pandas converters
时间: 2024-01-19 14:18:19 浏览: 105
在pandas中,converters是read_csv函数的一个参数,用于指定对特定列进行数据类型转换的函数。通过converters参数,可以将读取的数据中的某些列转换为指定的数据类型。
以下是一个示例,演示如何使用converters参数将百分比字符串转换为数字:
```python
import pandas as pd
# 定义转换函数,将百分比字符串转换为浮点数
def percentage_to_float(val):
return float(val.rstrip("%")) / 100
# 读取CSV文件,并将"A"列的百分比字符串转换为浮点数
df = pd.read_csv("my_data.txt", converters={"A": percentage_to_float})
# 打印转换后的数据
print(df)
```
输出结果:
```
A B
0 0.10 3
1 0.15 4
```
在上述示例中,我们定义了一个名为percentage_to_float的转换函数,该函数将百分比字符串转换为浮点数。然后,我们使用read_csv函数读取了一个名为my_data.txt的CSV文件,并通过converters参数将"A"列的数据类型转换为浮点数。最后,我们打印了转换后的数据。
相关问题
UnicodeDecodeError Traceback (most recent call last) <ipython-input-13-d8bda818b845> in <module> 1 import pandas as pd 2 from IPython.display import display ----> 3 data = pd.read_csv('goods.csv', encoding='utf-8') 4 data.insert(2, 'goods', '') 5 def get_goods(title): C:\u01\anaconda3\lib\site-packages\pandas\io\parsers.py in read_csv(filepath_or_buffer, sep, delimiter, header, names, index_col, usecols, squeeze, prefix, mangle_dupe_cols, dtype, engine, converters, true_values, false_values, skipinitialspace, skiprows, skipfooter, nrows, na_values, keep_default_na, na_filter, verbose, skip_blank_lines, parse_dates, infer_datetime_format, keep_date_col, date_parser, dayfirst, cache_dates, iterator, chunksize, compression, thousands, decimal, lineterminator, quotechar, quoting, doublequote, escapechar, comment, encoding, dialect, error_bad_lines, warn_bad_lines, delim_whitespace, low_memory, memory_map, float_precision, storage_options) 608 kwds.update(kwds_defaults) 609 --> 610 return _read(filepath_or_buffer, kwds) 611 612 C:\u01\anaconda3\lib\site-packages\pandas\io\parsers.py in _read(filepath_or_buffer, kwds) 460 461 # Create the parser. --> 462 parser = TextFileReader(filepath_or_buffer, **kwds) 463 464 if chunksize or iterator: C:\u01\anaconda3\lib\site-packages\pandas\io\parsers.py in __init__(self, f, engine, **kwds) 817 self.options["has_index_names"] = kwds["has_index_names"] 818 --> 819 self._engine = self._make_engine(self.engine) 820 821 def close(self): C:\u01\anaconda3\lib\site-packages\pandas\io\parsers.py in _make_engine(self, engine) 1048 ) 1049 # error: Too many arguments for "ParserBase" -> 1050 return mapping[engine](self.f, **self.options) # type: ignore[call-arg] 1051 1052 def _failover_to_python(self): C:\u01\anaconda3\lib\site-packages\pandas\io\parsers.py in __init__(self, src, **kwds) 1896 1897 try: -> 1898 self._reader = parsers.TextReader(self.handles.handle, **kwds) 1899 except Exception: 1900 self.handles.close() pandas\_libs\parsers.pyx in pandas._libs.parsers.TextReader.__cinit__() pandas\_libs\parsers.pyx in pandas._libs.parsers.TextReader._get_header() pandas\_libs\parsers.pyx in pandas._libs.parsers.TextReader._tokenize_rows() pandas\_libs\parsers.pyx in pandas._libs.parsers.raise_parser_error() UnicodeDecodeError: 'utf-8' codec can't decode byte 0xca in position 83: invalid continuation byte
这个错误通常是由于在读取文件时指定的编码与实际编码不匹配导致的。在这种情况下,建议尝试使用其他编码格式重新读取文件。你可以尝试使用 "GBK" 或 "GB2312" 等中文编码格式。例如,你可以这样修改代码:
```python
data = pd.read_csv('goods.csv', encoding='GBK')
```
如果还是无法解决问题,建议检查文件本身是否存在编码问题,可能需要在其他编辑器中重新保存文件并指定正确的编码格式。
pandas读取excel、
pandas是一个强大的Python数据分析库,可以用来读取和处理各种数据格式,包括Excel文件。下面是使用pandas读取Excel文件的方法:
1.首先需要安装pandas库,可以使用以下命令进行安装:
```shell
pip install pandas
```
2.使用pandas.read_excel()函数读取Excel文件,该函数的参数包括:
- io:Excel文件的路径或URL。
- sheet_name:要读取的工作表的名称或索引。默认为0,即第一个工作表。
- header:指定哪一行作为列名。默认为0,即第一行。
- names:指定列名。如果header=None,则必须指定列名。
- index_col:指定哪一列作为行索引。
- usecols:指定要读取的列。
- dtype:指定每列的数据类型。
- parse_dates:指定哪些列需要解析为日期。
- na_values:指定哪些值应该被视为缺失值。
- converters:指定每列的转换器函数。
- skiprows:指定要跳过的行数。
- nrows:指定要读取的行数。
- true_values、false_values:指定哪些值应该被视为True和False。
下面是一个读取Excel文件的例子:
```python
import pandas as pd
# 读取Excel文件
df = pd.read_excel('example.xlsx', sheet_name='Sheet1', header=0, index_col=None)
# 打印前5行数据
print(df.head())
```
阅读全文
相关推荐
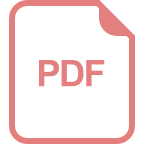
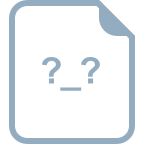
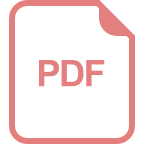
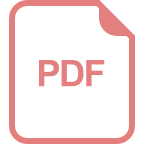
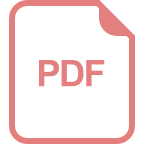









