请帮我讲解一下人狼羊菜过河问题的代码
时间: 2023-12-03 10:44:17 浏览: 112
人狼羊菜过河问题是一个经典的逻辑谜题,其中有四个角色需要过河,但是只有一条小船,且小船只能容纳两个人。而在四个角色中,狼会吃羊,羊会吃菜,因此需要注意安排过河顺序,以保证所有角色都能安全到达对岸。
以下是一个 Python 实现的人狼羊菜过河问题代码:
```python
# 定义初始状态
start = {'farmer': True, 'wolf': True, 'sheep': True, 'cabbage': True}
# 定义目标状态
goal = {'farmer': False, 'wolf': False, 'sheep': False, 'cabbage': False}
# 定义动作
actions = {
('farmer',): ['farmer_cross'],
('farmer', 'wolf'): ['farmer_cross_with_wolf'],
('farmer', 'sheep'): ['farmer_cross_with_sheep'],
('farmer', 'cabbage'): ['farmer_cross_with_cabbage']
}
# 定义状态转移函数
def transition(state, action):
new_state = state.copy()
if 'wolf' in action:
new_state['wolf'] = not state['wolf']
if 'sheep' in action:
new_state['sheep'] = not state['sheep']
if 'cabbage' in action:
new_state['cabbage'] = not state['cabbage']
new_state['farmer'] = not state['farmer']
return new_state
# 定义判断状态是否合法的函数
def is_valid(state):
if state['wolf'] == state['sheep'] and state['farmer'] != state['wolf']:
return False
if state['sheep'] == state['cabbage'] and state['farmer'] != state['sheep']:
return False
return True
# 定义求解函数
def solve(state, goal, actions, transition, is_valid, path=[]):
if state == goal:
return path
for action, next_actions in actions.items():
if state['farmer'] in action:
for next_action in next_actions:
next_state = transition(state, action)
if is_valid(next_state) and next_state not in path:
new_path = path + [(action, next_action)]
result = solve(next_state, goal, actions, transition, is_valid, new_path)
if result is not None:
return result
return None
# 输出结果
result = solve(start, goal, actions, transition, is_valid)
for action in result:
print(action[0], '->', action[1])
```
这个代码实现了一个求解人狼羊菜过河问题的函数 `solve`,它接受初始状态、目标状态、动作、状态转移函数和判断状态是否合法的函数作为参数,并返回一个路径,表示从初始状态到达目标状态的一系列动作。在实现过程中,我们定义了初始状态、目标状态、动作和状态转移函数,以及判断状态是否合法的函数。其中,动作是一个字典,键表示当前在岸上的角色,值表示当前这些角色可以进行的动作。状态转移函数接受一个状态和一个动作,返回一个新的状态,表示执行这个动作后的状态。判断状态是否合法的函数用于排除一些不符合规则的状态。最后,我们使用深度优先搜索求解从初始状态到达目标状态的路径,并输出结果。
阅读全文
相关推荐
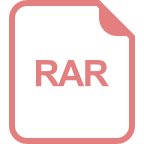



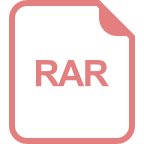
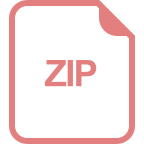
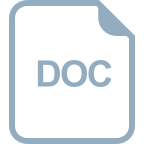









