输出二叉树先序遍历结果的第k个结点
时间: 2023-11-26 11:59:17 浏览: 101
假设二叉树的先序遍历结果为 preOrderList,则该问题可以通过以下步骤解决:
1. 定义一个计数器 count 和一个指针 index,初始值分别为 0 和 0。
2. 从 preOrderList 中依次取出每个结点值进行判断:
- 如果 count 的值等于 k,返回当前结点的值。
- 如果 count 的值小于 k,将 count 的值加 1。
3. 如果遍历结束还没有找到第 k 个结点,则返回空。
以下是一个 Python 实现的代码示例:
```python
class TreeNode:
def __init__(self, val):
self.val = val
self.left = None
self.right = None
def kth_node_of_pre_order_traversal(root: TreeNode, k: int) -> int:
if not root or k <= 0:
return None
pre_order_list = []
stack = [root]
while stack:
node = stack.pop()
pre_order_list.append(node.val)
if node.right:
stack.append(node.right)
if node.left:
stack.append(node.left)
count = 0
index = 0
while index < len(pre_order_list):
if count == k:
return pre_order_list[index]
if count < k:
count += 1
index += 1
return None
```
其中,类 TreeNode 定义了二叉树的结构,函数 kth_node_of_pre_order_traversal 实现了输出二叉树先序遍历结果的第 k 个结点的功能。
阅读全文
相关推荐
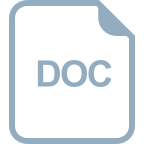
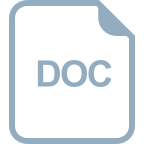
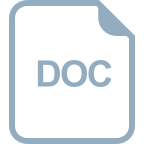















