基类为正方形类,数据包含边长,方法包含求面积、周长、构造 函数、输出函数;子类为正方体,保护继承于正方形类,数据包含高, 新增方法包含构造函数、求体积函数,在主函数中构建子类对象,并 将输出结果保存在文件中。
时间: 2024-02-23 20:00:50 浏览: 28
好的,以下是 C++ 代码实现:
```c++
#include <iostream>
#include <fstream>
using namespace std;
class Square {
protected:
double length;
public:
Square(double len) : length(len) {}
virtual double getArea() { return length * length; }
virtual double getPerimeter() { return 4 * length; }
virtual void printInfo() { cout << "正方形:边长=" << length << ",面积=" << getArea() << ",周长=" << getPerimeter() << endl; }
};
class Cube : protected Square {
private:
double height;
public:
Cube(double len, double h) : Square(len), height(h) {}
double getVolume() { return length * length * height; }
void printInfo() { cout << "正方体:边长=" << length << ",高=" << height << ",体积=" << getVolume() << endl; }
};
int main() {
Cube cube(5, 8);
cube.printInfo();
ofstream outfile("result.txt");
if (outfile.is_open()) {
outfile << "正方体:边长=" << cube.length << ",高=" << cube.height << ",体积=" << cube.getVolume() << endl;
outfile.close();
cout << "结果已保存在 result.txt 文件中。" << endl;
} else {
cerr << "无法打开文件!" << endl;
}
return 0;
}
```
正方形类 Square 有一个 protected 成员变量 length 表示边长,有求面积和周长的方法 getArea 和 getPerimeter,还有一个输出信息的方法 printInfo。
正方体类 Cube 继承自 Square,有一个新增的 protected 成员变量 height 表示高,有求体积的方法 getVolume,重载了 printInfo 方法,输出正方体的信息。
在主函数中构建了一个边长为 5,高为 8 的正方体对象 cube,调用其 printInfo 方法输出信息,并将结果保存在 result.txt 文件中。
相关推荐
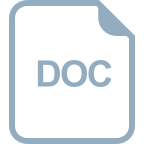
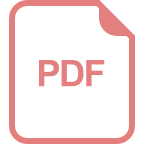














