输入一个每个运算数都仅为一位数且只包含+ - * /运算符的后缀表达式,要求计算该表达式的值。使用c语言结合数据结构的知识用代码实现上述要求
时间: 2024-10-21 15:17:29 浏览: 50
在C语言中,我们可以使用栈来解析和计算后缀表达式,也称为逆波兰表示法(Reverse Polish Notation, RPN)。下面是使用栈的一个基本算法实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义操作数的数据类型,这里假设是整型
typedef int Operand;
// 操作符的优先级,用于栈操作
enum Priority {
PRIORITY_ADD = 1,
PRIORITY_SUB = 1,
PRIORITY_MUL = 2,
PRIORITY_DIV = 2,
PRIORITY_END
};
// 结构体代表操作数或运算符
struct Token {
char type;
union {
Operand value;
enum Priority priority;
} data;
};
// 判断字符是否是数字或运算符
int isDigit(char c) {
return (c >= '0' && c <= '9');
}
// 读取下一个有效token
struct Token nextToken(char* input) {
while(*input == ' ') input++; // 跳过空格
if(isDigit(*input)) {
struct Token token = { .type = '+', .data.value = *input++ - '0' };
while(isDigit(*input)) token.data.value = token.data.value * 10 + *input++ - '0';
return token;
}
switch(*input++) {
case '+':
return { .type = '+', .data.priority = PRIORITY_ADD };
case '-':
return { .type = '-', .data.priority = PRIORITY_SUB };
case '*':
return { .type = '*', .data.priority = PRIORITY_MUL };
case '/':
return { .type = '/', .data.priority = PRIORITY_DIV };
default:
printf("Invalid character %c\n", *input);
exit(1);
}
}
// 计算栈顶两个元素的运算结果并返回新值
Operand calculate(int top, int secondTop) {
if(top == secondTop || data[top].priority < data[secondTop].priority)
return data[top].value;
else if(data[top].priority > data[secondTop].priority)
return data[secondTop].value;
else if(data[top].type == '+')
return data[secondTop].value + data[top].value;
else if(data[top].type == '-')
return data[secondTop].value - data[top].value;
else if(data[top].type == '*')
return data[secondTop].value * data[top].value;
else if(data[top].type == '/') {
if(data[secondTop].value != 0)
return data[secondTop].value / data[top].value;
else
printf("Division by zero error\n");
exit(1);
}
}
// 主函数处理后缀表达式
double evaluatePostfix(char* postfixExp) {
stack struct Token s;
s.top = -1; // 初始化栈为空
for(char c : postfixExp) {
struct Token token = nextToken(&c);
switch(token.type) {
case '+': case '-':
while(s.top >= 0 && data[s.top].priority >= token.data.priority)
push(s, calculate(s.top, s.top - 1));
s.top++;
break;
case '*': case '/':
while(s.top >= 0 && (data[s.top].type == '*' || data[s.top].type == '/') && data[s.top].priority >= token.data.priority)
push(s, calculate(s.top, s.top - 1));
s.top++;
break;
default:
push(s, token); // 如果是数字,直接入栈
}
}
// 处理完所有元素,栈顶剩下的就是最终结果
while(s.top >= 0)
result += calculate(s.top, s.top - 1);
return (double)result;
}
int main() {
char postfixExp[] = "2 3 + 4 *"; // 示例后缀表达式
double result = evaluatePostfix(postfixExp);
printf("The result of the expression is: %.2f\n", result);
return 0;
}
```
这个代码片段展示了如何通过栈实现后缀表达式的求值。请注意,`push()` 和 `pop()` 函数在这里未给出,你需要自定义它们以便将token压入和弹出栈。同时,由于题目提到的单位数字和浮点数需求,这里将计算结果显示为浮点数。
阅读全文
相关推荐
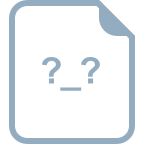
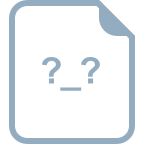
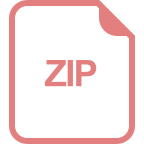


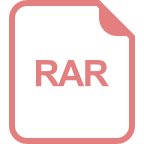
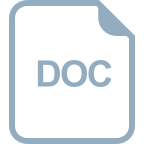




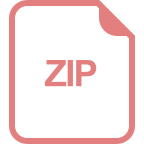
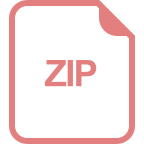
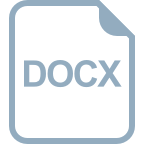





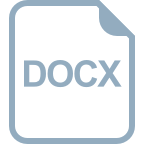