stm32循迹小车代码
时间: 2023-09-02 11:15:37 浏览: 89
循迹小车的代码可以分为以下几个部分:
1.引入头文件和定义全局变量
```
#include "stm32f10x.h"
#include "delay.h"
#include "usart.h"
#define IN1 GPIO_Pin_0
#define IN2 GPIO_Pin_1
#define IN3 GPIO_Pin_2
#define IN4 GPIO_Pin_3
#define ENA TIM1->CCR1
#define ENB TIM1->CCR2
uint16_t LeftSpeed = 0;
uint16_t RightSpeed = 0;
```
2.初始化函数
```
void Init()
{
delay_init();
USART1_Init(9600);
TIM1_PWM_Init(5000-1, 71); //PWM频率为5KHz
TIM_SetCompare1(TIM1, 0);
TIM_SetCompare2(TIM1, 0);
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA | RCC_APB2Periph_GPIOB, ENABLE);
GPIO_InitStructure.GPIO_Pin = IN1 | IN2 | IN3 | IN4;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
}
```
3.控制左右轮速度的函数
```
void SetLeftSpeed(int16_t speed)
{
if(speed > 0)
{
GPIO_WriteBit(GPIOA, IN1, Bit_SET);
GPIO_WriteBit(GPIOA, IN2, Bit_RESET);
ENA = speed;
}
else if(speed < 0)
{
GPIO_WriteBit(GPIOA, IN1, Bit_RESET);
GPIO_WriteBit(GPIOA, IN2, Bit_SET);
ENA = -speed;
}
else
{
GPIO_WriteBit(GPIOA, IN1, Bit_RESET);
GPIO_WriteBit(GPIOA, IN2, Bit_RESET);
ENA = 0;
}
}
void SetRightSpeed(int16_t speed)
{
if(speed > 0)
{
GPIO_WriteBit(GPIOA, IN3, Bit_RESET);
GPIO_WriteBit(GPIOA, IN4, Bit_SET);
ENB = speed;
}
else if(speed < 0)
{
GPIO_WriteBit(GPIOA, IN3, Bit_SET);
GPIO_WriteBit(GPIOA, IN4, Bit_RESET);
ENB = -speed;
}
else
{
GPIO_WriteBit(GPIOA, IN3, Bit_RESET);
GPIO_WriteBit(GPIOA, IN4, Bit_RESET);
ENB = 0;
}
}
```
4.循迹函数
```
void RunTrack()
{
uint16_t Left, Mid, Right;
Left = GPIO_ReadInputDataBit(GPIOB, GPIO_Pin_11);
Mid = GPIO_ReadInputDataBit(GPIOB, GPIO_Pin_10);
Right = GPIO_ReadInputDataBit(GPIOB, GPIO_Pin_1);
if(Left == 1 && Mid == 0 && Right == 1)
{
SetLeftSpeed(1000);
SetRightSpeed(1000);
}
else if(Left == 1 && Mid == 0 && Right == 0)
{
SetLeftSpeed(1000);
SetRightSpeed(800);
}
else if(Left == 0 && Mid == 0 && Right == 1)
{
SetLeftSpeed(800);
SetRightSpeed(1000);
}
else if(Left == 0 && Mid == 1 && Right == 0)
{
SetLeftSpeed(1000);
SetRightSpeed(1000);
}
else if(Left == 1 && Mid == 1 && Right == 0)
{
SetLeftSpeed(1000);
SetRightSpeed(800);
}
else if(Left == 0 && Mid == 1 && Right == 1)
{
SetLeftSpeed(800);
SetRightSpeed(1000);
}
else
{
SetLeftSpeed(0);
SetRightSpeed(0);
}
}
```
5.主函数
```
int main(void)
{
Init();
while (1)
{
RunTrack();
delay_ms(10); //控制循迹小车的循迹速度
}
}
```
以上就是循迹小车的代码,需要注意的是,具体的接线方式和传感器的编号可能会因为不同的循迹小车而有所不同,需要具体的参考相关的文档。
阅读全文
相关推荐
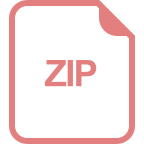
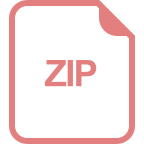
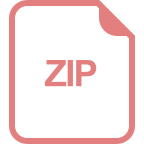
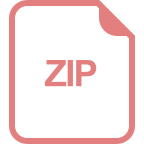
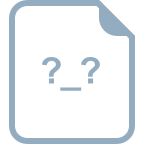
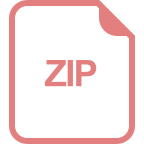
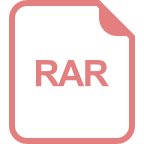
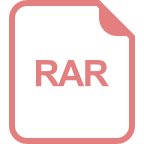
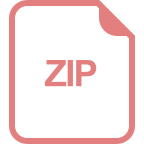
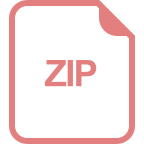







