python编写函数,参数为一个字符串,返回这个字符串所有子串里面构成回文串的最大子串
时间: 2024-11-07 22:24:06 浏览: 36
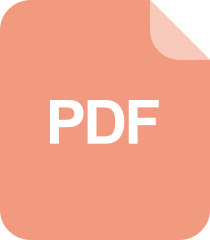
Python实现判断一个字符串是否包含子串的方法总结
在Python中,我们可以编写一个函数来找到一个字符串中最大的回文子串。这通常通过动态规划或中心扩散算法来实现。这里我将提供一个中心扩散法的基本思路:
```python
def longest_palindrome(s):
# 初始化长度为0的最长回文子串
max_length = 0
start = 0
# 对每个字符为中心向两边扩展
for i in range(len(s)):
# 检查奇数长度的回文
len1 = expand_around_center(s, i, i)
if len1 > max_length:
start = i - (len1 - 1) // 2
max_length = len1
# 检查偶数长度的回文
len2 = expand_around_center(s, i, i + 1)
if len2 > max_length:
start = i - len2 // 2
max_length = len2
return s[start:start + max_length]
# 辅助函数,检查以中心点为中心的左右两个指针是否构成回文
def expand_around_center(s, left, right):
while left >= 0 and right < len(s) and s[left] == s[right]:
left -= 1
right += 1
return right - left - 1
# 示例:
s = "babad"
print(longest_palindrome(s)) # 输出 "bab" 或 "aba"
```
这个函数会找出给定字符串中最长的回文子串。如果你想要进一步优化或理解细节,可以查看相关的数据结构和动态规划教程。
阅读全文
相关推荐
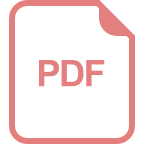
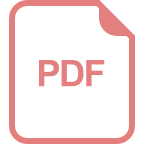















