用C++写一个RAS加密解密程序
时间: 2023-09-16 18:15:59 浏览: 86
好的,以下是一个简单的RAS加密解密程序的C++实现:
```
#include <iostream>
#include <openssl/rsa.h>
#include <openssl/pem.h>
#include <openssl/err.h>
using namespace std;
// 生成RSA密钥对
RSA* generateRSAKeyPair(int keyLength) {
RSA* rsa = NULL;
BIGNUM* bn = NULL;
FILE* fp = NULL;
int ret = 0;
// 创建RSA结构体
rsa = RSA_new();
if (rsa == NULL) {
return NULL;
}
// 生成大数
bn = BN_new();
ret = BN_set_word(bn, RSA_F4);
if (ret != 1) {
goto free_all;
}
// 生成RSA密钥对
ret = RSA_generate_key_ex(rsa, keyLength, bn, NULL);
if (ret != 1) {
goto free_all;
}
// 返回生成的RSA密钥对
return rsa;
free_all:
if (rsa != NULL) {
RSA_free(rsa);
}
if (bn != NULL) {
BN_free(bn);
}
if (fp != NULL) {
fclose(fp);
}
return NULL;
}
// RSA加密
int RSAEncrypt(const unsigned char* inData, int inLen, unsigned char* outData, RSA* rsa) {
int ret = 0;
// 获取RSA公钥
const BIGNUM* e = RSA_get0_e(rsa);
const BIGNUM* n = RSA_get0_n(rsa);
// 创建RSA公钥结构体
RSA* pubRSA = RSA_new();
ret = RSA_set0_key(pubRSA, const_cast<BIGNUM*>(n), const_cast<BIGNUM*>(e), NULL);
if (ret != 1) {
goto free_all;
}
// RSA加密
ret = RSA_public_encrypt(inLen, inData, outData, pubRSA, RSA_PKCS1_PADDING);
if (ret < 0) {
goto free_all;
}
// 释放RSA公钥结构体
RSA_free(pubRSA);
// 返回加密数据长度
return ret;
free_all:
if (pubRSA != NULL) {
RSA_free(pubRSA);
}
return -1;
}
// RSA解密
int RSADecrypt(const unsigned char* inData, int inLen, unsigned char* outData, RSA* rsa) {
int ret = 0;
// 获取RSA私钥
const BIGNUM* d = RSA_get0_d(rsa);
const BIGNUM* n = RSA_get0_n(rsa);
// 创建RSA私钥结构体
RSA* priRSA = RSA_new();
ret = RSA_set0_key(priRSA, const_cast<BIGNUM*>(n), const_cast<BIGNUM*>(d), NULL);
if (ret != 1) {
goto free_all;
}
// RSA解密
ret = RSA_private_decrypt(inLen, inData, outData, priRSA, RSA_PKCS1_PADDING);
if (ret < 0) {
goto free_all;
}
// 释放RSA私钥结构体
RSA_free(priRSA);
// 返回解密数据长度
return ret;
free_all:
if (priRSA != NULL) {
RSA_free(priRSA);
}
return -1;
}
int main() {
RSA* rsa = NULL;
unsigned char inData[1024] = "Hello, World!";
unsigned char outData[1024] = {0};
int outLen = 0;
// 生成RSA密钥对
rsa = generateRSAKeyPair(1024);
if (rsa == NULL) {
cout << "Generate RSA key pair failed!" << endl;
return -1;
}
// RSA加密
outLen = RSAEncrypt(inData, strlen((const char*)inData), outData, rsa);
if (outLen < 0) {
cout << "RSA encrypt failed!" << endl;
return -1;
}
// RSA解密
outLen = RSADecrypt(outData, outLen, inData, rsa);
if (outLen < 0) {
cout << "RSA decrypt failed!" << endl;
return -1;
}
// 输出解密结果
cout << "RSA decrypt result: " << inData << endl;
// 释放RSA密钥对
RSA_free(rsa);
return 0;
}
```
这个程序使用了OpenSSL库来实现RAS加密解密。在程序中,先使用`generateRSAKeyPair`函数生成RSA密钥对,然后使用`RSAEncrypt`函数来加密数据,使用`RSADecrypt`函数来解密数据。程序最终输出解密结果。
阅读全文
相关推荐

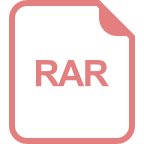
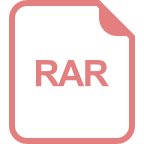
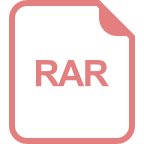
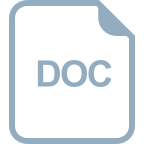
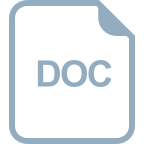
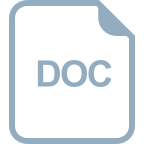
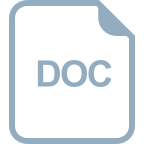
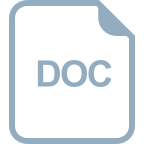
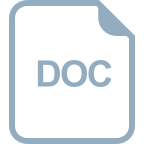
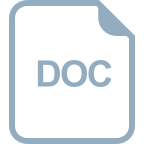




