欧拉角转换成旋转矩阵c++
时间: 2023-09-13 19:06:49 浏览: 202
欧拉角到旋转矩阵的转换在C++中可以通过数学库来实现。以下是一个示例代码:
```cpp
#include <iostream>
#include <cmath>
#include <Eigen/Dense> // 使用Eigen库进行矩阵计算
using namespace Eigen;
// 欧拉角到旋转矩阵的转换函数
Matrix3d eulerToRotationMatrix(double roll, double pitch, double yaw) {
// 将欧拉角转换为弧度
double phi = roll * M_PI / 180.0;
double theta = pitch * M_PI / 180.0;
double psi = yaw * M_PI / 180.0;
Matrix3d rotationMatrix;
// 计算旋转矩阵的元素
rotationMatrix(0, 0) = cos(psi) * cos(theta);
rotationMatrix(0, 1) = -sin(psi) * cos(phi) + cos(psi) * sin(theta) * sin(phi);
rotationMatrix(0, 2) = sin(psi) * sin(phi) + cos(psi) * sin(theta) * cos(phi);
rotationMatrix(1, 0) = sin(psi) * cos(theta);
rotationMatrix(1, 1) = cos(psi) * cos(phi) + sin(psi) * sin(theta) * sin(phi);
rotationMatrix(1, 2) = -cos(psi) * sin(phi) + sin(psi) * sin(theta) * cos(phi);
rotationMatrix(2, 0) = -sin(theta);
rotationMatrix(2, 1) = cos(theta) * sin(phi);
rotationMatrix(2, 2) = cos(theta) * cos(phi);
return rotationMatrix;
}
int main() {
// 示例欧拉角
double roll = 45.0;
double pitch = 30.0;
double yaw = 60.0;
// 欧拉角到旋转矩阵的转换
Matrix3d rotationMatrix = eulerToRotationMatrix(roll, pitch, yaw);
// 输出旋转矩阵
std::cout << "Rotation Matrix:\n" << rotationMatrix << std::endl;
return 0;
}
```
在上述示例代码中,我们使用了Eigen库来进行矩阵计算。在`eulerToRotationMatrix`函数中,我们将欧拉角转换为弧度,并计算旋转矩阵的元素。最后,我们使用示例欧拉角调用`eulerToRotationMatrix`函数,并输出旋转矩阵。
请确保已经安装了Eigen库,并将其包含在代码中。你可以根据自己的需求修改示例代码。
阅读全文
相关推荐

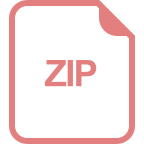
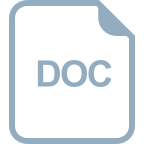


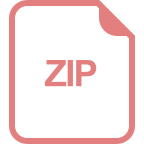




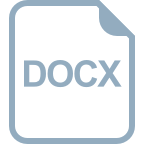
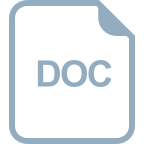
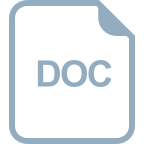
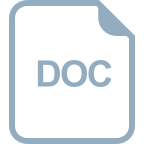

