请详细给出 Towers(4,a,b,c)的函数调用过程和move( )过程.
时间: 2024-05-29 12:12:47 浏览: 7
Towers(4,a,b,c)的函数调用过程如下:
首先,我们将4个圆盘分成两个部分:最下面的一个圆盘,和其余的三个圆盘。
1. 将最下面的圆盘从柱子a移动到柱子c,通过调用move(a,c)函数。
2. 将剩下的三个圆盘从柱子a移动到柱子b,通过调用Towers(3,a,c,b)函数。
3. 将最下面的圆盘从柱子c移动到柱子b,通过调用move(c,b)函数。
4. 将剩下的三个圆盘从柱子c移动到柱子a,通过调用Towers(3,b,a,c)函数。
5. 将最下面的圆盘从柱子b移动到柱子a,通过调用move(b,a)函数。
6. 最后,将剩下的三个圆盘从柱子b移动到柱子c,通过调用Towers(3,a,b,c)函数。
而move()函数的过程则是:
1. 将最上层的盘子从起始柱子移动到目标柱子。
2. 输出一条消息,说明移动了哪个盘子和它从哪个柱子移动到了哪个柱子。
所以,Towers(4,a,b,c)的函数调用过程和move()函数的过程,都是为了解决汉诺塔问题的。
相关问题
汉诺塔问题的示例图汉诺塔是一个数学难题,其问题描述为如何将所有圆盘从A移动到C。请用Python编写一个汉诺塔的移动函数,采用递归方法解决这个难题,要求输入汉诺塔的层数,输出整个移动流程;并再设计一个绘图函数,用turtle实现上图效果,表述汉诺塔移动流程。
好的,这是一个Python的代码实现,包括汉诺塔移动函数和绘图函数:
```python
import turtle
# 汉诺塔移动函数
def hanoi(n, a, b, c):
if n == 1:
print(a, '->', c)
else:
hanoi(n-1, a, c, b)
print(a, '->', c)
hanoi(n-1, b, a, c)
# 绘图函数
def draw_hanoi(n, a, b, c, width, height):
def draw_tower(x, y, w, h):
t.penup()
t.goto(x, y)
t.pendown()
t.setheading(0)
t.forward(w/2)
t.right(90)
t.forward(h)
t.right(90)
t.forward(w)
t.right(90)
t.forward(h)
t.right(90)
t.forward(w/2)
def draw_disk(x, y, w, h):
t.penup()
t.goto(x, y)
t.pendown()
t.setheading(0)
t.forward(w/2)
t.right(90)
t.forward(h)
t.right(90)
t.forward(w)
t.right(90)
t.forward(h)
t.right(90)
t.forward(w/2)
def move_disk(src, dst):
disk = towers[src].pop()
x, y = positions[src][len(towers[src])]
t.penup()
t.goto(x, y)
t.pendown()
x, y = positions[dst][len(towers[dst])]
t.goto(x, y)
towers[dst].append(disk)
t = turtle.Turtle()
t.speed('fastest')
t.hideturtle()
t.penup()
tower_width = width // 3
tower_height = height // (n+2)
positions = {
a: [(tower_width*0.5, -tower_height*i) for i in range(n)],
b: [(tower_width*1.5, -tower_height*i) for i in range(n)],
c: [(tower_width*2.5, -tower_height*i) for i in range(n)],
}
draw_tower(tower_width*0.5, 0, tower_width, height)
draw_tower(tower_width*1.5, 0, tower_width, height)
draw_tower(tower_width*2.5, 0, tower_width, height)
towers = {
a: list(range(n, 0, -1)),
b: [],
c: [],
}
disks = [turtle.Turtle() for _ in range(n)]
for i in range(n):
w = tower_width * (n-i) / n
h = tower_height
draw_disk(*positions[a][i], w, h)
def move(n, src, dst, tmp):
if n == 1:
move_disk(src, dst)
else:
move(n-1, src, tmp, dst)
move_disk(src, dst)
move(n-1, tmp, dst, src)
move(n, a, c, b)
turtle.done()
# 测试
n = 3
hanoi(n, 'A', 'B', 'C')
draw_hanoi(n, 'A', 'B', 'C', 600, 400)
```
这个程序实现了汉诺塔移动函数 `hanoi()` 和绘图函数 `draw_hanoi()`。其中,`hanoi()` 函数使用递归实现汉诺塔的移动,将圆盘从 A 移动到 C。`draw_hanoi()` 函数使用 `turtle` 模块绘制汉诺塔的图形,并模拟移动过程。在 `draw_hanoi()` 函数中,使用 `positions` 变量记录每个圆盘的位置,使用 `towers` 变量记录每个塔的状态,使用 `disks` 变量记录每个圆盘的图形。在 `move()` 函数中,使用递归模拟移动过程,并在每次移动时更新 `towers` 和 `disks` 变量。最后调用 `turtle.done()` 函数显示图形。
用户可自己移动汉诺塔上盘子的移动,并且当完成汉诺塔时,会给出恭喜字样
以下是一个带用户交互的 C++ 汉诺塔小游戏的代码:
```c++
#include <iostream>
#include <stack>
using namespace std;
void move(stack<int>& source, stack<int>& target, char s, char t) {
int disk = source.top();
source.pop();
target.push(disk);
cout << "Move disk " << disk << " from " << s << " to " << t << endl;
}
void hanoi(stack<int>& source, stack<int>& target, stack<int>& auxiliary, int n) {
if (n == 1) {
move(source, target, 'A', 'C');
return;
}
hanoi(source, auxiliary, target, n-1);
move(source, target, 'A', 'C');
hanoi(auxiliary, target, source, n-1);
}
int main() {
int n;
cout << "Enter the number of disks: ";
cin >> n;
stack<int> A, B, C;
for (int i = n; i >= 1; i--) {
A.push(i);
}
while (true) {
cout << "Current state of the towers:" << endl;
cout << "A: ";
stack<int> tempA = A;
while (!tempA.empty()) {
cout << tempA.top() << " ";
tempA.pop();
}
cout << endl;
cout << "B: ";
stack<int> tempB = B;
while (!tempB.empty()) {
cout << tempB.top() << " ";
tempB.pop();
}
cout << endl;
cout << "C: ";
stack<int> tempC = C;
while (!tempC.empty()) {
cout << tempC.top() << " ";
tempC.pop();
}
cout << endl;
if (C.size() == n) {
cout << "Congratulations, you have completed the game!" << endl;
break;
}
char s, t;
cout << "Enter the tower to move from (A/B/C): ";
cin >> s;
cout << "Enter the tower to move to (A/B/C): ";
cin >> t;
stack<int>* source, *target;
if (s == 'A') {
source = &A;
} else if (s == 'B') {
source = &B;
} else {
source = &C;
}
if (t == 'A') {
target = &A;
} else if (t == 'B') {
target = &B;
} else {
target = &C;
}
if (source->empty() || (!target->empty() && source->top() > target->top())) {
cout << "Invalid move, please try again." << endl;
continue;
}
move(*source, *target, s, t);
}
return 0;
}
```
运行该程序后,会要求用户输入盘子的数量,然后输出当前汉诺塔的状态。用户可以输入要移动的盘子的来源和目的地,程序会判断是否合法并移动盘子,直到用户完成汉诺塔。
相关推荐
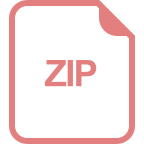
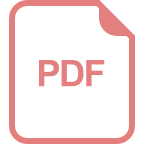












