。
相关问题
watch_folder = r'D:/zip' upload_url = 'http://192.168.1.226:6060/dz' def upload_file(file_path): with open(file_path, 'rb') as file: files = {'file': file} response = requests.post(upload_url, files=files) print(f"Uploaded {file_path}: {response.status_code}") def monitor_folder(): files = set() while True: current_files = set(os.listdir(watch_folder)) new_files = current_files - files for file in new_files: file_path = os.path.join(watch_folder, file) upload_file(file_path) files = current_files time.sleep(15) if __name__ == "__main__": monitor_folder() 加代码实现文件上传完成后将watch_folder = r'D:/zip'里的文件删除,而watch_folder = r'D:/zip'不动
要在文件上传完成后将 `watch_folder` 中的文件删除,可以在文件上传完成后使用 `os.remove()` 函数删除文件。以下是修改后的代码示例:
```python
import os
import time
import requests
watch_folder = r'D:/zip'
upload_url = 'http://192.168.1.226:6060/dz'
def upload_file(file_path):
with open(file_path, 'rb') as file:
files = {'file': file}
response = requests.post(upload_url, files=files)
print(f"Uploaded {file_path}: {response.status_code}")
def delete_file(file_path):
os.remove(file_path)
print(f"Deleted {file_path}")
def monitor_folder():
files = set()
while True:
current_files = set(os.listdir(watch_folder))
new_files = current_files - files
for file in new_files:
file_path = os.path.join(watch_folder, file)
upload_file(file_path)
delete_file(file_path)
files = current_files
time.sleep(15)
if __name__ == "__main__":
monitor_folder()
```
在上述代码中,我添加了一个名为 `delete_file()` 的新函数,用于删除已上传的文件。在上传完成后调用 `delete_file()` 函数,将已上传的文件从 `watch_folder` 中删除。
请注意,删除文件是一个不可逆的操作,请谨慎使用。确保你在明确了解代码功能和操作的情况下进行操作,并备份重要文件。
希望对你有所帮助!如果还有其他问题,请随时提问。
watch_folder = r'D:/gangko/zip' upload_url = 'http://192.168.1.226:6060/dz' class FileEventHandler(FileSystemEventHandler): def on_created(self, event): if not event.is_directory: file_path = event.src_path with open(file_path, 'rb') as file: files = {'file': file} response = requests.post(upload_url, files=files) print(f"Uploaded {file_path}: {response.status_code}") if __name__ == "__main__": event_handler = FileEventHandler() observer = Observer() observer.schedule(event_handler, watch_folder, recursive=True) try: observer.start() while True: time.sleep(1) except KeyboardInterrupt: observer.stop() observer.join() 为什么这段代码拷贝的压缩文件都损坏了
根据你提供的代码,我看不出为什么拷贝的压缩文件会损坏。这段代码是一个简单的文件监视器,当在 `watch_folder` 目录下创建新文件时,它会将文件上传到 `upload_url` 指定的地址。没有看到对文件进行压缩或解压缩的操作。
如果你发现拷贝的压缩文件都损坏了,可能是其他部分的代码或操作导致的问题。请确保以下几点:
1. 确保文件在拷贝过程中没有被修改或损坏。可以尝试手动拷贝文件并检查是否损坏。
2. 确保上传到 `upload_url` 的代码没有对文件进行修改、损坏或解压缩操作。
3. 检查 `upload_url` 目标地址和接收文件的服务是否正常工作,并且能够正确处理接收到的文件。
如果问题仍然存在,我建议你检查其他与文件拷贝、压缩或上传相关的代码或操作。可能有其他部分的代码导致了文件损坏的问题。
希望能帮到你!如果还有其他问题,请随时提问。
相关推荐
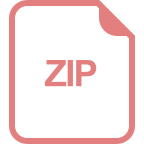













