在一个数组中出现次数最多的数称为众数。用分治法设计求解众数问题的算法,并分析时间复杂度 写出C++伪代码
时间: 2024-04-29 15:21:08 浏览: 166
分治法解决众数问题的算法思路如下:
1. 将数组分成两部分,分别递归求解每一部分的众数;
2. 如果两部分的众数相同,则返回该众数;
3. 如果两部分的众数不同,则需要分别计算两部分众数出现的次数,返回出现次数较多的众数。
时间复杂度分析:
设递归深度为h,则每层的时间复杂度为O(n),因为需要遍历数组寻找众数。总的时间复杂度为O(nlogn)。
C语言实现:
```c
#include <stdio.h>
int majorityElement(int* nums, int numsSize) {
if (numsSize == 1) {
return nums[0];
}
int mid = numsSize / 2;
int left = majorityElement(nums, mid);
int right = majorityElement(nums+mid, numsSize-mid);
if (left == right) {
return left;
}
int left_count = 0, right_count = 0;
for (int i = 0; i < numsSize; i++) {
if (nums[i] == left) {
left_count++;
} else if (nums[i] == right) {
right_count++;
}
}
return (left_count > right_count) ? left : right;
}
int main() {
int nums[] = {1, 2, 2, 2, 3, 4, 2};
int numsSize = sizeof(nums) / sizeof(nums[0]);
int result = majorityElement(nums, numsSize);
printf("The majority element is %d\n", result);
return 0;
}
```
伪代码实现:
```
function majorityElement(nums, l, r)
if l == r then
return nums[l]
end if
mid = (l+r) / 2
left = majorityElement(nums, l, mid)
right = majorityElement(nums, mid+1, r)
if left == right then
return left
end if
left_count = 0, right_count = 0
for i = l to r do
if nums[i] == left then
left_count = left_count + 1
else if nums[i] == right then
right_count = right_count + 1
end if
end for
if left_count > right_count then
return left
else
return right
end if
end function
nums = [1, 2, 2, 2, 3, 4, 2]
result = majorityElement(nums, 0, nums.length-1)
print("The majority element is " + result)
```
阅读全文
相关推荐




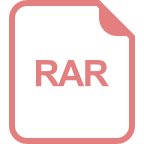










