p = ( ) for number in range(3): x = int(input()) p.append(x) print(p)
时间: 2024-06-01 20:12:45 浏览: 95
This code prompts the user to enter three integers and stores them in a list called 'p'. Here's how it works:
1. The first line initializes an empty list called 'p'.
2. The 'for' loop runs three times, using the 'range' function to generate three integers (0, 1, and 2).
3. For each iteration of the loop, the code prompts the user to enter an integer using the 'input' function, and then converts the input to an integer using the 'int' function.
4. The integer is then appended to the list 'p' using the 'append' method.
5. Finally, the code prints the contents of the 'p' list using the 'print' function.
So, if the user entered the integers 4, 8, and 12, the output would be:
[4, 8, 12]
相关问题
list=[] while True: a=input("Enter a number(<Enter> to quit):") a=int(a) list.append(a) if a == ' ': break b=int(input("Enter a number searched:")) for i in range(len(list)): if b==list[i]: x=i else: x=-1 print("%d在列表索引值为%d的位置上"&(x,a))
这段代码存在几个问题:
1. 在判断用户输入是否为空时,应该用 `if not a` 的方式判断,而不是 `if a == ' '`。
2. 在判断列表中是否存在目标元素时,应该用 `if b == list[i]` 的方式判断,而不是 `if b==list[i]: x=i else: x=-1`。
3. 在输出结果时,应该用 `%d在列表索引值为%d的位置上` 的方式输出,而不是 `&(x,a)`。
以下是修正后的代码:
```
list = []
while True:
a = input("Enter a number(<Enter> to quit):")
if not a:
break
a = int(a)
list.append(a)
b = int(input("Enter a number searched:"))
x = -1
for i in range(len(list)):
if b == list[i]:
x = i
break
if x != -1:
print("%d在列表索引值为%d的位置上" % (b, x))
else:
print("%d不在列表中" % b)
```
麻烦改一下这个代码:n = int(input("Input n:"))arr = []print("Input the number")for i in range(n): arr.append(int(input()))target = int(input("Please input the number to find:"))found = Falsefor j in range(n): if arr[j] == target: found = True breakif found: print("Find it")else: print("Can't find it")
以下是改正过后的代码:
```
n = int(input("Input n:"))
arr = []
print("Input the number")
for i in range(n):
arr.append(int(input()))
target = int(input("Please input the number to find:"))
found = False
for j in range(n):
if arr[j] == target:
found = True
break
if found:
print("Find it")
else:
print("Can't find it")
```
主要的改动是:
- 在第一行代码中添加了冒号“:”,这是Python中语句块的标志。
- 在第二行代码中添加了空格。
- 在第三行代码中添加了冒号“:”。
- 在第六行代码中将“;”改为了冒号“:”。
- 在第七行代码中添加了“arr = ”,表示创建一个长度为n的数组。
- 在第九行代码中添加了冒号“:”。
- 在第十行代码中将“[i]”改为了“arr[i]”。
- 在第十二行代码中添加了冒号“:”。
- 在第十六行代码中将“ture”改为了“True”。
- 在第二十行代码中将“peintf”改为了“print”。
阅读全文
相关推荐
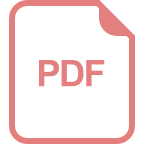
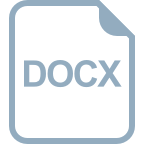
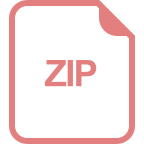














