写一段代码,在OpenGl上实现基于种子区域填充算法,并调用该算法对绘制图形的指定区域进行填充。
时间: 2023-11-22 19:52:24 浏览: 112
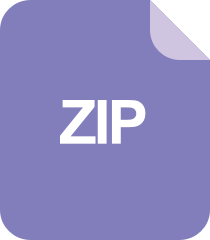
种子填充算法
下面是一个简单的基于种子区域填充算法的OpenGL代码示例:
```c++
#include <GL/glut.h>
// 定义种子颜色
float seedColor[3] = {1.0f, 0.0f, 0.0f};
void seedFill(int x, int y, float* fillColor, float* seedColor)
{
// 获取当前像素的颜色
float currentColor[3];
glReadPixels(x, y, 1, 1, GL_RGB, GL_FLOAT, currentColor);
// 如果当前像素的颜色等于种子颜色,则填充当前像素
if (currentColor[0] == seedColor[0] && currentColor[1] == seedColor[1] && currentColor[2] == seedColor[2])
{
glColor3fv(fillColor);
glBegin(GL_POINTS);
glVertex2i(x, y);
glEnd();
// 递归填充当前像素的上下左右四个相邻像素
seedFill(x+1, y, fillColor, seedColor);
seedFill(x-1, y, fillColor, seedColor);
seedFill(x, y+1, fillColor, seedColor);
seedFill(x, y-1, fillColor, seedColor);
}
}
void display()
{
glClear(GL_COLOR_BUFFER_BIT);
// 绘制一个矩形作为需要填充的区域
glColor3f(0.0f, 0.0f, 0.0f);
glBegin(GL_LINE_LOOP);
glVertex2i(100, 100);
glVertex2i(200, 100);
glVertex2i(200, 200);
glVertex2i(100, 200);
glEnd();
// 在矩形中心点设置一个种子点,并调用种子填充函数进行填充
int seedX = 150;
int seedY = 150;
seedFill(seedX, seedY, fillColor, seedColor);
glFlush();
}
int main(int argc, char** argv)
{
glutInit(&argc, argv);
glutInitDisplayMode(GLUT_SINGLE | GLUT_RGB);
glutInitWindowSize(400, 400);
glutCreateWindow("Seed Fill Demo");
glClearColor(1.0, 1.0, 1.0, 0.0);
gluOrtho2D(0, 400, 0, 400);
glutDisplayFunc(display);
glutMainLoop();
return 0;
}
```
在该代码示例中,我们定义了一个 `seedFill` 函数用于填充指定区域。该函数使用递归的方式依次填充当前像素的上下左右四个相邻像素,并判断当前像素的颜色是否等于种子颜色,如果相等,则将当前像素填充为指定颜色。在 `display` 函数中,我们绘制了一个矩形作为需要填充的区域,并在矩形中心点设置一个种子点,调用 `seedFill` 函数进行填充。最终,我们使用 `glFlush` 函数将填充后的图形显示在屏幕上。
阅读全文
相关推荐





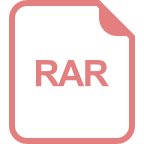
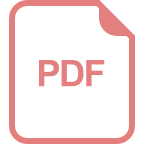
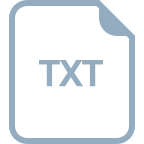
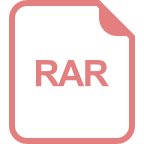
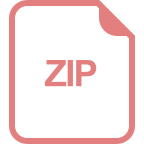
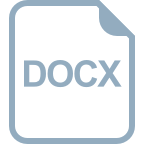
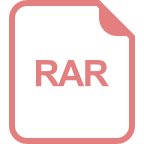
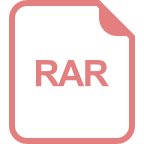
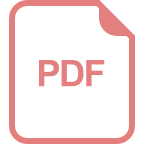
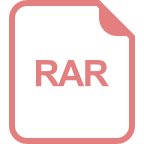
