unity控制网络摄像头打开
时间: 2023-09-13 20:14:04 浏览: 56
要在Unity中控制网络摄像头打开,您需要使用WebCamTexture类。
以下是一些简单的代码示例,可以在Unity中打开网络摄像头:
1. 首先,在Unity中创建一个Scene并添加一个空的GameObject。
2. 在Inspector窗格中,单击“Add Component”按钮并选择“WebCamTexture”。
3. 在“WebCamTexture”组件中,选择您要使用的网络摄像头并设置其他选项。
4. 在脚本中添加以下代码:
```
using UnityEngine;
using System.Collections;
public class CameraController : MonoBehaviour {
public WebCamTexture webcamTexture;
void Start () {
webcamTexture = new WebCamTexture();
GetComponent<Renderer>().material.mainTexture = webcamTexture;
webcamTexture.Play();
}
}
```
5. 在场景中将脚本添加到GameObject上。
6. 您应该能够看到网络摄像头的图像在Unity中打开。
请注意,此代码示例仅限于控制网络摄像头的打开。如果您需要更高级的功能,例如捕获摄像头图像或更改摄像头设置,请参阅WebCamTexture类文档以获取更多信息。
相关问题
unity ar 打开摄像头
要在Unity中打开摄像头,你需要使用Unity的AR工具包,例如Vuforia或AR Foundation。这些工具包都提供了API来访问摄像头,并在摄像头中实现增强现实。
以下是使用AR Foundation打开摄像头的示例代码:
```csharp
using UnityEngine;
using UnityEngine.XR.ARFoundation;
public class ARCameraController : MonoBehaviour
{
[SerializeField]
private ARCameraManager cameraManager;
void Start()
{
cameraManager.requestedFacingDirection = UnityEngine.XR.ARSubsystems.CameraFacingDirection.World;
cameraManager.startingRequestedLightEstimation = UnityEngine.XR.ARSubsystems.LightEstimation.AmbientIntensity;
cameraManager.frameReceived += OnFrameReceived;
}
void OnFrameReceived(ARCameraFrameEventArgs args)
{
// Process the camera frame here
}
}
```
在上面的示例代码中,我们首先获取了ARCameraManager对象,然后设置了所需的摄像头方向和光照估计。最后,我们注册了一个回调函数OnFrameReceived,该函数将在每个摄像头帧到达时被调用。在OnFrameReceived函数中,你可以访问摄像头帧并进行任何所需的处理。
请注意,这只是一个示例代码,你需要在自己的项目中进行适当的修改和调整。同时,你需要确保你的设备支持AR功能并且已经安装了所需的AR工具包。
unity3D控制摄像头移动
你可以使用以下代码来控制摄像头在平面上的移动:
```c#
public float speed = 10.0f; // 摄像头移动速度
public float rotationSpeed = 100.0f; // 摄像头旋转速度
void Update () {
float horizontalMovement = Input.GetAxis("Horizontal"); // 获取水平方向移动的输入值
float verticalMovement = Input.GetAxis("Vertical"); // 获取垂直方向移动的输入值
// 计算摄像头在平面上的移动向量
Vector3 moveDirection = new Vector3(horizontalMovement, 0, verticalMovement);
moveDirection = transform.TransformDirection(moveDirection);
moveDirection *= speed;
// 控制摄像头的移动
transform.position += moveDirection * Time.deltaTime;
// 控制摄像头的旋转
float rotation = Input.GetAxis("Mouse X") * rotationSpeed * Time.deltaTime;
transform.Rotate(0, rotation, 0);
}
```
在上面的代码中,我们使用了 `Input.GetAxis` 函数来获取玩家的输入值,并计算了摄像头在平面上的移动向量。然后使用 `transform.position` 来控制摄像头的移动,使用 `transform.Rotate` 来控制摄像头的旋转。你可以根据自己的需求调整代码中的参数。
相关推荐
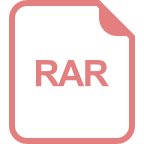
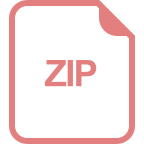
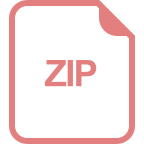












