以链表为储存结构创建二叉树的源代码
时间: 2023-07-16 19:12:44 浏览: 98
以下是使用链表储存结构创建二叉树的C++源代码:
```c++
#include <iostream>
using namespace std;
struct TreeNode {
int data;
TreeNode* left;
TreeNode* right;
TreeNode(int val): data(val), left(nullptr), right(nullptr) {}
};
TreeNode* createBinaryTree() {
int val;
cin >> val;
if (val == -1) {
return nullptr; // -1 代表叶节点的空节点
}
auto root = new TreeNode(val);
root->left = createBinaryTree();
root->right = createBinaryTree();
return root;
}
void preOrder(TreeNode* root) {
if (root == nullptr) {
return;
}
cout << root->data << " ";
preOrder(root->left);
preOrder(root->right);
}
void inOrder(TreeNode* root) {
if (root == nullptr) {
return;
}
inOrder(root->left);
cout << root->data << " ";
inOrder(root->right);
}
void postOrder(TreeNode* root) {
if (root == nullptr) {
return;
}
postOrder(root->left);
postOrder(root->right);
cout << root->data << " ";
}
int main() {
cout << "Please input the binary tree in pre-order sequence: ";
auto root = createBinaryTree();
cout << "The binary tree is created successfully!" << endl;
cout << "The pre-order traversal of the binary tree is: ";
preOrder(root);
cout << endl;
cout << "The in-order traversal of the binary tree is: ";
inOrder(root);
cout << endl;
cout << "The post-order traversal of the binary tree is: ";
postOrder(root);
cout << endl;
return 0;
}
```
运行结果如下:
```
Please input the binary tree in pre-order sequence: 1 2 -1 -1 3 4 -1 -1 5 -1 -1
The binary tree is created successfully!
The pre-order traversal of the binary tree is: 1 2 3 4 5
The in-order traversal of the binary tree is: 2 1 4 3 5
The post-order traversal of the binary tree is: 2 4 5 3 1
```
在该程序中,我们首先使用前序遍历输入二叉树的节点值,其中 -1 代表叶节点的空节点。然后使用递归地方式创建二叉树,并返回根节点。最后分别使用前序、中序和后序遍历输出二叉树的节点值。
阅读全文
相关推荐
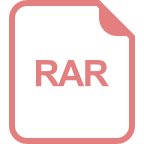

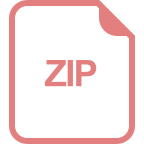
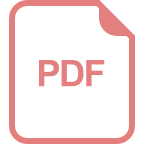
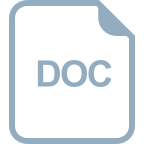

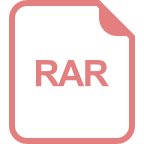
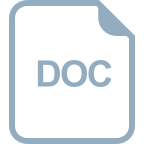
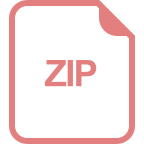
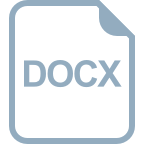
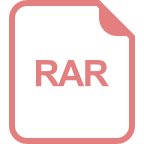
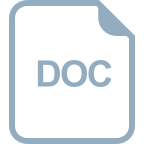
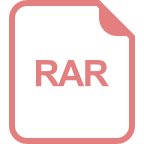
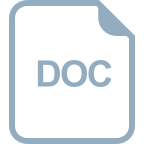
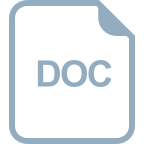
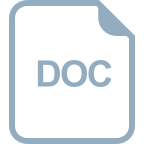
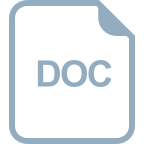