用C语言实现两个多项式相乘运算
时间: 2024-10-10 08:02:43 浏览: 53
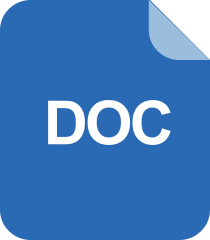
数据结构(C语言)用单链表存储一元多项式并实现两个多项式的相加运算.doc

在C语言中,我们可以使用数组来表示多项式的系数,然后通过循环结构来实现两个多项式的乘法。由于多项式乘法涉及到每个系数的逐项相乘并累加,所以通常会创建一个新的数组来存储结果。这里是一个简单的示例:
```c
#include <stdio.h>
#define MAX_TERMS 50 // 用于存储最大项数
// 函数声明
void multiplyPolynomials(int* poly1, int* poly2, int* result, int n1, int n2);
int main() {
int poly1[MAX_TERMS], poly2[MAX_TERMS], result[MAX_TERMS * MAX_TERMS];
int n1 = 3, n2 = 4; // 第一个多项式的阶数和第二个多项式的阶数
int numTerms = 0;
// 输入两个多项式的系数
printf("Enter coefficients for the first polynomial (separated by spaces): ");
for (int i = 0; i < n1 + 1; i++) {
scanf("%d", &poly1[i]);
}
printf("Enter coefficients for the second polynomial (separated by spaces): ");
for (int i = 0; i < n2 + 1; i++) {
scanf("%d", &poly2[i]);
}
// 乘法操作
multiplyPolynomials(poly1, poly2, result, n1, n2);
// 输出结果多项式的系数
printf("\nProduct of the polynomials:\n");
for (int i = 0; i <= n1 + n2; i++) {
if (result[i] != 0) {
printf("%dx^%d + ", result[i], i);
}
numTerms++;
if (numTerms % 5 == 0) { // 每五行显示一次
printf("\n");
}
}
return 0;
}
// 实现多项式乘法函数
void multiplyPolynomials(int* poly1, int* poly2, int* result, int n1, int n2) {
for (int i = 0; i <= n1; i++) {
for (int j = 0; j <= n2; j++) {
int term = poly1[i] * poly2[j]; // 相乘得到临时结果
result[i+j] += term; // 累加到相应位置
}
}
}
```
在这个例子中,`multiplyPolynomials`函数接受两个输入多项式的系数数组、结果数组以及它们的阶数,然后遍历每一个可能的乘积项并将它们加到结果数组对应的位置。
阅读全文
相关推荐
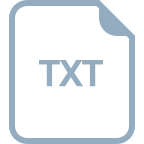
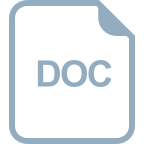

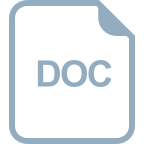
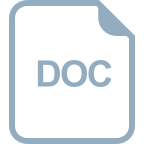


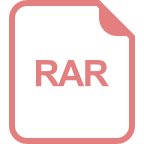
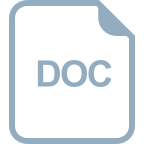
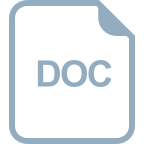
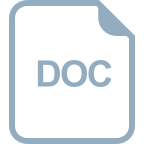
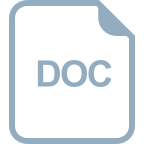
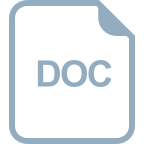
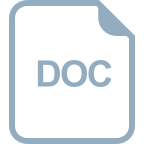
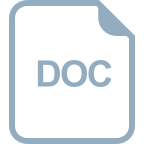
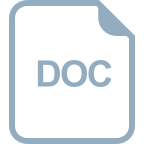
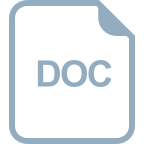
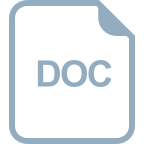