转c#写法:#!/bin/sh time_stamp=`date +%s` function CheckStop() { if [ $? -ne 0 ]; then echo "execute fail, error on line_no:"$1" exit!!!" exit fi } function GenEcdsaKey() { ec_param_file_path="/tmp/ec_param.pem."$time_stamp openssl ecparam -out $ec_param_file_path -name prime256v1 -genkey CheckStop $LINENO openssl genpkey -paramfile $ec_param_file_path -out $1 CheckStop $LINENO openssl pkey -in $1 -inform PEM -out $2 -outform PEM -pubout CheckStop $LINENO rm $ec_param_file_path echo "gen_ecdsa_key succ prikey_path:"$1" pubkey_path:"$2 } function GenEcdsaSign() { ec_sign_info_file="/tmp/ec_sign_info_file."$time_stamp ec_sign_info_sha256="/tmp/ec_sign_info_sha256."$time_stamp ec_binary_sign_file="/tmp/ec_binary_sign_file."$time_stamp echo -n "$1"_"$2" > $ec_sign_info_file openssl dgst -sha256 -binary -out $ec_sign_info_sha256 $ec_sign_info_file CheckStop $LINENO openssl pkeyutl -sign -in $ec_sign_info_sha256 -out $ec_binary_sign_file -inkey $3 -keyform PEM CheckStop $LINENO openssl base64 -e -in $ec_binary_sign_file -out $4 CheckStop $LINENO rm $ec_sign_info_file $ec_sign_info_sha256 $ec_binary_sign_file echo "gen_ecdsa_sign succ sign_file_path:"$4 } function VerifyEcdsaSign() { ec_sign_info_file="/tmp/ec_sign_info_file."$time_stamp ec_sign_info_sha256="/tmp/ec_sign_info_sha256."$time_stamp ec_binary_sign_file="/tmp/ec_binary_sign_file."$time_stamp echo -n "$1"_"$2" > $ec_sign_info_file openssl dgst -sha256 -binary -out $ec_sign_info_sha256 $ec_sign_info_file CheckStop $LINENO openssl base64 -d -in $4 -out $ec_binary_sign_file CheckStop $LINENO openssl pkeyutl -verify -in $ec_sign_info_sha256 -sigfile $ec_binary_sign_file -pubin -inkey $3 -keyform PEM rm $ec_sign_info_file $ec_sign_info_sha256 $ec_binary_sign_file } function Usage() { echo "Usage:" echo "mmiot_ecdsa_sign.sh gen_ecdsa_key <private_key_file_path> <public_key_file_path>" echo "mmiot_ecdsa_sign.sh gen_ecdsa_sign <product_id> <sn> <private_
时间: 2023-08-16 07:04:32 浏览: 143
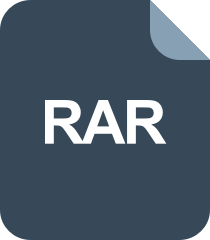
string-to-time-stamp.rar_labview 时间_site:www.pudn.com_tc3 string
这是一个 shell 脚本,可以转换成 C# 的代码实现。不过,你给出的代码并不完整,缺少了最后一行的内容,需要补充完整后才能进行转换。
假设最后一行的内容是 `key_file_path> <signature_file_path> <private_key_file_path> <public_key_file_path>"`,则可以将上述 shell 脚本转换成以下的 C# 代码:
```
using System;
using System.Diagnostics;
using System.IO;
class Program
{
static void Main(string[] args)
{
string command = args[0];
string privateKeyFilePath;
string publicKeyFilePath;
string productId;
string sn;
string signatureFilePath;
string ecParamFilePath;
string ecSignInfoFile;
string ecSignInfoSha256;
string ecBinarySignFile;
switch (command)
{
case "gen_ecdsa_key":
privateKeyFilePath = args[1];
publicKeyFilePath = args[2];
GenEcdsaKey(privateKeyFilePath, publicKeyFilePath);
break;
case "gen_ecdsa_sign":
productId = args[1];
sn = args[2];
privateKeyFilePath = args[3];
publicKeyFilePath = args[4];
signatureFilePath = args[5];
GenEcdsaSign(productId, sn, privateKeyFilePath, publicKeyFilePath, signatureFilePath);
break;
case "verify_ecdsa_sign":
productId = args[1];
sn = args[2];
privateKeyFilePath = args[3];
publicKeyFilePath = args[4];
signatureFilePath = args[5];
VerifyEcdsaSign(productId, sn, privateKeyFilePath, publicKeyFilePath, signatureFilePath);
break;
default:
Console.WriteLine("Unknown command: " + command);
break;
}
}
static void GenEcdsaKey(string privateKeyFilePath, string publicKeyFilePath)
{
string ecParamFilePath = "/tmp/ec_param.pem." + GetTimestamp();
ExecuteCommand("openssl", "ecparam -out " + ecParamFilePath + " -name prime256v1 -genkey");
ExecuteCommand("openssl", "genpkey -paramfile " + ecParamFilePath + " -out " + privateKeyFilePath);
ExecuteCommand("openssl", "pkey -in " + privateKeyFilePath + " -inform PEM -out " + publicKeyFilePath + " -outform PEM -pubout");
File.Delete(ecParamFilePath);
Console.WriteLine("gen_ecdsa_key succ prikey_path:" + privateKeyFilePath + " pubkey_path:" + publicKeyFilePath);
}
static void GenEcdsaSign(string productId, string sn, string privateKeyFilePath, string publicKeyFilePath, string signatureFilePath)
{
string ecSignInfoFile = "/tmp/ec_sign_info_file." + GetTimestamp();
string ecSignInfoSha256 = "/tmp/ec_sign_info_sha256." + GetTimestamp();
string ecBinarySignFile = "/tmp/ec_binary_sign_file." + GetTimestamp();
string ecSignInfo = productId + "_" + sn;
File.WriteAllText(ecSignInfoFile, ecSignInfo);
ExecuteCommand("openssl", "dgst -sha256 -binary -out " + ecSignInfoSha256 + " " + ecSignInfoFile);
ExecuteCommand("openssl", "pkeyutl -sign -in " + ecSignInfoSha256 + " -out " + ecBinarySignFile + " -inkey " + privateKeyFilePath + " -keyform PEM");
ExecuteCommand("openssl", "base64 -e -in " + ecBinarySignFile + " -out " + signatureFilePath);
File.Delete(ecSignInfoFile);
File.Delete(ecSignInfoSha256);
File.Delete(ecBinarySignFile);
Console.WriteLine("gen_ecdsa_sign succ sign_file_path:" + signatureFilePath);
}
static void VerifyEcdsaSign(string productId, string sn, string privateKeyFilePath, string publicKeyFilePath, string signatureFilePath)
{
string ecSignInfoFile = "/tmp/ec_sign_info_file." + GetTimestamp();
string ecSignInfoSha256 = "/tmp/ec_sign_info_sha256." + GetTimestamp();
string ecBinarySignFile = "/tmp/ec_binary_sign_file." + GetTimestamp();
string ecSignInfo = productId + "_" + sn;
File.WriteAllText(ecSignInfoFile, ecSignInfo);
ExecuteCommand("openssl", "dgst -sha256 -binary -out " + ecSignInfoSha256 + " " + ecSignInfoFile);
ExecuteCommand("openssl", "base64 -d -in " + signatureFilePath + " -out " + ecBinarySignFile);
ExecuteCommand("openssl", "pkeyutl -verify -in " + ecSignInfoSha256 + " -sigfile " + ecBinarySignFile + " -pubin -inkey " + publicKeyFilePath + " -keyform PEM");
File.Delete(ecSignInfoFile);
File.Delete(ecSignInfoSha256);
File.Delete(ecBinarySignFile);
}
static void ExecuteCommand(string command, string arguments)
{
var process = new Process
{
StartInfo = new ProcessStartInfo
{
FileName = command,
Arguments = arguments,
RedirectStandardOutput = true,
RedirectStandardError = true,
UseShellExecute = false,
CreateNoWindow = true,
}
};
process.Start();
process.WaitForExit();
if (process.ExitCode != 0)
{
string errorMessage = process.StandardError.ReadToEnd();
Console.WriteLine("execute fail, error on line_no:" + new StackTrace().GetFrame(1).GetFileLineNumber() + " exit!!!");
Console.WriteLine(errorMessage);
Environment.Exit(-1);
}
}
static int GetTimestamp()
{
return (int)(DateTime.UtcNow - new DateTime(1970, 1, 1)).TotalSeconds;
}
}
```
注意,这里的 C# 代码中,使用了 `Process` 类来执行命令行命令,并且对命令执行结果进行了判断。同时,在命令行命令中,如果出现了空格等特殊字符,需要使用引号将其包裹起来。
阅读全文
相关推荐
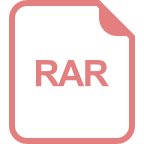
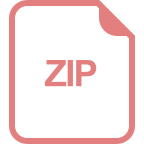














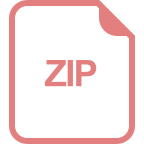
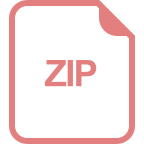