当在vue项目中,用axios发起请求时,需要携带的body参数和header参数分别要写在什么位置
时间: 2024-01-22 22:19:52 浏览: 178
在Vue项目中使用axios发起请求时,我们可以使用以下方法来携带请求参数:
1. 在get请求中,将参数拼接在url后面,例如:
```
axios.get('/api/user?id=123')
```
2. 在post请求中,将参数放在data属性中,例如:
```
axios.post('/api/user', {
id: 123,
name: 'John'
})
```
3. 在发送请求时,可以添加headers属性来设置请求头,例如:
```
axios.post('/api/user', {
id: 123,
name: 'John'
}, {
headers: {
'Content-Type': 'application/json'
}
})
```
其中,headers属性可以设置多个请求头,以对象的形式传递。
相关问题
基于vue和elementui、axios、和springboot写一个评论区的模块,有点赞数+评论
好的,下面是一个简单的实现过程。
前端部分:
1. 使用 Vue CLI 创建一个新项目,然后安装 Element UI、Axios 和 Vue Router。
```
vue create my-project
cd my-project
npm install element-ui axios vue-router --save
```
2. 在 `src` 目录下创建一个 `views` 文件夹,里面放置一个 `Comment.vue` 组件。
```vue
<template>
<div>
<div class="comment-header">
<h3>评论</h3>
<el-button type="primary" @click="showForm = true">添加评论</el-button>
</div>
<div class="comment-body">
<el-card v-for="comment in comments" :key="comment.id">
<div class="comment-info">
<span class="comment-author">{{ comment.author }}</span>
<span class="comment-date">{{ comment.date }}</span>
</div>
<div class="comment-content">{{ comment.content }}</div>
<div class="comment-actions">
<el-button size="small" type="text" @click="editComment(comment)">编辑</el-button>
<el-button size="small" type="text" @click="deleteComment(comment)">删除</el-button>
<el-button size="small" type="text" @click="likeComment(comment)">
{{ comment.likes }} <i class="el-icon-thumb-up"></i>
</el-button>
</div>
</el-card>
</div>
<el-dialog title="添加评论" :visible.sync="showForm" width="50%" center>
<el-form :model="newComment" label-width="80px">
<el-form-item label="用户名">
<el-input v-model="newComment.author"></el-input>
</el-form-item>
<el-form-item label="评论内容">
<el-input type="textarea" v-model="newComment.content"></el-input>
</el-form-item>
</el-form>
<div slot="footer" class="dialog-footer">
<el-button @click="showForm = false">取消</el-button>
<el-button type="primary" @click="addComment">确定</el-button>
</div>
</el-dialog>
</div>
</template>
<script>
import axios from 'axios';
export default {
name: 'Comment',
data() {
return {
comments: [],
showForm: false,
newComment: {
author: '',
content: ''
}
};
},
methods: {
fetchComments() {
axios.get('/api/comments').then(response => {
this.comments = response.data;
}).catch(error => {
console.log(error);
});
},
addComment() {
axios.post('/api/comments', this.newComment).then(response => {
this.comments.push(response.data);
this.newComment.author = '';
this.newComment.content = '';
this.showForm = false;
}).catch(error => {
console.log(error);
});
},
editComment(comment) {
// TODO
},
deleteComment(comment) {
axios.delete(`/api/comments/${comment.id}`).then(response => {
const index = this.comments.indexOf(comment);
this.comments.splice(index, 1);
}).catch(error => {
console.log(error);
});
},
likeComment(comment) {
axios.post(`/api/comments/${comment.id}/like`).then(response => {
comment.likes = response.data.likes;
}).catch(error => {
console.log(error);
});
}
},
mounted() {
this.fetchComments();
}
};
</script>
<style scoped>
.comment-header {
display: flex;
justify-content: space-between;
align-items: center;
margin-bottom: 16px;
}
.comment-info {
display: flex;
justify-content: space-between;
margin-bottom: 8px;
}
.comment-author {
font-weight: bold;
}
.comment-date {
color: #999;
}
.comment-content {
margin-bottom: 8px;
}
.comment-actions {
display: flex;
justify-content: flex-end;
}
</style>
```
3. 在 `src` 目录下创建一个 `router` 文件夹,里面放置一个 `index.js` 路由配置文件。
```js
import Vue from 'vue';
import VueRouter from 'vue-router';
import Comment from '@/views/Comment.vue';
Vue.use(VueRouter);
const routes = [
{
path: '/',
name: 'Comment',
component: Comment
}
];
const router = new VueRouter({
mode: 'history',
base: process.env.BASE_URL,
routes
});
export default router;
```
4. 在 `src` 目录下创建一个 `main.js` 入口文件,里面配置 Axios 和 Element UI。
```js
import Vue from 'vue';
import App from './App.vue';
import router from './router';
import axios from 'axios';
import ElementUI from 'element-ui';
import 'element-ui/lib/theme-chalk/index.css';
Vue.config.productionTip = false;
axios.defaults.baseURL = 'http://localhost:8080';
Vue.prototype.$http = axios;
Vue.use(ElementUI);
new Vue({
router,
render: h => h(App)
}).$mount('#app');
```
5. 创建一个 `public` 文件夹,里面放置一个 `index.html` 文件,用于渲染 Vue 应用。
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>My Project</title>
</head>
<body>
<div id="app"></div>
<script src="/dist/js/chunk-vendors.js"></script>
<script src="/dist/js/app.js"></script>
</body>
</html>
```
后端部分:
1. 使用 Spring Initializr 创建一个新项目,然后添加 Spring Web、Spring Data JPA 和 H2 Database 的依赖。
2. 在 `src/main/java` 目录下创建一个 `com.example.myproject` 包,里面放置一个 `MyProjectApplication.java` 入口文件。
```java
package com.example.myproject;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class MyProjectApplication {
public static void main(String[] args) {
SpringApplication.run(MyProjectApplication.class, args);
}
}
```
3. 在 `src/main/java` 目录下创建一个 `com.example.myproject.controller` 包,里面放置一个 `CommentController.java` 控制器,用于处理评论相关的 API 请求。
```java
package com.example.myproject.controller;
import com.example.myproject.entity.Comment;
import com.example.myproject.repository.CommentRepository;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.*;
import javax.transaction.Transactional;
import java.util.List;
@RestController
@RequestMapping("/api/comments")
public class CommentController {
@Autowired
private CommentRepository commentRepository;
@GetMapping
public List<Comment> getAllComments() {
return commentRepository.findAll();
}
@PostMapping
public Comment createComment(@RequestBody Comment comment) {
return commentRepository.save(comment);
}
@PutMapping("/{id}")
public ResponseEntity<Comment> updateComment(@PathVariable Long id, @RequestBody Comment comment) {
Comment existingComment = commentRepository.findById(id).orElse(null);
if (existingComment == null) {
return ResponseEntity.notFound().build();
}
existingComment.setAuthor(comment.getAuthor());
existingComment.setContent(comment.getContent());
Comment updatedComment = commentRepository.save(existingComment);
return ResponseEntity.ok(updatedComment);
}
@DeleteMapping("/{id}")
public ResponseEntity<Comment> deleteComment(@PathVariable Long id) {
Comment comment = commentRepository.findById(id).orElse(null);
if (comment == null) {
return ResponseEntity.notFound().build();
}
commentRepository.delete(comment);
return ResponseEntity.ok(comment);
}
@Transactional
@PostMapping("/{id}/like")
public ResponseEntity<Comment> likeComment(@PathVariable Long id) {
Comment comment = commentRepository.findById(id).orElse(null);
if (comment == null) {
return ResponseEntity.notFound().build();
}
comment.setLikes(comment.getLikes() + 1);
Comment updatedComment = commentRepository.save(comment);
return ResponseEntity.ok(updatedComment);
}
}
```
4. 在 `src/main/java` 目录下创建一个 `com.example.myproject.entity` 包,里面放置一个 `Comment.java` 实体类,用于表示评论。
```java
package com.example.myproject.entity;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
import java.util.Date;
@Entity
public class Comment {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String author;
private String content;
private Integer likes = 0;
private Date date = new Date();
public Comment() {}
public Comment(String author, String content) {
this.author = author;
this.content = content;
}
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public String getAuthor() {
return author;
}
public void setAuthor(String author) {
this.author = author;
}
public String getContent() {
return content;
}
public void setContent(String content) {
this.content = content;
}
public Integer getLikes() {
return likes;
}
public void setLikes(Integer likes) {
this.likes = likes;
}
public Date getDate() {
return date;
}
public void setDate(Date date) {
this.date = date;
}
}
```
5. 在 `src/main/java` 目录下创建一个 `com.example.myproject.repository` 包,里面放置一个 `CommentRepository.java` 接口,用于与数据库交互。
```java
package com.example.myproject.repository;
import com.example.myproject.entity.Comment;
import org.springframework.data.jpa.repository.JpaRepository;
import org.springframework.stereotype.Repository;
@Repository
public interface CommentRepository extends JpaRepository<Comment, Long> {
}
```
6. 在 `src/main/resources` 目录下创建一个 `application.properties` 配置文件,用于配置 H2 Database。
```
spring.datasource.url=jdbc:h2:mem:testdb
spring.datasource.driverClassName=org.h2.Driver
spring.datasource.username=sa
spring.datasource.password=
spring.jpa.database-platform=org.hibernate.dialect.H2Dialect
spring.jpa.show-sql=true
spring.h2.console.enabled=true
spring.h2.console.path=/h2-console
```
7. 运行项目,访问 `http://localhost:8080/h2-console` 可以进入 H2 Database 控制台,查看评论表的数据。
8. 运行项目,访问 `http://localhost:8080/` 可以进入 Vue 应用,查看评论区的界面效果。
以上就是一个简单的基于 Vue 和 Spring Boot 的评论区模块的实现过程。
用vue组件+vue路由+vuex+axios实现增删改查
好的,下面是一个简单的示例:
首先,我们需要创建一个Vue组件,比如一个用户列表组件,用于展示用户列表和实现增删改查操作:
```html
<template>
<div>
<h2>用户列表</h2>
<table>
<thead>
<tr>
<th>ID</th>
<th>姓名</th>
<th>年龄</th>
<th>性别</th>
<th>操作</th>
</tr>
</thead>
<tbody>
<tr v-for="user in users" :key="user.id">
<td>{{ user.id }}</td>
<td>{{ user.name }}</td>
<td>{{ user.age }}</td>
<td>{{ user.gender }}</td>
<td>
<button @click="editUser(user)">编辑</button>
<button @click="deleteUser(user.id)">删除</button>
</td>
</tr>
</tbody>
</table>
<button @click="addUser">添加用户</button>
<UserForm ref="userForm" @submit="submitUser"></UserForm>
</div>
</template>
<script>
import UserForm from './UserForm.vue'
import { mapGetters, mapActions } from 'vuex'
export default {
components: {
UserForm
},
computed: {
...mapGetters(['users'])
},
methods: {
...mapActions(['fetchUsers', 'createUser', 'updateUser', 'deleteUser']),
addUser () {
this.$refs.userForm.show()
},
editUser (user) {
this.$refs.userForm.show(user)
},
submitUser (user) {
if (user.id) {
this.updateUser(user)
} else {
this.createUser(user)
}
}
},
mounted () {
this.fetchUsers()
}
}
</script>
```
在上面的代码中,我们定义了一个用户列表组件,其中包含了一个表格用于展示用户列表,以及一个添加用户和编辑用户的按钮。点击添加用户按钮会弹出一个表单组件,用于输入新增用户的信息,点击编辑按钮同样会弹出该表单组件,并将选中用户的信息填充到表单中。在表单中填写完用户信息并点击确定后,会触发`submit`事件,我们根据用户是否存在`id`属性来判断是新增用户还是更新用户,然后调用相应的`createUser`或`updateUser`方法。
接下来,我们需要创建一个用户表单组件,用于输入用户信息:
```html
<template>
<div class="modal" v-show="visible">
<div class="modal-mask"></div>
<div class="modal-wrapper">
<div class="modal-container">
<div class="modal-header">
<slot name="header">
{{ formTitle }}
</slot>
</div>
<div class="modal-body">
<form>
<div>
<label for="name">姓名</label>
<input id="name" type="text" v-model="user.name" />
</div>
<div>
<label for="age">年龄</label>
<input id="age" type="text" v-model="user.age" />
</div>
<div>
<label for="gender">性别</label>
<select id="gender" v-model="user.gender">
<option value="男">男</option>
<option value="女">女</option>
</select>
</div>
</form>
</div>
<div class="modal-footer">
<button class="modal-default-button" @click="cancel">取消</button>
<button class="modal-default-button" @click="submitForm">确定</button>
</div>
</div>
</div>
</div>
</template>
<script>
export default {
props: {
user: {
type: Object,
default: () => ({})
}
},
data () {
return {
formTitle: this.user.id ? '编辑用户' : '添加用户'
}
},
computed: {
visible: {
get () {
return this.user.id !== undefined
},
set (value) {
this.$emit('update:visible', value)
}
}
},
methods: {
show (user = {}) {
this.$emit('update:user', user)
this.visible = true
},
cancel () {
this.visible = false
},
submitForm () {
this.$emit('submit', this.user)
this.visible = false
}
}
}
</script>
```
在上面的代码中,我们定义了一个用户表单组件,其中包含了姓名、年龄和性别三个输入框,以及确定和取消按钮。在表单中填写完用户信息并点击确定按钮后,会触发`submit`事件,并将用户信息作为参数传递给父组件。
接下来,我们需要创建一个Vuex模块,用于管理用户列表和实现增删改查操作:
```javascript
import axios from 'axios'
const state = {
users: []
}
const mutations = {
setUsers (state, users) {
state.users = users
},
addUser (state, user) {
state.users.push(user)
},
updateUser (state, user) {
const index = state.users.findIndex(u => u.id === user.id)
if (index !== -1) {
state.users.splice(index, 1, user)
}
},
deleteUser (state, userId) {
const index = state.users.findIndex(u => u.id === userId)
if (index !== -1) {
state.users.splice(index, 1)
}
}
}
const actions = {
async fetchUsers ({ commit }) {
const response = await axios.get('/api/users')
commit('setUsers', response.data)
},
async createUser ({ commit }, user) {
const response = await axios.post('/api/users', user)
commit('addUser', response.data)
},
async updateUser ({ commit }, user) {
await axios.put(`/api/users/${user.id}`, user)
commit('updateUser', user)
},
async deleteUser ({ commit }, userId) {
await axios.delete(`/api/users/${userId}`)
commit('deleteUser', userId)
}
}
const getters = {
users: state => state.users
}
export default {
state,
mutations,
actions,
getters
}
```
在上面的代码中,我们定义了一个名为`users`的状态,用于存储用户列表。然后,我们定义了四个Mutation方法,分别用于设置用户列表、添加用户、更新用户和删除用户。接着,我们定义了四个Action方法,分别用于获取用户列表、创建用户、更新用户和删除用户。最后,我们定义了一个Getter方法,用于获取用户列表。
最后,我们需要配置Vue Router和创建一个入口文件,用于启动我们的应用:
```javascript
import Vue from 'vue'
import VueRouter from 'vue-router'
import Vuex from 'vuex'
import App from './App.vue'
import UserList from './components/UserList.vue'
import UserStore from './store/user'
Vue.use(VueRouter)
Vue.use(Vuex)
const router = new VueRouter({
mode: 'history',
routes: [
{ path: '/', component: UserList }
]
})
const store = new Vuex.Store({
modules: {
user: UserStore
}
})
new Vue({
el: '#app',
router,
store,
render: h => h(App)
})
```
在上面的代码中,我们首先引入了Vue、Vue Router和Vuex,并定义了一个Vue实例,其包含了一个`UserList`组件和一个名为`user`的Vuex模块。然后,我们创建了一个Vue Router实例,并将其传递给Vue实例。最后,我们启动了应用,并将Vue实例挂载到`#app`元素上。
到这里,我们就完成了用Vue组件、Vue Router、Vuex和Axios实现增删改查的示例。当然,这只是一个简单的示例,实际应用中可能需要更多的功能和处理更多的异常情况。
阅读全文
相关推荐
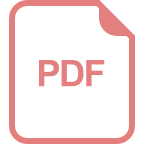
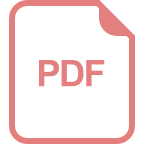
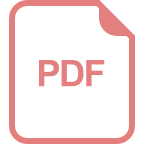
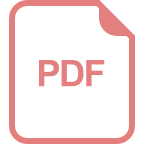
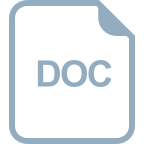











