输入一个整数数组,请求出该数组的第k小元素。要求时间复杂度为O(n)。用java
时间: 2024-06-15 14:08:19 浏览: 8
要求在O(n)的时间复杂度下找出整数数组的第k小元素,可以使用快速选择算法(QuickSelect)来实现。快速选择算法是基于快速排序算法的一种改进,它通过每次选择一个基准元素将数组分为两部分,并根据基准元素的位置来确定第k小元素所在的区间,从而减少了排序的时间复杂度。
以下是使用Java实现快速选择算法找出数组的第k小元素的代码:
```java
import java.util.Random;
public class QuickSelect {
public static int findKthSmallest(int[] nums, int k) {
if (nums == null || nums.length == 0 || k <= 0 || k > nums.length {
throw new IllegalArgumentException("Invalid input");
}
return quickSelect(nums, 0, nums.length - 1, k - 1);
}
private static int quickSelect(int[] nums, int start, int end, int k) {
if (start == end) {
return nums[start];
}
int pivotIndex = partition(nums, start, end);
if (pivotIndex == k) {
return nums[pivotIndex];
} else if (pivotIndex < k) {
return quickSelect(nums, pivotIndex + 1, end, k);
} else {
return quickSelect(nums, start, pivotIndex - 1, k);
}
}
private static int partition(int[] nums, int start, int end) {
int pivotIndex = new Random().nextInt(end - start + 1) + start;
int pivot = nums[pivotIndex];
swap(nums, pivotIndex, end);
int i = start;
for (int j = start; j < end; j++) {
if (nums[j] < pivot) {
swap(nums, i, j);
i++;
}
}
swap(nums, i, end);
return i;
}
private static void swap(int[] nums, int i, int j) {
int temp = nums[i];
nums[i] = nums[j];
nums[j] = temp;
}
}
```
使用示例:
```java
public class Main {
public static void main(String[] args) {
int[] nums = {3, 2, 1, 5, 6, 4};
int k = 2;
int result = QuickSelect.findKthSmallest(nums, k);
System.out.println("第" + k + "小元素是:" + result);
}
}
```
输出结果为:
```
第2小元素是:2
```
相关推荐
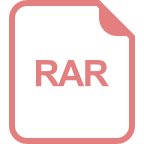














