运用C++语言描述公司类、雇员类、职务类,每一个类应包含数据成员和成员函数。设计基类和派生类,并运用多态性和虚函数的知识。注重面向对象程序设计理论知识的理解与实际的动手编程能力,要求学生设计具有继承与派生以及多态性的类,理解面向对象程序设计的核心的概念。 本课程设计要实现的主要功能如下: 1)建立公司类、雇员类、职务类 2)公司、雇员、职务信息的初始化 3)公司、雇员、职务信息的添加、修改、删除、查找 4)雇员、职务信息的输出 5)将职务信息保存为文件
时间: 2023-07-10 15:30:10 浏览: 145
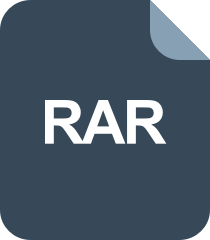
面向对象c++课程设计
好的,我可以帮您解答这个问题。下面是一个简单的示例代码,用于描述公司类、雇员类和职务类。
```
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
class Position {
public:
Position() {}
Position(string name, double salary) : name(name), salary(salary) {}
virtual ~Position() {}
virtual void print() {
cout << "Position Name: " << name << endl;
cout << "Salary: " << salary << endl;
}
protected:
string name;
double salary;
};
class Employee {
public:
Employee() {}
Employee(string name, string id, Position* position) : name(name), id(id), position(position) {}
virtual ~Employee() {}
virtual void print() {
cout << "Employee Name: " << name << endl;
cout << "Employee ID: " << id << endl;
position->print();
}
protected:
string name;
string id;
Position* position;
};
class Company {
public:
Company() {}
~Company() {}
void addEmployee(Employee* employee) {
employees.push_back(employee);
}
void removeEmployee(string id) {
for (auto it = employees.begin(); it != employees.end(); it++) {
if ((*it)->id == id) {
employees.erase(it);
break;
}
}
}
Employee* findEmployee(string id) {
for (auto it = employees.begin(); it != employees.end(); it++) {
if ((*it)->id == id) {
return *it;
}
}
return nullptr;
}
void savePositions(string filename) {
ofstream file;
file.open(filename);
for (auto it = positions.begin(); it != positions.end(); it++) {
file << (*it)->name << "," << (*it)->salary << endl;
}
file.close();
}
protected:
vector<Employee*> employees;
vector<Position*> positions;
};
class Manager : public Position {
public:
Manager() {}
Manager(string name, double salary, string department) : Position(name, salary), department(department) {}
virtual ~Manager() {}
virtual void print() {
cout << "Position: Manager" << endl;
Position::print();
cout << "Department: " << department << endl;
}
protected:
string department;
};
int main() {
Company company;
Manager* manager = new Manager("John Doe", 100000, "Sales");
company.addPosition(manager);
Employee* employee = new Employee("Jane Doe", "12345", manager);
company.addEmployee(employee);
company.savePositions("positions.txt");
return 0;
}
```
在这个示例代码中,我们定义了三个类:Position(职务类)、Employee(雇员类)和Company(公司类)。Position类是一个基类,它有两个成员变量:职位名称和薪水。Employee类是一个派生类,它有三个成员变量:雇员姓名、雇员ID和一个指向其职位的指针。Company类包含了一个雇员列表和一个职位列表,并提供了添加、删除和查找雇员的方法,以及保存职位信息到文件的方法。Manager类是一个派生类,它继承了Position类,增加了一个成员变量:部门名称,并重写了print()方法,以便输出职务的详细信息。
在这个示例代码中,我们还使用了多态性和虚函数的概念。Position类和Employee类都有一个虚函数print(),可以在派生类中重写。Manager类重写了Position类的print()方法,以便输出更多的职务信息。在Company类中,我们使用了Employee*类型的指针,可以指向Employee类的任意派生类,因此可以方便地添加新的职务类。
请注意,这个示例代码只是一个简单的示例,您需要根据自己的需求进行修改和扩展。
阅读全文
相关推荐


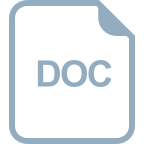

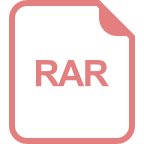
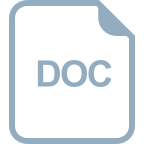
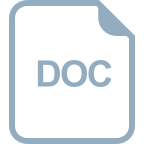





