C++thread类
时间: 2023-09-27 20:09:13 浏览: 89
C语言中没有内置的Thread类,但可以使用第三方库来实现多线程功能。常用的库包括pthread和Windows API。
对于Linux平台,可以使用pthread库来创建和管理线程。pthread库提供了一组函数来创建线程、同步线程、互斥访问等功能。以下是一个简单的示例代码:
```c
#include <stdio.h>
#include <pthread.h>
void* thread_func(void* arg) {
int thread_num = *(int*)arg;
printf("Hello from thread %d\n", thread_num);
return NULL;
}
int main() {
pthread_t thread1, thread2;
int num1 = 1, num2 = 2;
pthread_create(&thread1, NULL, thread_func, &num1);
pthread_create(&thread2, NULL, thread_func, &num2);
pthread_join(thread1, NULL);
pthread_join(thread2, NULL);
return 0;
}
```
在上面的例子中,我们使用了pthread_create函数来创建两个线程,并且传递了线程函数thread_func和对应的参数。每个线程函数打印了一个简单的消息,并通过pthread_join函数等待线程执行完毕。
对于Windows平台,可以使用Windows API中的CreateThread函数来创建线程。以下是一个简单的示例代码:
```c
#include <stdio.h>
#include <windows.h>
DWORD WINAPI thread_func(LPVOID arg) {
int thread_num = *(int*)arg;
printf("Hello from thread %d\n", thread_num);
return 0;
}
int main() {
HANDLE thread1, thread2;
int num1 = 1, num2 = 2;
thread1 = CreateThread(NULL, 0, thread_func, &num1, 0, NULL);
thread2 = CreateThread(NULL, 0, thread_func, &num2, 0, NULL);
WaitForSingleObject(thread1, INFINITE);
WaitForSingleObject(thread2, INFINITE);
CloseHandle(thread1);
CloseHandle(thread2);
return 0;
}
```
在上面的例子中,我们使用了CreateThread函数来创建两个线程,并且传递了线程函数thread_func和对应的参数。每个线程函数打印了一个简单的消息,并通过WaitForSingleObject函数等待线程执行完毕。
以上是使用pthread和Windows API来实现多线程的示例代码。注意,多线程编程需要谨慎处理共享资源的访问和同步,以避免出现竞态条件和死锁等问题。
阅读全文
相关推荐
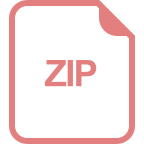
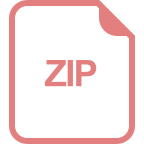
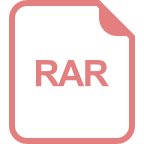
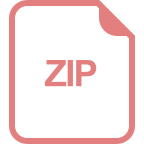
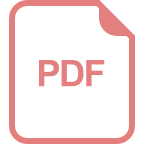
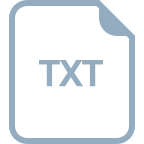
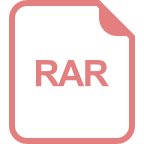
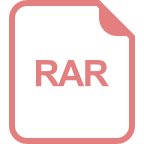
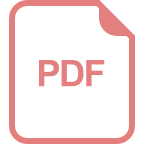
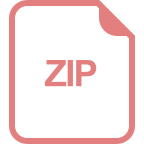
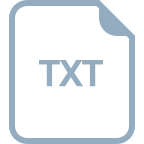
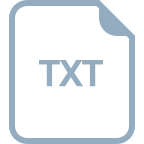
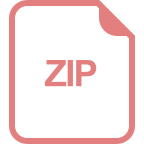
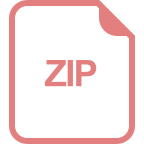
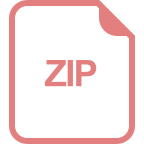
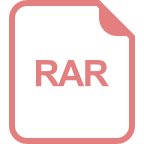