帮我写一个上位机读取数据并保存为txt格式的代码
时间: 2024-03-13 16:44:53 浏览: 122
好的,以下是一个简单的 C# WinForms 上位机程序,可以读取串口数据并将数据保存到文本文件中:
```
using System;
using System.IO.Ports;
using System.Windows.Forms;
namespace SerialPortReader
{
public partial class MainForm : Form
{
private SerialPort serialPort;
public MainForm()
{
InitializeComponent();
serialPort = new SerialPort();
serialPort.DataReceived += SerialPort_DataReceived;
}
private void btnConnect_Click(object sender, EventArgs e)
{
if (!serialPort.IsOpen)
{
serialPort.PortName = cmbPortName.Text;
serialPort.BaudRate = int.Parse(cmbBaudRate.Text);
serialPort.DataBits = int.Parse(cmbDataBits.Text);
serialPort.Parity = (Parity)Enum.Parse(typeof(Parity), cmbParity.Text);
serialPort.StopBits = (StopBits)Enum.Parse(typeof(StopBits), cmbStopBits.Text);
serialPort.Open();
btnConnect.Text = "Disconnect";
}
else
{
serialPort.Close();
btnConnect.Text = "Connect";
}
}
private void SerialPort_DataReceived(object sender, SerialDataReceivedEventArgs e)
{
string data = serialPort.ReadExisting();
this.Invoke(new Action(() =>
{
txtData.AppendText(data);
}));
}
private void btnSave_Click(object sender, EventArgs e)
{
if (txtData.Text.Length > 0)
{
SaveFileDialog dialog = new SaveFileDialog();
dialog.Filter = "Text Files|*.txt";
dialog.Title = "Save Data";
if (dialog.ShowDialog() == DialogResult.OK)
{
string fileName = dialog.FileName;
System.IO.File.WriteAllText(fileName, txtData.Text);
MessageBox.Show("Data saved successfully.");
}
}
}
}
}
```
该程序包含以下控件:
- 一个 ComboBox,用于选择串口名称;
- 一个 ComboBox,用于选择波特率;
- 一个 ComboBox,用于选择数据位;
- 一个 ComboBox,用于选择校验位;
- 一个 ComboBox,用于选择停止位;
- 一个按钮,用于连接/断开串口;
- 一个文本框,用于显示串口数据;
- 一个按钮,用于保存数据到文本文件。
在程序启动时,会创建一个 SerialPort 对象,并将 DataReceived 事件处理函数绑定到该对象的事件处理程序上。当串口接收到数据时,会触发 DataReceived 事件,从而调用 SerialPort_DataReceived 函数,该函数将读取到的数据追加到文本框中。
当用户单击 Connect 按钮时,会根据配置的串口参数打开或关闭串口。当用户单击 Save 按钮时,会弹出一个 SaveFileDialog,允许用户选择要保存的文件路径和名称,并将文本框中的数据保存到选定的文件中。
请注意,该程序只是一个简单的示例,可能需要根据实际需求进行修改。特别是,在实际应用中,需要考虑错误处理、数据解析、界面优化等方面的问题。
阅读全文
相关推荐
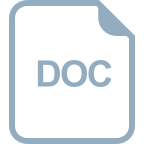
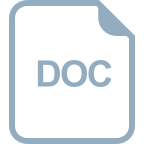
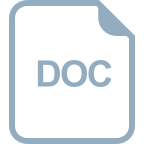
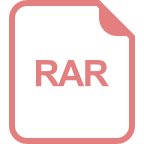
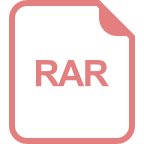
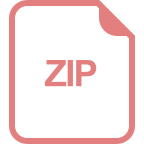
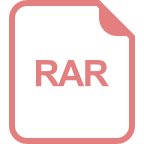
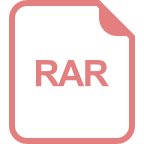










