Java代码实现采用基本队列解决走迷宫问题,其中,迷宫有一个入口和一个出口,其中白色单元表示通路,黑色单元表示不通路。试寻找一条从入口到出口的路径,每一部只能从一个白色单元走到相邻的白色单元,直至出口,并输出路径(不定义方向)
时间: 2023-09-07 18:04:28 浏览: 78
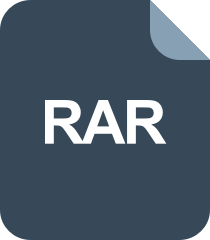
migong.rar_visual c_迷宫问题
### 回答1:
以下是Java代码实现基本队列解决走迷宫问题的示例:
```java
import java.util.LinkedList;
import java.util.Queue;
public class MazeSolver {
private final static int[][] directions = {{0, 1}, {1, 0}, {0, -1}, {-1, 0}}; // 右下左上四个方向
private final static char PATH = '0'; // 表示通路的字符
private final static char WALL = '1'; // 表示不通路的字符
public static void main(String[] args) {
char[][] maze = {
{'0', '1', '1', '1', '1', '1'},
{'0', '0', '0', '1', '1', '1'},
{'1', '0', '1', '0', '0', '1'},
{'1', '0', '1', '1', '0', '1'},
{'1', '0', '0', '0', '0', '0'},
{'1', '1', '1', '1', '1', '0'},
};
int[] start = {0, 0}; // 入口坐标
int[] end = {5, 5}; // 出口坐标
char[][] path = findPath(maze, start, end);
if (path != null) {
for (int i = 0; i < path.length; i++) {
for (int j = 0; j < path[0].length; j++) {
System.out.print(path[i][j] + " ");
}
System.out.println();
}
} else {
System.out.println("No path found!");
}
}
public static char[][] findPath(char[][] maze, int[] start, int[] end) {
int m = maze.length;
int n = maze[0].length;
Queue<int[]> queue = new LinkedList<>();
queue.offer(start);
while (!queue.isEmpty()) {
int[] curr = queue.poll();
if (curr[0] == end[0] && curr[1] == end[1]) {
maze[curr[0]][curr[1]] = PATH; // 标记终点
return maze;
}
for (int[] direction : directions) {
int x = curr[0] + direction[0];
int y = curr[1] + direction[1];
if (x >= 0 && x < m && y >= 0 && y < n && maze[x][y] == PATH) {
maze[x][y] = 'x'; // 标记已经走过的点
queue.offer(new int[]{x, y});
}
}
}
return null;
}
}
```
在上述代码中,我们使用了一个二维字符数组表示迷宫,其中 `0` 表示通路,`1` 表示不通路。起点和终点分别用一个长度为 2 的整型数组表示,用队列来存储待处理的点。在每个点周围搜索四个方向,如果某个方向上的点是通路并且没有被标记过,则将其标记为已经走过的点,并将其加入队列中。如果找到了终点,则返回标记好的路径,否则返回 `null` 表示没有找到路径。
### 回答2:
解决走迷宫问题可以使用基本队列来实现。首先我们假设迷宫由一个二维数组 maze 表示,其中 0 表示白色单元(通路),1 表示黑色单元(不通路)。
我们可以定义一个队列 queue,用来保存待探索的白色单元的坐标。初始化时,将入口的坐标放入队列中。
然后,使用一个二维数组 visited 来记录已经访问过的单元。初始化 visited 为全 0,表示未访问过。如果一个单元已经被访问过,就将 visited 的对应位置设置为 1。
接下来,我们可以使用广度优先搜索的方式来遍历迷宫。每次从队列中取出一个坐标,检查它的上、下、左、右四个相邻的单元是否为通路(即为白色单元)。如果是通路且未访问过,则将其坐标放入队列中,并将 visited 的对应位置设置为已访问。
当队列为空时,表示已经遍历完所有可达的单元。此时,如果出口已经被访问过,说明从入口到出口存在一条路径。我们可以从 visited 数组中逆向寻找路径,即从出口开始,根据上、下、左、右四个相邻的单元是否被访问过来确定路径。最终得到的路径就是从入口到出口的路径。
下面是Java代码实现的示例:
```java
import java.util.LinkedList;
import java.util.Queue;
public class MazeSolver {
public static void solveMaze(int[][] maze, int[] entry, int[] exit) {
int rows = maze.length;
int cols = maze[0].length;
int[][] visited = new int[rows][cols];
Queue<int[]> queue = new LinkedList<>();
queue.offer(entry);
visited[entry[0]][entry[1]] = 1;
int[][] directions = {{-1, 0}, {1, 0}, {0, -1}, {0, 1}};
while (!queue.isEmpty()) {
int[] curr = queue.poll();
int row = curr[0];
int col = curr[1];
if (row == exit[0] && col == exit[1]) {
break;
}
for (int[] dir : directions) {
int newRow = row + dir[0];
int newCol = col + dir[1];
if (newRow >= 0 && newRow < rows && newCol >= 0 && newCol < cols
&& maze[newRow][newCol] == 0 && visited[newRow][newCol] == 0) {
queue.offer(new int[]{newRow, newCol});
visited[newRow][newCol] = 1;
}
}
}
if (visited[exit[0]][exit[1]] == 0) {
System.out.println("无法找到路径");
return;
}
int[][] path = new int[rows][cols];
int row = exit[0];
int col = exit[1];
while (row != entry[0] || col != entry[1]) {
path[row][col] = 1;
for (int[] dir : directions) {
int newRow = row + dir[0];
int newCol = col + dir[1];
if (newRow >= 0 && newRow < rows && newCol >= 0 && newCol < cols
&& visited[newRow][newCol] == 1) {
row = newRow;
col = newCol;
break;
}
}
}
path[entry[0]][entry[1]] = 1;
System.out.println("路径为:");
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
if (path[i][j] == 1) {
System.out.print("* ");
} else {
System.out.print(" ");
}
}
System.out.println();
}
}
}
```
以上代码实现了基本队列解决走迷宫问题的功能,可以找到从入口到出口的路径,并输出路径。
### 回答3:
采用基本队列解决走迷宫问题的Java代码如下:
```java
import java.util.LinkedList;
import java.util.Queue;
public class MazeSolver {
private int[][] maze; // 迷宫二维数组表示,0表示通路,1表示不通路
private int[][] directions = {{0, 1}, {1, 0}, {0, -1}, {-1, 0}}; // 四个方向的移动向量
private int rows, cols; // 迷宫的行数和列数
public MazeSolver(int[][] maze) {
this.maze = maze;
this.rows = maze.length;
this.cols = maze[0].length;
}
public void solveMaze(int startRow, int startCol, int endRow, int endCol) {
int[][] visited = new int[rows][cols]; // 记录每个单元格是否访问过,0表示未访问,1表示已访问
int[][] parent = new int[rows][cols]; // 记录每个单元格的父节点坐标,用于回溯路径
Queue<int[]> queue = new LinkedList<>();
queue.offer(new int[]{startRow, startCol}); // 将起点入队
visited[startRow][startCol] = 1; // 标记起点已访问
boolean foundPath = false;
while (!queue.isEmpty()) {
int[] current = queue.poll(); // 当前所处位置
int currRow = current[0];
int currCol = current[1];
// 判断是否到达终点
if (currRow == endRow && currCol == endCol) {
foundPath = true;
break;
}
// 遍历四个方向
for (int[] direction : directions) {
int nextRow = currRow + direction[0];
int nextCol = currCol + direction[1];
// 判断下一个位置是否在迷宫范围内且为通路且未访问过
if (nextRow >= 0 && nextRow < rows && nextCol >= 0 && nextCol < cols
&& maze[nextRow][nextCol] == 0 && visited[nextRow][nextCol] == 0) {
queue.offer(new int[]{nextRow, nextCol}); // 将下一个位置入队
visited[nextRow][nextCol] = 1; // 标记下一个位置已访问
parent[nextRow][nextCol] = currRow * cols + currCol; // 记录下一个位置的父节点坐标
}
}
}
if (foundPath) {
// 输出路径
int currentRow = endRow;
int currentCol = endCol;
while (!(currentRow == startRow && currentCol == startCol)) {
System.out.println("(" + currentRow + ", " + currentCol + ")");
int parentRow = parent[currentRow][currentCol] / cols;
int parentCol = parent[currentRow][currentCol] % cols;
currentRow = parentRow;
currentCol = parentCol;
}
System.out.println("(" + startRow + ", " + startCol + ")");
} else {
System.out.println("迷宫无解");
}
}
public static void main(String[] args) {
int[][] maze = {
{0, 1, 1, 1, 1},
{0, 0, 0, 0, 1},
{1, 1, 1, 0, 1},
{1, 1, 1, 0, 1},
{1, 1, 1, 0, 0},
{1, 1, 1, 1, 0}
};
int startRow = 0;
int startCol = 0;
int endRow = 5;
int endCol = 4;
MazeSolver solver = new MazeSolver(maze);
solver.solveMaze(startRow, startCol, endRow, endCol);
}
}
```
该代码通过使用基本队列和广度优先搜索(BFS)算法的思想,以Java语言实现了走迷宫问题的求解。主要步骤如下:
1. 定义了一个`MazeSolver`类,内部包含了迷宫数组、移动方向数组、迷宫的行数和列数等成员变量。
2. 构造器中初始化了迷宫的相关数据。
3. `solveMaze`方法接受起始点和终点的行列坐标作为参数,并实现了整个迷宫求解的逻辑。
4. 在求解过程中,使用`Queue`来保存待访问的位置。初始将起始点入队,并标记起始点为已访问。
5. 使用`while`循环,直到队列为空或找到终点为止,不断从队列中取出一个位置,并遍历四个方向的相邻位置。
6. 对每个相邻位置,判断它是否在迷宫范围内且为通路且未访问过,若满足条件,则将其入队,并标记为已访问。
7. 同时,记录每个单元格的父节点坐标,以便后续通过父节点回溯路径。
8. 如果找到终点,则通过父节点的链表回溯路径,并按照要求输出路径。
9. 如果未找到终点,则输出"迷宫无解"。
10. 在`main`方法中,定义迷宫二维数组和起始、终点坐标,并创建`MazeSolver`对象调用`solveMaze`方法进行求解。
阅读全文
相关推荐
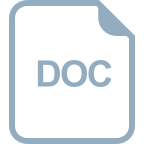
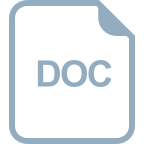





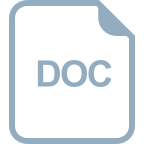









