用python写一个单源最短路径算法代码(包括关键行注释)
时间: 2024-11-15 11:25:19 浏览: 38
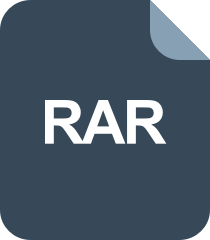
zuiduanlujing.rar_单源最短路径
当然可以。在Python中,我们可以使用Dijkstra's algorithm (迪杰斯特拉算法) 来解决单源最短路径问题。这个算法通常用于图论中,找到从一个节点到所有其他节点的最短路径。这里是一个基本的实现,我将为你解释关键部分:
```python
import heapq
def dijkstra(graph, start):
# 初始化:距离字典记录每个节点到起始点的距离,初始值为无穷大(除了起点)
distances = {node: float('infinity') for node in graph}
distances[start] = 0
# 优先队列,堆顶是最小距离的节点
priority_queue = [(0, start)]
while priority_queue:
# 提取当前距离最小的节点
current_distance, current_node = heapq.heappop(priority_queue)
# 如果已经找到了更短的路径,更新其邻居节点的距离
if current_distance > distances[current_node]:
continue
# 遍历当前节点的所有邻居
for neighbor, weight in graph[current_node].items():
distance = current_distance + weight
# 更新邻接节点的最短距离,如果新路径比之前的更短
if distance < distances[neighbor]:
distances[neighbor] = distance
# 把邻居节点加入优先队列,带入新的估计距离
heapq.heappush(priority_queue, (distance, neighbor))
return distances
# 使用示例:
graph = {
'A': {'B': 1, 'C': 4},
'B': {'A': 1, 'C': 2, 'D': 5},
'C': {'A': 4, 'B': 2, 'D': 1},
'D': {'B': 5, 'C': 1}
}
shortest_paths = dijkstra(graph, 'A')
print("Shortest paths from 'A':", shortest_paths)
```
关键部分是`heapq.heappop`和`heapq.heappush`函数,它们分别用于从堆中弹出最小元素和插入元素保持堆的性质。另外,通过不断迭代直到优先队列为空,算法保证了每次处理的节点都是未发现更短路径的最近节点。
阅读全文
相关推荐
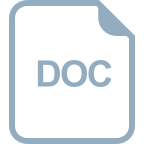
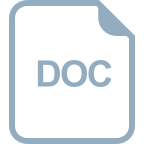

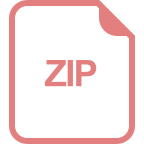
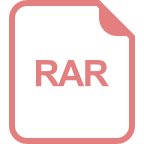
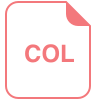
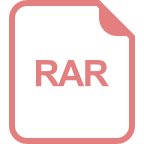
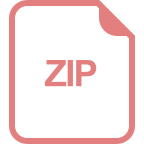
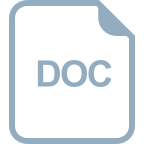


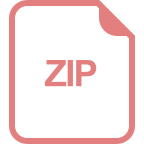
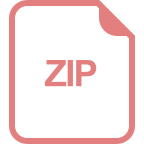
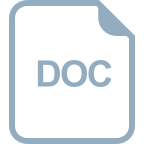
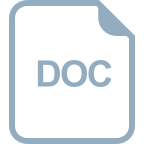
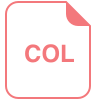
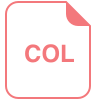
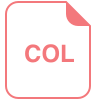