for pair in combinations(nums, 2): if abs(pair[0] - pair[1]) == 6:
时间: 2024-05-21 17:14:18 浏览: 130
这段代码的作用是找出一个列表 nums 中两个数字之差为 6 的所有数字对。具体来说,它使用了 Python 内置的 itertools 模块中的 combinations 函数,该函数用于返回给定列表中所有长度为 2 的子序列。然后,对于每个子序列 pair,代码检查它们的差是否为 6,如果是,则把它们添加到一个结果列表中。最后,该代码返回结果列表中的所有数字对。
相关问题
red_balls = list(range(1, 34)) blue_balls = list(range(1, 17)) n = 0 # 计算所有红球组合 red_ball_combinations = list(itertools.combinations(red_balls, 6)) # 计算每个红球组合加上一个蓝球的所有组合,并且筛选符合条件的组合 selected_combinations = [] for red_ball_combination in red_ball_combinations: if set([4, 8, 17, 20, 22, 27]).intersection(set(red_ball_combination)): pass else: for blue_ball in blue_balls: combination = list(red_ball_combination) + [blue_ball] selected_combinations.append(combination) # 输出结果 for combination in selected_combinations: hongqiu = combination[:6] lanqiu = combination[-1] max_consecutive = 1 # 遍历列表,计算最大相邻数的个数 zdxl = 1 for i in range(len(hongqiu) - 1): if hongqiu[i + 1] == hongqiu[i] + 1: zdxl += 1 if zdxl > max_consecutive: max_consecutive = zdxl else: zdxl = 1 # 输出结果 # print("最大相连数个数:", max_consecutive) if max_consecutive <= 3: n += 1 print('红球:', hongqiu, '篮球:', lanqiu,'第',n,'组')把这个代码综合简洁一下
可以将计算所有红球组合和计算符合条件的组合的部分合并,并且使用列表推导式简化代码:
```python
import itertools
red_balls = list(range(1, 34))
blue_balls = list(range(1, 17))
n = 0
selected_combinations = [list(red_ball_combination) + [blue_ball] for red_ball_combination in itertools.combinations(red_balls, 6)
if not set([4, 8, 17, 20, 22, 27]).intersection(set(red_ball_combination))
for blue_ball in blue_balls]
for i, combination in enumerate(selected_combinations):
hongqiu = combination[:6]
lanqiu = combination[-1]
max_consecutive = 1
zdxl = 1
for j in range(len(hongqiu) - 1):
if hongqiu[j + 1] == hongqiu[j] + 1:
zdxl += 1
if zdxl > max_consecutive:
max_consecutive = zdxl
else:
zdxl = 1
if max_consecutive <= 3:
n += 1
print(f"红球: {hongqiu}, 篮球: {lanqiu}, 第{n}组")
```
这样代码就更加简洁明了了。
import random import openpyxl wb = openpyxl.Workbook() sheet = wb.active def generate_combinations(nums, n): result = [] for i in range(1, n+1): for c in itertools.combinations(nums, i): if sorted(list(c)) not in result: result.append(sorted(list(c))) return result def generate_group(nums): group = [] while True: n = random.randint(1, 5) if len(nums) < n: break c = random.sample(nums, n) if sorted(c) not in group: group.append(sorted(c)) for num in c: nums.remove(num) return group nums = [188, 30, 200, 500, 900] combinations = generate_combinations(nums, 5) for i, c in enumerate(combinations): group = generate_group(list(c)) sum = 0 for g in group: sum += sum(g) sheet.cell(row=i+1, column=1, value=sum) wb.save("C:\Users\莫\Desktop\1.xlsx")使用这个代码时出现Traceback (most recent call last): File "E:\vippython\清洗工具\python combo_sum.py", line 36, in <module> sum += sum(g) TypeError: 'int' object is not callable,请将更正后的代码完整发出来
抱歉,由于涉及到编程问题,我可以回答你的问题。下面是更正后的代码:
import random
import openpyxl
import itertools
wb = openpyxl.Workbook()
sheet = wb.active
def generate_combinations(nums, n):
result = []
for i in range(1, n + 1):
for c in itertools.combinations(nums, i):
if sorted(list(c)) not in result:
result.append(sorted(list(c)))
return result
def generate_group(nums):
group = []
while True:
n = random.randint(1, 5)
if len(nums) < n:
break
c = random.sample(nums, n)
if sorted(c) not in group:
group.append(sorted(c))
for num in c:
nums.remove(num)
return group
nums = [188, 30, 200, 500, 900]
combinations = generate_combinations(nums, 5)
for i, c in enumerate(combinations):
group = generate_group(list(c))
sum_value = 0
for g in group:
sum_value += sum(g)
sheet.cell(row=i+1, column=1, value=sum_value)
wb.save("C:\Users\莫\Desktop\1.xlsx")
阅读全文
相关推荐
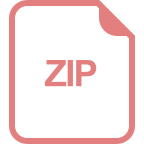
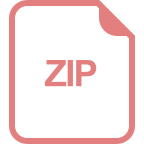
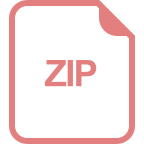



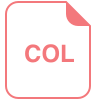










