用tkinter实现五子棋
时间: 2023-11-05 18:20:39 浏览: 58
可以使用tkinter库来实现五子棋游戏。首先,你需要创建一个游戏界面窗口,并在窗口中添加一个棋盘。然后,你可以通过点击棋盘上的位置来落子。接下来,你需要编写一些逻辑来判断游戏的胜负以及防止无效的落子。最后,你可以在游戏结束时显示胜利者或者平局的信息。
以下是一个简单的示例代码,可以帮助你开始实现五子棋游戏:
```python
import tkinter as tk
class Gobang:
def __init__(self, width=15, height=15):
self.width = width
self.height = height
self.board = [[0] * height for _ in range(width)]
self.player = 1
self.game_over = False
self.window = tk.Tk()
self.window.title("五子棋")
self.canvas = tk.Canvas(self.window, width=width*30, height=height*30, bg="white")
self.canvas.pack()
self.canvas.bind("<Button-1>", self.on_click)
self.draw_board()
def draw_board(self):
for i in range(self.width):
self.canvas.create_line(i*30, 0, i*30, self.height*30)
for i in range(self.height):
self.canvas.create_line(0, i*30, self.width*30, i*30)
def draw_piece(self, x, y):
if self.board[x][y] != 0:
return
color = "black" if self.player == 1 else "white"
self.canvas.create_oval(x*30-15, y*30-15, x*30+15, y*30+15, fill=color)
self.board[x][y] = self.player
if self.check_win(x, y):
self.game_over = True
winner = "黑棋" if self.player == 1 else "白棋"
self.canvas.create_text(self.width*15, self.height*15, text="{}胜利!".format(winner))
if not self.game_over:
self.player = 2 if self.player == 1 else 1
def check_win(self, x, y):
directions = [(1, 0), (0, 1), (1, 1), (1, -1)]
for dx, dy in directions:
count = 1
for i in range(1, 5):
new_x, new_y = x + i*dx, y + i*dy
if 0 <= new_x < self.width and 0 <= new_y < self.height and self.board[new_x][new_y] == self.player:
count += 1
else:
break
for i in range(1, 5):
new_x, new_y = x - i*dx, y - i*dy
if 0 <= new_x < self.width and 0 <= new_y < self.height and self.board[new_x][new_y] == self.player:
count += 1
else:
break
if count >= 5:
return True
return False
def on_click(self, event):
if self.game_over:
return
x = event.x // 30
y = event.y // 30
self.draw_piece(x, y)
def start(self):
self.window.mainloop()
game = Gobang()
game.start()
```
你可以运行上述代码来尝试玩五子棋游戏。在游戏窗口中,你可以点击棋盘上的位置来落子,黑棋先手。当有一方连成五子时,游戏结束并显示胜利者。
相关推荐
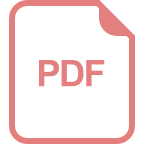
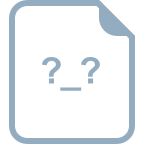
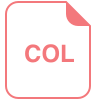
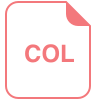
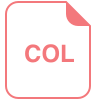
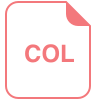
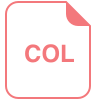









