判断是否为二叉排序树的c语言代码
时间: 2024-11-28 16:12:26 浏览: 37
判断一个二叉树是否为二叉排序树(BST)通常需要检查每个节点的左子树中的所有节点值都小于当前节点的值,而右子树中的所有节点值都大于当前节点的值。以下是C语言的一个简单代码实现,使用了递归的方式:
```c
#include <stdbool.h>
#include <stdio.h>
// 定义二叉树结点结构体
typedef struct Node {
int data;
struct Node* left;
struct Node* right;
} Node;
// 创建新节点
Node* newNode(int data) {
Node* node = (Node*)malloc(sizeof(Node));
node->data = data;
node->left = NULL;
node->right = NULL;
return node;
}
// 插入节点到BST,这里假设已经满足BST条件
Node* insert(Node* root, int data) {
if (root == NULL) {
return newNode(data);
}
if (data < root->data) {
root->left = insert(root->left, data);
} else if (data > root->data) {
root->right = insert(root->right, data);
}
return root;
}
// 判断给定的二叉树是否为BST
bool isValidBST(Node* root) {
return helper(root, INT_MIN, INT_MAX);
}
// 辅助函数,检查当前节点及左子树的所有节点值是否都在min到max范围内
bool helper(Node* node, int min, int max) {
if (node == NULL) {
return true;
}
if (node->data < min || node->data > max) {
return false;
}
return helper(node->left, min, node->data - 1) &&
helper(node->right, node->data + 1, max);
}
int main() {
Node* root = NULL;
// 插入数据,构建BST
root = insert(root, 4);
insert(root, 2);
insert(root, 6);
insert(root, 1);
insert(root, 3);
insert(root, 5);
insert(root, 7);
if (isValidBST(root)) {
printf("Given tree is a Binary Search Tree.\n");
} else {
printf("Given tree is not a Binary Search Tree.\n");
}
return 0;
}
阅读全文
相关推荐
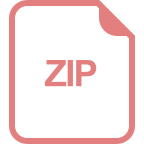
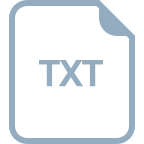
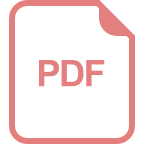


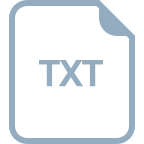












