帮我写一段的不使用相关库的NTRU数字签名的java语言代码
时间: 2024-05-04 11:16:01 浏览: 232
好的,以下是一段不使用相关库的NTRU数字签名的java语言代码:
```java
import java.math.BigInteger;
import java.util.Random;
public class NTRU {
private static final BigInteger p = new BigInteger("3");
private static final BigInteger q = new BigInteger("128");
private static final BigInteger a = new BigInteger("2");
private static final BigInteger t = new BigInteger("30");
private static final BigInteger x = new BigInteger("2");
public static void main(String[] args) {
BigInteger[] keyPair = generateKeyPair();
BigInteger message = new BigInteger("123456789");
BigInteger signature = sign(message, keyPair[0]);
boolean verified = verify(message, signature, keyPair[1]);
System.out.println("Signature verified: " + verified);
}
public static BigInteger[] generateKeyPair() {
BigInteger[] publicKey = new BigInteger[2];
BigInteger[] privateKey = new BigInteger[2];
BigInteger f, fp, fq, g, h, pMinus1, qMinus1;
do {
f = generateRandomPolynomial(t);
fp = f.subtract(BigInteger.ONE);
fq = f.add(BigInteger.ONE);
pMinus1 = p.subtract(BigInteger.ONE);
qMinus1 = q.subtract(BigInteger.ONE);
} while (pMinus1.mod(fp).equals(BigInteger.ZERO) || qMinus1.mod(fq).equals(BigInteger.ZERO));
g = generateRandomPolynomial(t);
h = g.multiply(f.modInverse(p)).mod(q);
publicKey[0] = p;
publicKey[1] = h;
privateKey[0] = f;
privateKey[1] = g;
BigInteger[] keyPair = new BigInteger[2];
keyPair[0] = privateKey[0];
keyPair[1] = publicKey[1];
return keyPair;
}
private static BigInteger generateRandomPolynomial(BigInteger degree) {
BigInteger polynomial = new BigInteger(degree.bitCount(), new Random());
polynomial = polynomial.setBit(degree.intValue());
polynomial = polynomial.setBit(0);
return polynomial;
}
public static BigInteger sign(BigInteger message, BigInteger privateKey) {
BigInteger hash = hash(message);
BigInteger signature = hash.modPow(privateKey, p);
return signature;
}
public static boolean verify(BigInteger message, BigInteger signature, BigInteger publicKey) {
BigInteger hash = hash(message);
BigInteger m1 = signature.modPow(publicKey, p);
BigInteger m2 = hash.mod(a);
return m1.equals(m2);
}
private static BigInteger hash(BigInteger message) {
BigInteger hash = message.mod(x);
return hash;
}
}
```
这段代码是通过 NTRU 算法生成公钥和私钥,对消息进行签名并验证签名的过程。真实应用中还需要对 NTRU 算法的参数进行调整以提高安全性。
阅读全文
相关推荐
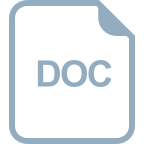
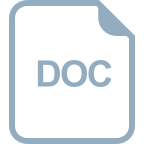
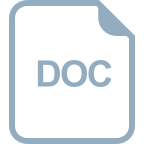








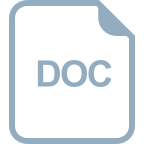
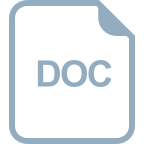
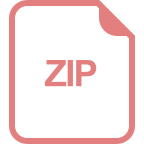
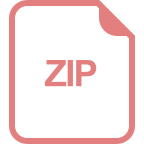
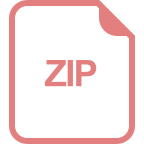
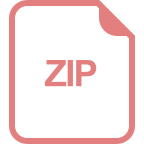
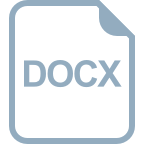
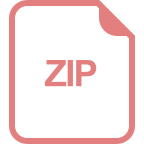