opencv找出图片中的所有黑色圆点,并在另一张图上单独显示出来,并记录圆形的圆心在图上的坐标
时间: 2024-02-27 20:58:52 浏览: 60
好的,这是一个比较复杂的问题,需要使用到图像处理库 OpenCV。以下是大致的步骤:
1. 读取图片并将其转换为灰度图像。
```python
import cv2
img = cv2.imread("image.jpg")
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
```
2. 使用 Hough 变换检测圆形。
```python
circles = cv2.HoughCircles(gray, cv2.HOUGH_GRADIENT, dp=1, minDist=50, param1=50, param2=30, minRadius=0, maxRadius=0)
```
3. 在另一张图上单独显示出圆形。
```python
output = img.copy()
if circles is not None:
circles = np.round(circles[0, :]).astype("int")
for (x, y, r) in circles:
cv2.circle(output, (x, y), r, (0, 255, 0), 2)
```
4. 记录圆形的圆心在图上的坐标。
```python
if circles is not None:
for (x, y, r) in circles:
print(f"Circle center coordinates: ({x}, {y})")
```
完整的代码如下所示:
```python
import cv2
import numpy as np
# Load image
img = cv2.imread("image.jpg")
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# Detect circles using Hough transform
circles = cv2.HoughCircles(gray, cv2.HOUGH_GRADIENT, dp=1, minDist=50, param1=50, param2=30, minRadius=0, maxRadius=0)
# Draw circles on the output image and print coordinates of circle centers
output = img.copy()
if circles is not None:
circles = np.round(circles[0, :]).astype("int")
for (x, y, r) in circles:
cv2.circle(output, (x, y), r, (0, 255, 0), 2)
print(f"Circle center coordinates: ({x}, {y})")
# Display output image
cv2.imshow("output", output)
cv2.waitKey(0)
```
注意,Hough 变换的参数需要根据具体情况进行调整,以达到最佳的效果。
阅读全文
相关推荐
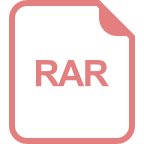
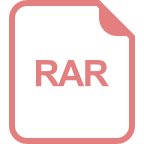
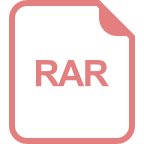
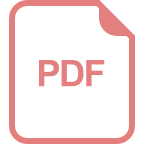
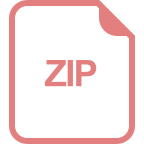
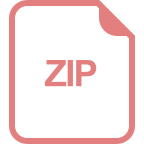
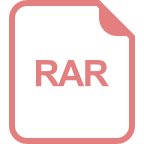
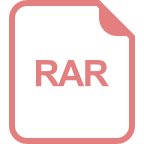
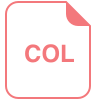



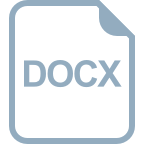