libusb_bulk_transfer 事例
时间: 2023-06-28 07:12:02 浏览: 99
以下是使用libusb库进行bulk传输的示例代码:
```C
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <libusb-1.0/libusb.h>
#define VENDOR_ID 0x1234 // 你的设备的厂商ID
#define PRODUCT_ID 0x5678 // 你的设备的产品ID
#define ENDPOINT_IN 0x81 // 用于IN传输的端点地址
#define ENDPOINT_OUT 0x02 // 用于OUT传输的端点地址
#define MAX_PACKET_SIZE 64 // 最大数据包大小
int main(int argc, char *argv[]) {
libusb_device **list;
libusb_device_handle *handle = NULL;
int result, num_devices, i, transferred;
unsigned char buffer[256];
// 初始化libusb库
result = libusb_init(NULL);
if (result < 0) {
printf("libusb initialization failed\n");
return 1;
}
// 获取连接的USB设备列表
num_devices = libusb_get_device_list(NULL, &list);
if (num_devices < 0) {
printf("could not get device list\n");
libusb_exit(NULL);
return 1;
}
// 查找指定的USB设备
for (i = 0; i < num_devices; i++) {
libusb_device *device = list[i];
struct libusb_device_descriptor desc;
result = libusb_get_device_descriptor(device, &desc);
if (result < 0) {
printf("could not get device descriptor\n");
continue;
}
if (desc.idVendor == VENDOR_ID && desc.idProduct == PRODUCT_ID) {
// 打开设备
result = libusb_open(device, &handle);
if (result < 0) {
printf("could not open device\n");
continue;
}
// 重置设备
result = libusb_reset_device(handle);
if (result < 0) {
printf("could not reset device\n");
libusb_close(handle);
handle = NULL;
continue;
}
// claim interface
result = libusb_claim_interface(handle, 0);
if (result < 0) {
printf("could not claim interface\n");
libusb_close(handle);
handle = NULL;
continue;
}
// 向设备写入数据
memset(buffer, 0, sizeof(buffer));
buffer[0] = 0x01;
result = libusb_bulk_transfer(handle, ENDPOINT_OUT, buffer, MAX_PACKET_SIZE, &transferred, 0);
if (result < 0) {
printf("could not write data\n");
libusb_release_interface(handle, 0);
libusb_close(handle);
handle = NULL;
continue;
}
// 从设备读取数据
memset(buffer, 0, sizeof(buffer));
result = libusb_bulk_transfer(handle, ENDPOINT_IN, buffer, MAX_PACKET_SIZE, &transferred, 0);
if (result < 0) {
printf("could not read data\n");
libusb_release_interface(handle, 0);
libusb_close(handle);
handle = NULL;
continue;
}
// 处理读取到的数据
printf("received %d bytes of data: ", transferred);
for (i = 0; i < transferred; i++) {
printf("%02x ", buffer[i]);
}
printf("\n");
// 释放接口
libusb_release_interface(handle, 0);
// 关闭设备
libusb_close(handle);
handle = NULL;
break;
}
相关推荐
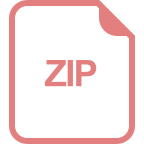














