用clojure写一个德州扑克概率计算器
时间: 2023-11-18 09:05:23 浏览: 214
好的,以下是一个用Clojure实现的德州扑克概率计算器的示例代码:
```clojure
(def suits ["H" "D" "C" "S"])
(def ranks ["2" "3" "4" "5" "6" "7" "8" "9" "T" "J" "Q" "K" "A"])
(defn new-deck []
(for [suit suits
rank ranks]
(str rank suit)))
(defn shuffle-deck [deck]
(shuffle deck))
(defn deal-hand [deck]
(take 2 deck))
(defn deal-board [deck]
(take 5 (drop 2 deck)))
(defn sort-hand [hand]
(sort-by #(index-of ranks %) hand))
(defn hand-straight? [hand]
(let [sorted-hand (sort-hand hand)
straight-ranks (take 5 (iterate inc (index-of ranks (first sorted-hand))))]
(= (map #(index-of ranks %) sorted-hand) straight-ranks)))
(defn hand-flush? [hand]
(let [suit-counts (frequencies (map last hand))]
(some #(>= % 5) suit-counts)))
(defn hand-straight-flush? [hand]
(and (hand-straight? hand) (hand-flush? hand)))
(defn hand-four-of-a-kind? [hand]
(let [rank-counts (frequencies (map first hand))]
(some #(= % 4) rank-counts)))
(defn hand-full-house? [hand]
(let [rank-counts (frequencies (map first hand))]
(and (some #(= % 3) rank-counts) (some #(= % 2) rank-counts))))
(defn hand-three-of-a-kind? [hand]
(let [rank-counts (frequencies (map first hand))]
(some #(= % 3) rank-counts)))
(defn hand-two-pairs? [hand]
(let [rank-counts (frequencies (map first hand))]
(= (count (filter #(= % 2) rank-counts)) 2)))
(defn hand-one-pair? [hand]
(let [rank-counts (frequencies (map first hand))]
(some #(= % 2) rank-counts)))
(defn hand-high-card [hand]
(last (sort-hand hand)))
(defn hand-type [hand]
(cond-> :high-card
(hand-straight-flush? hand) (constantly :straight-flush)
(hand-four-of-a-kind? hand) (constantly :four-of-a-kind)
(hand-full-house? hand) (constantly :full-house)
(hand-flush? hand) (constantly :flush)
(hand-straight? hand) (constantly :straight)
(hand-three-of-a-kind? hand) (constantly :three-of-a-kind)
(hand-two-pairs? hand) (constantly :two-pairs)
(hand-one-pair? hand) (constantly :one-pair)))
(defn simulate [n]
(let [deck (shuffle-deck (new-deck))
hands (for [i (range n)]
(let [hand (deal-hand deck)
board (deal-board deck)
cards (concat hand board)]
(hand-type cards)))]
(frequencies hands)))
(simulate 100000)
```
这个程序定义了一些基本函数,比如生成一副牌、洗牌、发牌、牌型判断等,最后通过模拟大量牌局来计算每种牌型的概率。
您可以在此基础上进行修改和扩展,比如增加计算胜率的功能等。
阅读全文
相关推荐















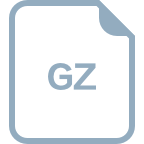


