python的tkinter的俄罗斯方块
时间: 2023-10-19 11:26:06 浏览: 52
以下是一个简单的Python Tkinter俄罗斯方块游戏的代码示例:
```python
import tkinter as tk
import random
# 定义方块的形状
shapes = [
[[1, 1, 1], [0, 1, 0]],
[[0, 2, 2], [2, 2, 0]],
[[3, 3, 0], [0, 3, 3]],
[[4, 0, 0], [4, 4, 4]],
[[0, 0, 5], [5, 5, 5]],
[[6, 6, 6, 6]],
[[7, 7], [7, 7]]
]
# 定义每个方块的颜色
colors = [
"cyan", "yellow", "purple", "green", "red", "orange", "blue"
]
class Tetris(tk.Frame):
def __init__(self, master):
super().__init__(master)
self.master = master
self.canvas = tk.Canvas(self, width=200, height=400, borderwidth=0, highlightthickness=0)
self.canvas.pack(side="left")
self.score_label = tk.Label(self, text="Score: 0", font=("Helvetica", 16))
self.score_label.pack(side="top", pady=10)
self.grid = [[0 for y in range(20)] for x in range(10)]
self.current_shape = None
self.current_x = 0
self.current_y = 0
self.score = 0
self.timer_id = None
self.spawn_shape()
self.bind_all("<Key>", self.key_pressed)
self.pack()
def spawn_shape(self):
shape = random.choice(shapes)
self.current_shape = shape
self.current_x = 4
self.current_y = 0
self.draw_shape()
def draw_shape(self):
self.canvas.delete("all")
for y, row in enumerate(self.current_shape):
for x, color in enumerate(row):
if color > 0:
self.canvas.create_rectangle(
x * 20 + self.current_x * 20,
y * 20 + self.current_y * 20,
x * 20 + self.current_x * 20 + 20,
y * 20 + self.current_y * 20 + 20,
fill=colors[color - 1],
outline="white"
)
def key_pressed(self, event):
if event.keysym == "Left":
self.current_x -= 1
if self.check_collision():
self.current_x += 1
elif event.keysym == "Right":
self.current_x += 1
if self.check_collision():
self.current_x -= 1
elif event.keysym == "Down":
self.current_y += 1
if self.check_collision():
self.current_y -= 1
self.lock_shape()
elif event.keysym == "Up":
self.rotate_shape()
self.draw_shape()
def check_collision(self):
for y, row in enumerate(self.current_shape):
for x, color in enumerate(row):
if color > 0:
if (
x + self.current_x < 0 or
x + self.current_x > 9 or
y + self.current_y > 19 or
self.grid[x + self.current_x][y + self.current_y] > 0
):
return True
return False
def lock_shape(self):
for y, row in enumerate(self.current_shape):
for x, color in enumerate(row):
if color > 0:
self.grid[x + self.current_x][y + self.current_y] = color
self.check_lines()
self.spawn_shape()
def check_lines(self):
lines_cleared = 0
for y, row in enumerate(self.grid):
if all(row):
lines_cleared += 1
for x in range(len(row)):
row[x] = 0
for y2 in range(y, 0, -1):
for x2 in range(len(row)):
self.grid[x2][y2] = self.grid[x2][y2 - 1]
if lines_cleared > 0:
self.score += lines_cleared * 100
self.score_label.configure(text="Score: {}".format(self.score))
if self.check_collision():
self.game_over()
def rotate_shape(self):
new_shape = []
for x in range(len(self.current_shape[0])):
new_row = []
for y in range(len(self.current_shape)):
new_row.append(self.current_shape[len(self.current_shape) - y - 1][x])
new_shape.append(new_row)
self.current_shape = new_shape
if self.check_collision():
self.current_shape = self.rotate_shape_back(self.current_shape)
def rotate_shape_back(self, shape):
new_shape = []
for x in range(len(shape[0])):
new_row = []
for y in range(len(shape)):
new_row.append(shape[y][len(shape[0]) - x - 1])
new_shape.append(new_row)
return new_shape
def game_over(self):
self.canvas.create_text(
100, 200,
text="Game Over",
font=("Helvetica", 32),
fill="white"
)
self.after_cancel(self.timer_id)
def start(self):
self.timer_id = self.after(1000, self.run_game)
def run_game(self):
self.current_y += 1
if self.check_collision():
self.current_y -= 1
self.lock_shape()
self.draw_shape()
self.timer_id = self.after(1000, self.run_game)
root = tk.Tk()
root.title("Tetris")
game = Tetris(root)
game.start()
root.mainloop()
```
这个示例代码实现了一个简单的俄罗斯方块游戏,可以在Tkinter窗口中玩。
相关推荐
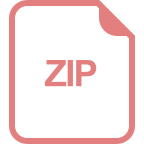
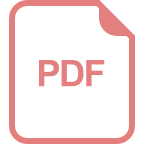
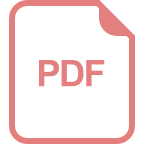














