灰狼优化算法python代码
时间: 2023-09-08 21:14:13 浏览: 77
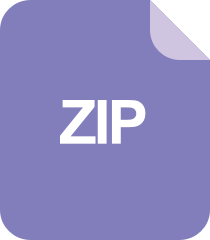
Python,灰狼优化算法

以下是灰狼优化算法的Python代码:
```python
import numpy as np
def obj_func(x):
# 定义目标函数
return sum(x**2)
class GreyWolfOptimizer:
def __init__(self, obj_func, dim, max_iter, lb, ub, pa=0.2, a=2):
self.obj_func = obj_func # 目标函数
self.dim = dim # 待优化变量个数
self.max_iter = max_iter # 最大迭代次数
self.lb = lb # 每个变量的下界
self.ub = ub # 每个变量的上界
self.pa = pa # 猎物逃跑概率
self.a = a # 搜索参数
self.alpha = np.zeros(self.dim) # 灰狼的位置向量
self.beta = np.zeros(self.dim) # 灰狼的位置向量
self.delta = np.zeros(self.dim) # 灰狼的位置向量
self.positions = np.zeros((self.dim, 3)) # 存储灰狼的位置向量
self.fitness = np.zeros(3) # 存储灰狼的适应度
def optimize(self):
# 初始化灰狼位置
for i in range(self.dim):
self.alpha[i] = self.lb[i] + (self.ub[i] - self.lb[i]) * np.random.rand()
self.beta[i] = self.lb[i] + (self.ub[i] - self.lb[i]) * np.random.rand()
self.delta[i] = self.lb[i] + (self.ub[i] - self.lb[i]) * np.random.rand()
# 迭代
for t in range(self.max_iter):
# 计算每个灰狼的适应度
self.fitness[0] = self.obj_func(self.alpha)
self.fitness[1] = self.obj_func(self.beta)
self.fitness[2] = self.obj_func(self.delta)
# 找到当前最优解
best_index = np.argmin(self.fitness)
best_fitness = self.fitness[best_index]
best_position = self.positions[:, best_index]
# 更新灰狼位置
for i in range(self.dim):
r1 = np.random.rand()
r2 = np.random.rand()
A1 = 2 * self.a * r1 - self.a
C1 = 2 * r2
D_alpha = abs(C1 * self.alpha[i] - self.positions[i, 0])
X1 = self.alpha[i] - A1 * D_alpha
r1 = np.random.rand()
r2 = np.random.rand()
A2 = 2 * self.a * r1 - self.a
C2 = 2 * r2
D_beta = abs(C2 * self.beta[i] - self.positions[i, 1])
X2 = self.beta[i] - A2 * D_beta
r1 = np.random.rand()
r2 = np.random.rand()
A3 = 2 * self.a * r1 - self.a
C3 = 2 * r2
D_delta = abs(C3 * self.delta[i] - self.positions[i, 2])
X3 = self.delta[i] - A3 * D_delta
self.positions[i, 0] = np.clip(X1, self.lb[i], self.ub[i])
self.positions[i, 1] = np.clip(X2, self.lb[i], self.ub[i])
self.positions[i, 2] = np.clip(X3, self.lb[i], self.ub[i])
# 猎物逃跑
for i in range(3):
if np.random.rand() < self.pa:
j_rand = np.random.randint(3)
while j_rand == i:
j_rand = np.random.randint(3)
D = np.abs(self.positions[:, i] - self.positions[:, j_rand])
r = np.random.rand()
self.positions[:, i] = best_position - r * D
return best_fitness, best_position
```
使用方法,可以先定义`obj_func`为自己的目标函数,然后设置灰狼优化器的各种参数,最后调用`optimize`方法即可得到最优解和最优解对应的参数值。
阅读全文
相关推荐
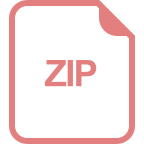





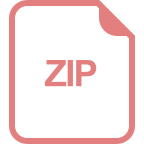








