读出一幅灰度图像("lena_gray.gif"),给这幅图像分别加入椒盐噪声和高斯噪声后并与前一张图显示在同一图像窗口中
时间: 2023-06-06 13:08:52 浏览: 145
好的,以下是使用Python代码实现你的要求:
```
import cv2
import numpy as np
# 读入灰度图像lena_gray.gif
img = cv2.imread('lena_gray.gif', cv2.IMREAD_GRAYSCALE)
# 添加椒盐噪声
pepper_salt_noise = np.copy(img)
pepper_salt_noise[img < 30] = 0
pepper_salt_noise[img > 225] = 255
pepper_salt_noise = np.uint8(pepper_salt_noise)
# 添加高斯噪声
gaussian_noise = np.copy(img)
cv2.randn(gaussian_noise, 0, 10)
gaussian_noise = cv2.add(gaussian_noise, img)
gaussian_noise = np.uint8(gaussian_noise)
# 在同一图像窗口中显示三张图片
cv2.imshow('lena_gray.gif, pepper_salt_noise, gaussian_noise', np.hstack([img, pepper_salt_noise, gaussian_noise]))
cv2.waitKey()
cv2.destroyAllWindows()
```
注意:此处只是提供代码,可能无法对图像进行路径的读取,请修改代码中的文件路径以适应你的环境。
相关问题
python编写代码读出一幅灰度图像("lena_gray.gif"),采用 3×3 拉普拉斯算子 𝑤=[1,1,1;1,−8,1;1,1,1] 对其进行滤波
可以这样编写代码:
```python
from PIL import Image
import numpy as np
# 读入图像
im = Image.open('lena_gray.gif')
im_arr = np.array(im)
# 定义拉普拉斯算子
w = np.array([[1, 1, 1],
[1, -8, 1],
[1, 1, 1]])
# 图像滤波
filtered = np.zeros_like(im_arr)
for i in range(1, im_arr.shape[0] - 1):
for j in range(1, im_arr.shape[1] - 1):
filtered[i][j] = np.sum(w * im_arr[i-1:i+2, j-1:j+2])
if filtered[i][j] < 0:
filtered[i][j] = 0
elif filtered[i][j] > 255:
filtered[i][j] = 255
# 显示滤波后的图像
Image.fromarray(filtered.astype(np.uint8)).show()
```
这段代码会读入lena_gray.gif图像,并采用3x3拉普拉斯算子对其进行滤波,滤波后的图像会显示在屏幕上。
% 读入灰度图像 gray_img = imread('lena_gray.tif'); % 将图像转换为伪彩色图像 color_img = ind2rgb(gray_img, jet(256)); % 显示图像 imshow(color_img);
这段代码的作用是将灰度图像转换为伪彩色图像,并使用jet调色板对其进行着色。然后将结果图像显示出来。具体来说:
- imread('lena_gray.tif') 读入了名为lena_gray.tif的灰度图像,将其存储在gray_img中。
- jet(256) 生成一个256色的调色板。
- ind2rgb(gray_img, jet(256)) 将灰度图像gray_img转换为伪彩色图像,使用jet调色板着色,结果存储在color_img中。
- imshow(color_img) 显示结果图像。
阅读全文
相关推荐



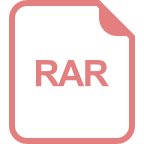
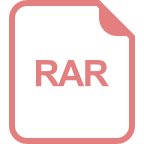







