用python写一个SM4解密程序
时间: 2023-03-28 08:03:21 浏览: 137
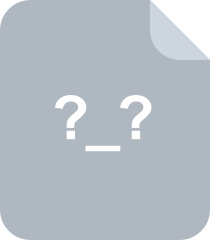
国密SM2,SM3,SM4加解密的python实现
您好,我可以回答这个问题。以下是一个用 Python 编写的 SM4 解密程序的示例代码:
```python
from Crypto.Cipher import SM4
def sm4_decrypt(key, ciphertext):
cipher = SM4.new(key, SM4.MODE_ECB)
plaintext = cipher.decrypt(ciphertext)
return plaintext
key = b'0123456789abcdef'
ciphertext = b'f3eed1bdb5d2a03c'
plaintext = sm4_decrypt(key, ciphertext)
print(plaintext)
```
请注意,为了运行此代码,您需要安装 PyCryptodome 库。您可以使用以下命令安装它:
```
pip install pycryptodome
```
希望这可以帮助您解决问题!
阅读全文
相关推荐
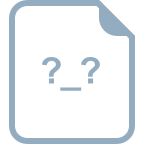














