Unity RegisterRawInputDevices
时间: 2024-04-12 09:30:15 浏览: 11
在Unity中,无法直接使用`RegisterRawInputDevices`函数来注册原始输入设备。但是,你可以通过使用Windows API和Unity的插件来实现。
以下是一个示例代码,展示如何在Unity中注册原始输入设备:
```csharp
using UnityEngine;
using System;
using System.Runtime.InteropServices;
public class RawInputRegistration : MonoBehaviour
{
[DllImport("user32.dll")]
private static extern bool RegisterRawInputDevices(ref RAWINPUTDEVICE pRawInputDevices, uint uiNumDevices, uint cbSize);
// Constants for input device types
private const ushort RIM_TYPEMOUSE = 0x0000;
private const ushort RIM_TYPEKEYBOARD = 0x0001;
private const ushort RIM_TYPEHID = 0x0002;
// Constants for raw input flags
private const uint RIDEV_INPUTSINK = 0x00000100;
private const uint RIDEV_NOLEGACY = 0x00000030;
// Structure for raw input device
[StructLayout(LayoutKind.Sequential)]
private struct RAWINPUTDEVICE
{
public ushort usUsagePage;
public ushort usUsage;
public uint dwFlags;
public IntPtr hwndTarget;
}
private void Start()
{
RegisterRawInputDevicesForMouse();
RegisterRawInputDevicesForKeyboard();
}
private void RegisterRawInputDevicesForMouse()
{
RAWINPUTDEVICE mouseDevice = new RAWINPUTDEVICE();
mouseDevice.usUsagePage = 0x01; // Generic desktop controls
mouseDevice.usUsage = 0x02; // Mouse
mouseDevice.dwFlags = RIDEV_INPUTSINK; // Receive input even when not in the foreground
mouseDevice.hwndTarget = IntPtr.Zero; // Null hwndTarget to receive input from all windows
if (!RegisterRawInputDevices(ref mouseDevice, 1, (uint)Marshal.SizeOf(mouseDevice)))
{
Debug.LogError("Failed to register raw input device for mouse.");
}
}
private void RegisterRawInputDevicesForKeyboard()
{
RAWINPUTDEVICE keyboardDevice = new RAWINPUTDEVICE();
keyboardDevice.usUsagePage = 0x01; // Generic desktop controls
keyboardDevice.usUsage = 0x06; // Keyboard
keyboardDevice.dwFlags = RIDEV_INPUTSINK | RIDEV_NOLEGACY; // Receive input even when not in the foreground, and disable legacy keyboard messages
keyboardDevice.hwndTarget = IntPtr.Zero; // Null hwndTarget to receive input from all windows
if (!RegisterRawInputDevices(ref keyboardDevice, 1, (uint)Marshal.SizeOf(keyboardDevice)))
{
Debug.LogError("Failed to register raw input device for keyboard.");
}
}
}
```
这段代码在Unity中注册了鼠标和键盘的原始输入设备。请注意,这个示例只是一个起点,你可能需要根据你的具体需求进行相应的修改和扩展。
重要提示:使用Windows API函数需要在Unity项目中启用`unsafe`代码,并且在构建项目时选择为Windows平台。此外,这些代码只能在Windows平台上运行,无法在其他平台上使用。
相关推荐
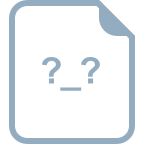
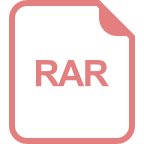
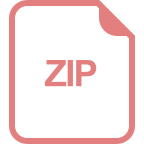








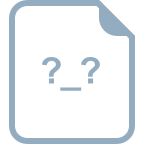
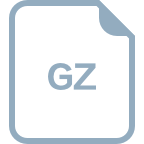