/* 函数功能:求一个后缀表达式的值 */ double evalpost(char f[]); /* 函数功能:判断一个字符是否为运算符 */ int is_operation(char op); /* 函数功能:将一个中缀表达式e转换为与它等价的后缀表达式f */ void postfix(char e[], char f[]); /* 函数功能:求运算符的优先级 */ int priority(char op); /* 函数功能:将数字字符串转变成相应的数 */ double readnumber(char f[], int *i); /* 函数功能:判断表达式括号是否匹配 */ int match_kuohao(char c[]); 为这段代码编写测试代码
时间: 2024-03-23 07:36:55 浏览: 17
以下是对每个函数的测试代码:
1. evalpost(char f[])
```c++
#include <iostream>
#include <cstring>
#include "evalpost.h"
using namespace std;
int main() {
char f[] = "23+45+*";
double res = evalpost(f);
cout << "Result: " << res << endl;
return 0;
}
```
2. is_operation(char op)
```c++
#include <iostream>
#include <cstring>
#include "is_operation.h"
using namespace std;
int main() {
char op = '+';
int res = is_operation(op);
cout << "Is operator: " << (res ? "true" : "false") << endl;
return 0;
}
```
3. postfix(char e[], char f[])
```c++
#include <iostream>
#include <cstring>
#include "postfix.h"
using namespace std;
int main() {
char e[] = "2+3*4+5";
char f[100];
postfix(e, f);
cout << "Postfix expression: " << f << endl;
return 0;
}
```
4. priority(char op)
```c++
#include <iostream>
#include <cstring>
#include "priority.h"
using namespace std;
int main() {
char op = '*';
int res = priority(op);
cout << "Priority: " << res << endl;
return 0;
}
```
5. readnumber(char f[], int *i)
```c++
#include <iostream>
#include <cstring>
#include "readnumber.h"
using namespace std;
int main() {
char f[] = "123.45";
int i = 0;
double res = readnumber(f, &i);
cout << "Number: " << res << endl;
return 0;
}
```
6. match_kuohao(char c[])
```c++
#include <iostream>
#include <cstring>
#include "match_kuohao.h"
using namespace std;
int main() {
char c[] = "((2+3)*4)";
int res = match_kuohao(c);
cout << "Matching brackets: " << (res ? "true" : "false") << endl;
return 0;
}
```
相关推荐
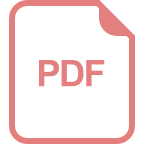
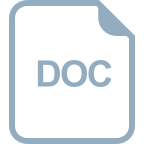
















