为以下代码提供一个数据集: from numpy import * # 创建不重复词的列表 ———— 词汇表 def createVocabList(dataSet): vocabSet = set([]) # 创建一个空集 for document in dataSet: vocabSet = vocabSet | set(document) # 创建两个集合的并集 return list(vocabSet)
时间: 2024-02-25 13:59:32 浏览: 101
这段代码是用于创建一个不重复词的列表,即词汇表,需要一个数据集作为输入。因此,可以为其提供一个包含多个文本的数据集,每个文本可以是一个字符串或者一个列表,其中每个元素表示一个词语。以下是一个示例数据集:
```
dataSet = [['hello', 'world', 'good', 'morning'],
['how', 'are', 'you', 'doing', 'today'],
['the', 'weather', 'is', 'great', 'today']]
```
该数据集包含三个文本,每个文本用一个列表来表示,列表中的每个元素都是一个词语。通过调用 `createVocabList(dataSet)` 函数,将返回一个列表,其中包含数据集中所有不重复的词语。
相关问题
import numpy as np import tensorflow_datasets as tfds import tensorflow as tf tfds.disable_progress_bar() import matplotlib.pyplot as plt def plot_graphs(history, metric): plt.plot(history.history[metric]) plt.plot(history.history['val_'+metric], '') plt.xlabel("Epochs") plt.ylabel(metric) plt.legend([metric, 'val_'+metric]) dataset, info = tfds.load('imdb_reviews', with_info=True, as_supervised=True) train_dataset, test_dataset = dataset['train'], dataset['test'] train_dataset.element_spec for example, label in train_dataset.take(1): print('text: ', example.numpy()) print('label: ', label.numpy()) BUFFER_SIZE = 10000 BATCH_SIZE = 64 train_dataset = train_dataset.shuffle(BUFFER_SIZE).batch(BATCH_SIZE).prefetch(tf.data.AUTOTUNE) test_dataset = test_dataset.batch(BATCH_SIZE).prefetch(tf.data.AUTOTUNE) for example, label in train_dataset.take(1): print('texts: ', example.numpy()[:3]) print() print('labels: ', label.numpy()[:3]) VOCAB_SIZE = 1000 encoder = tf.keras.layers.TextVectorization( max_tokens=VOCAB_SIZE) encoder.adapt(train_dataset.map(lambda text, label: text)) vocab = np.array(encoder.get_vocabulary()) vocab[:20] encoded_example = encoder(example)[:3].numpy() encoded_example for n in range(3): print("Original: ", example[n].numpy()) print("Round-trip: ", " ".join(vocab[encoded_example[n]])) print()解释一下这些代码。
这段代码是使用TensorFlow和TensorFlow Datasets加载IMDB电影评论数据集,然后对数据集进行预处理和向量化,以便进行情感分析的训练。下面是代码的详细解释:
1. 导入必要的库:
```
import numpy as np
import tensorflow_datasets as tfds
import tensorflow as tf
import matplotlib.pyplot as plt
```
2. 禁用进度条,以免在输出过程中出现过多的信息:
```
tfds.disable_progress_bar()
```
3. 加载IMDB评论数据集并获取有关数据集的信息:
```
dataset, info = tfds.load('imdb_reviews', with_info=True, as_supervised=True)
train_dataset, test_dataset = dataset['train'], dataset['test']
```
4. 查看数据集中的一个示例,并输出文本和标签:
```
for example, label in train_dataset.take(1):
print('text: ', example.numpy())
print('label: ', label.numpy())
```
5. 定义缓冲区大小和批次大小,并对训练和测试数据集进行批处理和预取:
```
BUFFER_SIZE = 10000
BATCH_SIZE = 64
train_dataset = train_dataset.shuffle(BUFFER_SIZE).batch(BATCH_SIZE).prefetch(tf.data.AUTOTUNE)
test_dataset = test_dataset.batch(BATCH_SIZE).prefetch(tf.data.AUTOTUNE)
```
6. 查看训练数据集中的前三个示例的文本和标签:
```
for example, label in train_dataset.take(1):
print('texts: ', example.numpy()[:3])
print()
print('labels: ', label.numpy()[:3])
```
7. 定义词汇表大小和文本向量化层,然后使用`adapt`方法对训练数据集进行适应:
```
VOCAB_SIZE = 1000
encoder = tf.keras.layers.TextVectorization(max_tokens=VOCAB_SIZE)
encoder.adapt(train_dataset.map(lambda text, label: text))
```
8. 获取词汇表并输出前20个词汇:
```
vocab = np.array(encoder.get_vocabulary())
vocab[:20]
```
9. 对一个示例进行编码,并输出编码结果:
```
encoded_example = encoder(example)[:3].numpy()
encoded_example
```
10. 对编码后的示例进行反向转换并输出结果:
```
for n in range(3):
print("Original: ", example[n].numpy())
print("Round-trip: ", " ".join(vocab[encoded_example[n]]))
print()
```
该代码段中的主要任务是将IMDB评论数据集加载到TensorFlow中,并准备进行情感分析训练。它包含了对数据的处理、向量化和预处理等步骤,是进行自然语言处理任务的常见流程。
本地词向量文件加载到情感分类模型中的pytorch代码
加载本地词向量文件到情感分类模型中的PyTorch代码可以按照以下步骤进行:
1. 导入必要的库
``` python
import torch
import torch.nn as nn
import numpy as np
```
2. 定义情感分类模型
``` python
class SentimentClassifier(nn.Module):
def __init__(self, input_dim, hidden_dim, output_dim, embeddings):
super(SentimentClassifier, self).__init__()
self.embedding_dim = embeddings.shape[1]
self.embedding = nn.Embedding(input_dim, self.embedding_dim)
self.embedding.weight.data.copy_(torch.from_numpy(embeddings))
self.embedding.weight.requires_grad = False
self.hidden_dim = hidden_dim
self.lstm = nn.LSTM(self.embedding_dim, hidden_dim, batch_first=True)
self.fc = nn.Linear(hidden_dim, output_dim)
def forward(self, text, text_lengths):
embedded = self.embedding(text)
packed_embedded = nn.utils.rnn.pack_padded_sequence(embedded, text_lengths.cpu(), batch_first=True)
packed_output, (hidden, cell) = self.lstm(packed_embedded)
output, output_lengths = nn.utils.rnn.pad_packed_sequence(packed_output, batch_first=True)
hidden = self.fc(torch.mean(output, dim=1))
return hidden
```
3. 加载本地词向量文件
``` python
def load_embeddings(embedding_file):
with open(embedding_file, 'r') as f:
embeddings = {}
for line in f:
values = line.strip().split()
word = values[0]
vector = np.asarray(values[1:], dtype='float32')
embeddings[word] = vector
return embeddings
```
4. 准备数据并创建模型实例
``` python
# 准备数据
vocab_size = len(vocab)
hidden_dim = 256
output_dim = 2
embeddings_file = 'word_embeddings.txt'
embeddings = load_embeddings(embeddings_file)
# 创建模型实例
model = SentimentClassifier(vocab_size, hidden_dim, output_dim, embeddings)
```
其中,`vocab` 是一个词汇表,可以使用 PyTorch 中的 `torchtext` 库来创建。`word_embeddings.txt` 是包含每个单词的向量表示的本地文件。
5. 训练和评估模型
使用 `torch.optim` 库中的优化器来训练模型,并使用 `torch.utils.data` 库中的数据加载器来加载数据。最后,使用测试集来评估模型的性能。
``` python
# 定义损失函数和优化器
criterion = nn.CrossEntropyLoss()
optimizer = torch.optim.Adam(model.parameters(), lr=0.001)
# 定义训练循环
def train(model, iterator, optimizer, criterion):
model.train()
for batch in iterator:
text, text_lengths = batch.text
optimizer.zero_grad()
predictions = model(text, text_lengths).squeeze(1)
loss = criterion(predictions, batch.label)
loss.backward()
optimizer.step()
# 定义评估函数
def evaluate(model, iterator, criterion):
model.eval()
total_loss = 0
total_correct = 0
with torch.no_grad():
for batch in iterator:
text, text_lengths = batch.text
predictions = model(text, text_lengths).squeeze(1)
loss = criterion(predictions, batch.label)
total_loss += loss.item()
total_correct += (predictions.argmax(1) == batch.label).sum().item()
return total_loss / len(iterator), total_correct / len(iterator.dataset)
# 训练模型
N_EPOCHS = 10
for epoch in range(N_EPOCHS):
train(model, train_iterator, optimizer, criterion)
train_loss, train_acc = evaluate(model, train_iterator, criterion)
test_loss, test_acc = evaluate(model, test_iterator, criterion)
print(f'Epoch: {epoch+1:02} | Train Loss: {train_loss:.3f} | Train Acc: {train_acc*100:.2f}% | Test Loss: {test_loss:.3f} | Test Acc: {test_acc*100:.2f}%')
```
阅读全文
相关推荐
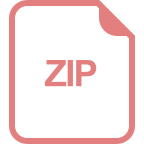
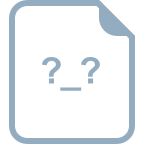
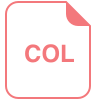
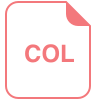
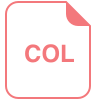
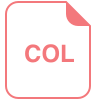
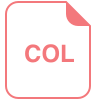
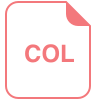
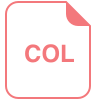
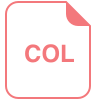
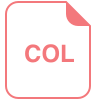
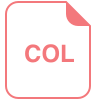
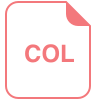
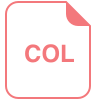
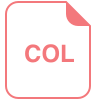
